Overview
ARC provides the JavaScript programming language for custom code that interconnects skills. The ARC JavaScript API provides access to standard ECMA 5.1 Script specifications and a library of additional functions. The other functions are defined in this section.
JavaScript is not JAVA. JavaScript, often abbreviated as JS, is a programming language that conforms to the ECMA 5.1 Script specification. JavaScript is high-level, just-in-time compiled, and multi-paradigm. It has curly-bracket syntax, dynamic typing, prototype-based object orientation, and first-class functions. JavaScript engines were initially used only in web browsers, but they are now core components of other software systems, such as Synthiam ARC. Although there are similarities between JavaScript and Java, including language name, syntax, and respective standard libraries, the two languages are distinct and differ significantly in design.
The ARC JavaScript engine exposes several objects with methods for interacting with hardware, the framework, and other robot skills. Information on creating global user-defined custom objects and methods is also provided at the end of this document.
ARC JavaScript Functions
In addition to the built-in ECMA 5.1 JavaScript classes (outlined below), ARC includes several types with methods to control your robot, read sensors, interact with the operating system, and more. To view built-in ARC JavaScript methods…
1)
Check the intellisense checkbox.
2)
Type one of the following class names, press ‘.’, and scroll through the available methods.
ADC
– EZB analog to digital converter functions
Audio
– streaming audio functions
COM
– local serial COM port functions
Digital
– EZB digital I/O functions
EZB
– EZB-specific functions for getting voltage, checking the connection, etc.
File
– writing and reading local files
I2C
– EZB I2C helper functions
Movement
– movement panel control of directions
Net
– networking functions
Ping
– EZB ultrasonic distance sensor functions
PWM
– EZB pulse width modulation on digital I/O pins
Servo
– EZB servo movement functions
UART
– EZB hardware UART and software Serial functions
Utility
– various utility functions
Supported JavaScript ECMA 5.1 Classes
The JavaScript ECMA 5.1 includes several classes for working with strings, math, and various features. For detailed information and examples, the following classes can be researched online at developer.mozilla.org and w3schools.com.
- Array
- Boolean
- Console (support for time, count, error, info, log)
- Date
- Error
- Iterator
- JSON
- Map
- Math
- Number
- Object
- Proxy
- Reflect
- RegExp
- Set
- String
- Symbol
Root Commands
Root commands do not belong to a class and are included for convenience to access global variables and send commands to other robot skills.
getVar( variableName, [default value] )
Retrieves the value from the Arc’s public global variable storage. These are variables published by robot skills, such as the Camera, Auto Position, Speech Recognition, etc... (Read More)
{variableName} - The name of the global variable as a string
{default value} – [Optional] If specified, this value is returned if the global variable doesn’t exist.
{return} – The value of the global variable
Example:
// Get the current direction the robot is moving
var direction = getVar(“$Direction”);
// Get the value of $test, and if it doesn’t exist, return false
var testVar = getVar(“$test”, false);
setVar( variableName, value )
Sets the value from Arc’s public global variable storage. This allows the variable to be available to other robot skills or scripts using getVar(). (Read More)
{variableName} - The name of the global variable as a string
{value} – The value that you wish to store in the global variable
Example:
// Set a value of 5 to be accessible by other robot skills
setVar(“$MyValue”, 5);
setVarObject( variableName, value )
Sets the value from Arc’s public global variable storage of the object. This allows the variable to be available to other robot skills or scripts using getVar(). (Read More). This differs from setVar() because it sets the value as an object without any translation. So you can pass entire objects, such as functions, classes, or multidimensional arrays.
{variableName} - The name of the global variable as a string
{value} – The object value that you wish to store in the global variable
Example:
// Set the multidimensional array to be accessible by other robot skills with getVar()
x = [ [0, 1, 2], [3, 4, 5] ];
setVar(“$MyValue”, x);
varExists( variableName )
Check if the global variable exists. (Read More)
{variableName} - The name of the global variable as a string
{return} – true/false Boolean if the variable exists
Example:
// print if the variable exists
print(varExists(“$MyValue”));
print( txt )
Writes the value to the console output.
{txt} – The value/data to print
Example:
// Print a string to the console output
print(“Hello World”);
sleep( timeMs )
Pauses the execution of the program for the specified time in milliseconds
{timeMS} – The time to pause
Example:
// Print a string, pause for 5 seconds, and print another string
print(“Hello”);
sleep(5000);
print(“ World”);
sleepRandom( minTimeMS, maxTimeMS )
Pauses the program's execution for random milliseconds between the min and max.
{minTimeMS} – The minimum time to pause
{maxTimeMS} – The maximum time to pause
Example:
// Print a string, pause for a random time, and print another string
print(“Hello”);
sleepRandom(1000, 5000);
print(“ World”);
controlCommand( control name, command, [parameters] )
Sends the command to the control name with the optional parameters. The cheat sheet displays all of the interrogated control commands supported by roobt skills in the project.
Example:
// Start the camera
controlCommand(“Camera”, “StartCamera”);
print(“Camera has started”);
showControl( controlName )
Shows the user interface control. This also adds the control to the stack, which can be
Example:
// Start the camera
controlCommand(“Camera”, “StartCamera”);
print(“Camera has started”);
closeControl( controlName )
Closed the user interface control and displayed
the previous control in the display stack.
Example:
CloseControl();
Variables
JavaScript variables are private to the namespace of each script engine. That means a variable created in a JavaScript script does not exist in other JavaScript scripts. For example, if you have variables declared in the JavaScript of the Camera control, the variables are not accessible in the JavaScript of the WiiMote control. However, you can share variables across scripts using global variable storage.
Public variables use ARC’s global variable storage manager, which is global across all robot skills and compilers. Public variables are accessed using the getVar(), setVar(), and setVarObject() JavaScript commands.
Blockly generates JavaScript, which means the variables will be private by default. The variables can start with a $ (dollar sign) to be public.
JavaScript Constants
A constant is a global variable available throughout the JavaScript environment within ARC. The variable value cannot be changed and is declared behind the scenes when your code is executed. These constant variables are also unique because they do not need to be surrounded by "quotes" when referenced. This is because their actual value is a number. Numbers (such as integers and decimal floats) do not need to be surrounded by quotes (although you may surround them with quotes if you prefer). Only string values need to be wrapped in quotes.
D0 ... D23
V0 ... V99
ADC0 ... ADC7
Custom JavaScript Extension
The ARC JavaScript engine exposes the ability to extend the built-in objects with user-defined custom objects. These custom objects can be either methods, variables, or entire classes. An example of doing this is in the Create Robot Skill manual. Access it by Clicking Here.
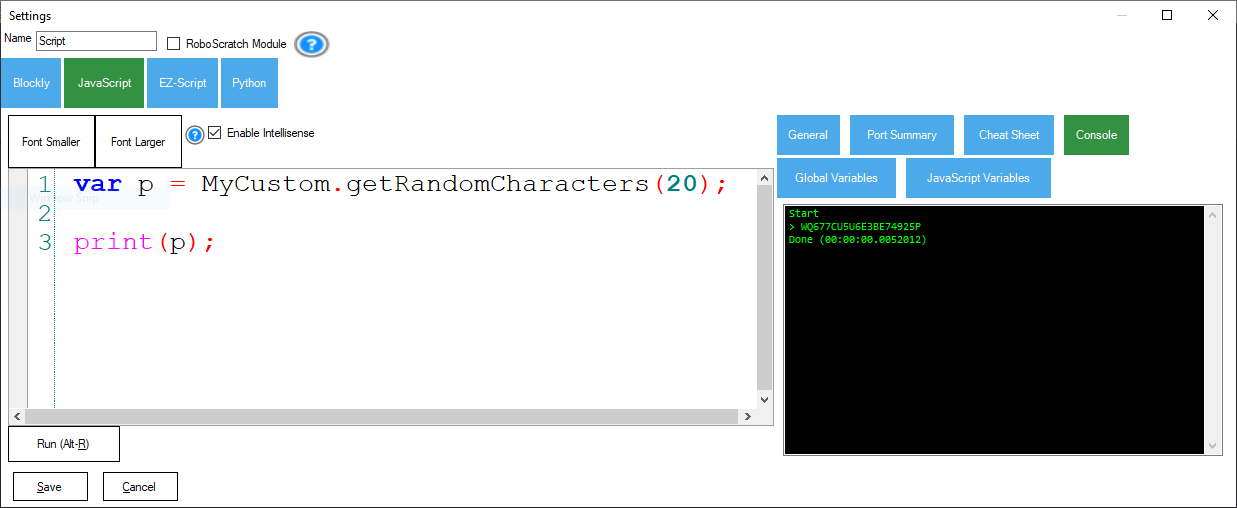