Add Custom Python Modules
Additional Python modules (.py
files) can be installed in the user's
My Documents\ARC\Python Modules folder.
Important Rules & Guidelines
- Pure Python Only: Only install modules written entirely in Python. Modules requiring C extensions or compiled components will not work with Synthiam ARC Python.
-
Proper File Structure: Ensure that each module is a single
.py
file or a properly structured package (i.e., a folder with an__init__.py
file). - Maintain Isolation: Place all custom modules within the designated folder so that they remain separate from other system or application modules.
- Version Compatibility: Verify that any module you install is compatible with the Synthiam ARC Python version you are using.
Using PIP with Synthiam ARC Python
PIP is a Python package installer that you can use to install Python modules. By default, PIP installs modules to a location different from the ARC folder. To have PIP install modules into your My Documents\ARC\Python Modules folder, follow these steps:
-
Locate or Create the PIP Configuration File:
-
Windows:
%HOME%\pip\pip.ini
(For example, if your username is Bob, this might beC:\Users\Bob\pip\pip.ini
.)
-
Windows:
-
Edit or Create the Configuration File:
Open the
pip.ini
(orpip.conf
) file in a text editor and add the following lines:[global] target=C:\Users\Bob\My Documents\ARC\Python Modules
Replace
C:\Users\Bob\My Documents\ARC\Python Modules
with the actual path to your ARC Python folder. -
Install Modules via PIP:
Run the PIP command from the c. PIP will now install any modules into the specified target directory.
-
Verify the Installation:
Confirm that the module files are present in the My Documents\ARC\Python Modules folder.
Creating an Example Module
Creating a Python module that prints "Hello, World!" is quite straightforward. You can create a Python file with a function that prints the desired message. Here's an example of a simple Python module named hello_world_module.py:
Please navigate to the My Documents\ARC\Python Modules folder and create a hello_world_module.py file. Enter the following code in the file.
# hello_world_module.py
def say_hello():
print("Hello, World!")
You can save this code in a file with the name hello_world_module.py. To use this module, you can import it into another Python script and call the say_hello function:
Now, add a SCRIPT robot skill to the project. Edit the script, switch to the Python tab, and insert this code.
# add to a python script in ARC
import hello_world_module
hello_world_module.say_hello()
When you run the Python script in ARC, it will import the hello_world_module and execute the say_hello function, which will print "Hello, World!" to the console output.
Example Module - TCP Client
This example module can be placed in the My Documents\ARC\Python Modules folder and named ARCClient.py (case sensitive is required). This example module will connect to the Synthiam ARC TCP Server, which can be enabled in the Connection robot skill. This script can also be added to any Python modules folder to use remotely from another machine. The ARC TCP Server uses EZ-Script so you'll need to reference the manual for ez-script here.
import socket
class ARCClient:
def __init__(self, server_ip, server_port):
self.server_ip = server_ip
self.server_port = server_port
self.sock = None
self.version = "unknown"
def connect(self):
self.sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
self.sock.connect((self.server_ip, self.server_port))
self.version = self._receive_response()
print("Version:", self.version.decode())
except Exception as e:
print("Connection error:", str(e))
def send_command(self, command):
if self.sock:
self.sock.sendall(command.encode())
response = self._receive_response()
return response.decode()
def close(self):
if self.sock:
self.sock.close()
self.sock = None
def _receive_response(self):
response = b''
while True:
data = self.sock.recv(1024)
if not data:
break
response += data
if b'\n' in data:
break
return response
def version(self):
return self.version
if __name__ == "__main__":
server_ip = '127.0.0.1'
server_port = 6666
command = "SayEZB(\"I am connected\")\n"
arc_client = ARCClient(server_ip, server_port)
arc_client.connect()
response = arc_client.send_command(command)
print("Received response:", response)
arc_client.close()
To use this module, add a Script robot skill to your project and place the following code...
from ARCClient import ARCClient
server_ip = '127.0.0.1'
server_port = 6666
command = "SayEZB(\"I am connected\")\n"
arc_client = ARCClient(server_ip, server_port)
arc_client.connect()
response = arc_client.send_command(command)
print("Received response:", response)
arc_client.close()
The TCP Server will also need to be enabled in the Connection robot skill.
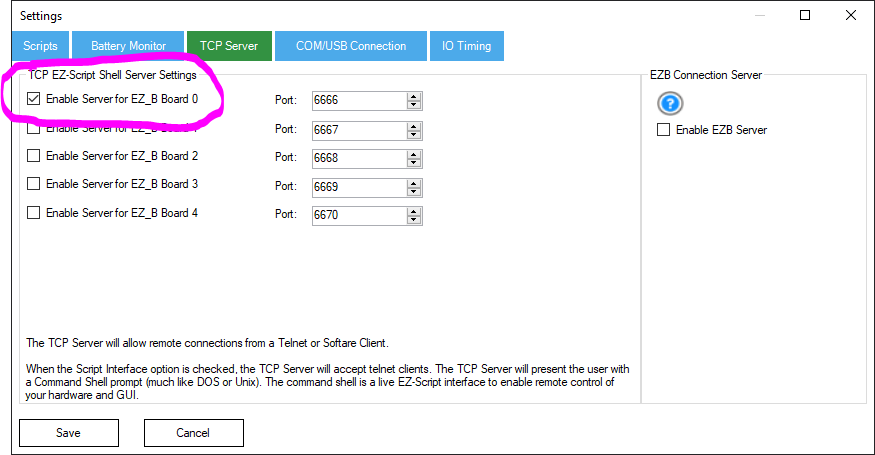