Example of how to create a plugin that becomes an ez-script function.
How to add the User Defined Function Example robot skill
- Load the most recent release of ARC (Get ARC).
- Press the Project tab from the top menu bar in ARC.
- Press Add Robot Skill from the button ribbon bar in ARC.
- Choose the Scripting category tab.
- Press the User Defined Function Example icon to add the robot skill to your project.
Don't have a robot yet?
Follow the Getting Started Guide to build a robot and use the User Defined Function Example robot skill.
How to use the User Defined Function Example robot skill
Add custom EZ-Script functions in c# using this example.
The source code is available here: UserDefinedFunctionExample.zip
Additional tutorial information can be found here: https://synthiam.com/Support/Create-Robot-Skill/Examples/Example-Custom-EZ-Script-Function
Optionally, you can create custom JavaScript functions with these instructions: https://synthiam.com/Support/Create-Robot-Skill/Examples/custom-javascript-extension
There is also an example code below the video.
using System;
using System.Drawing;
using System.Windows.Forms;
namespace User_Defined_Function_Example {
public partial class FormMaster : EZ_Builder.UCForms.FormPluginMaster {
public FormMaster() {
InitializeComponent();
}
private void FormMaster_Load(object sender, EventArgs e) {
// Intercept all unknown functions called from any EZ-Script globally.
// If a function is called that doesn't exist in the EZ-Script library, this event will execute
ExpressionEvaluation.FunctionEval.AdditionalFunctionEvent += FunctionEval_AdditionalFunctionEvent;
}
private void FormMaster_FormClosing(object sender, FormClosingEventArgs e) {
// Disconnect from the function event
ExpressionEvaluation.FunctionEval.AdditionalFunctionEvent -= FunctionEval_AdditionalFunctionEvent;
}
///
/// This is executed when a function is specified in any ez-scripting that isn't a native function.
/// You can check to see if the function that was called is your function.
/// If it is, do something and return something.
/// If you don't return something, a default value of TRUE is returned.
/// If you throw an exception, the EZ-Script control will receive the exception and present the error to the user.
///
private void FunctionEval_AdditionalFunctionEvent(object sender, ExpressionEvaluation.AdditionalFunctionEventArgs e) {
// Check if the function is our function (SetColor)
if (!e.Name.Equals("setcolor", StringComparison.InvariantCultureIgnoreCase))
return;
// Check if the correct number of parameters were passed to this function
if (e.Parameters.Length != 3)
throw new Exception("Expects 3 parameters. Usage: SetColor(red [0-255], green [0-255], blue [0-255]). Example: SetColor(20, 200,100)");
// Convert the parameters to datatypes
byte red = Convert.ToByte(e.Parameters[0]);
byte green = Convert.ToByte(e.Parameters[1]);
byte blue = Convert.ToByte(e.Parameters[2]);
// Do something
EZ_Builder.Invokers.SetBackColor(label1, Color.FromArgb(red, green, blue));
// Return something. Good idea to return TRUE if your function isn't meant to return anything
e.ReturnValue = true;
}
}
}
Related Questions
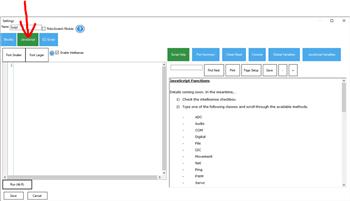
Scripts, Add Java Script
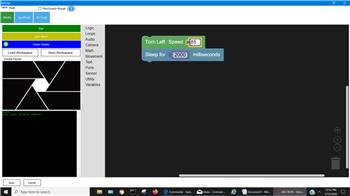
Turn Adventurebot Using Angles
Upgrade to ARC Pro
With ARC Pro, your robot is not just a machine; it's your creative partner in the journey of technological exploration.
I see that I can create a user-defined function in C#. But I don't know C#. So, can I create a user-defined function using EZ-Script? If so, what is the syntax? I searched up and down and did not see anything on it. Otherwise, I guess I can just use lots and lots of "Script" skills.
Maybe this will help. It's mentioned above: