Custom JavaScript Extension
A custom object can be assigned to the ARC JavaScript engine. This allows you to create custom methods that are exposed globally to the ARC framework. Once your robot skill is added to the project, the method will be available. This includes using the IntelliSense features within the ARC editor.
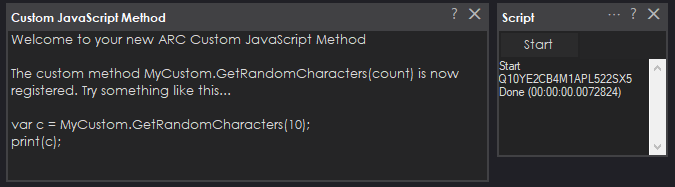
Download Example Source
Download the example source project for this robot skill here: Custom JavaScript Method.zip
The Robot Skill Code
Here is the main plugin code that subscribes to the OnSetValues event of the javascript engine. This event is raised every time the ARC JavaScript engine initializes. Your code is then responsible to assign an instance of your extension class to the engine. Lastly, when your robot skill is closed (removed from the project), you must unsubscribe from the OnSetValues event.
using System.Windows.Forms;
namespace CustomJavaScriptMethod {
public partial class MainForm : ARC.UCForms.FormPluginMaster {
public MainForm() {
InitializeComponent();
// Do not show the config button because this form has no user options
ConfigButton = false;
// Assign an event to apply the custom method(s) for the javascript engine
ARC.Scripting.JavaScript.JavascriptEngine.OnSetValues += JavascriptEngine_OnSetValues;
}
///
/// Event raised when the robot skill plugin is closed (i.e. removed from the ARC project)
///
private void MainForm_FormClosing(object sender, FormClosingEventArgs e) {
// Remove the event assignment when our robot skill form is closing
ARC.Scripting.JavaScript.JavascriptEngine.OnSetValues -= JavascriptEngine_OnSetValues;
}
///
/// This method is called by the OnSetValues event.
/// Every time the ARC JavaScript engine initializes, this method is called to assign our custom
/// extension method to the javascript engine.
///
private void JavascriptEngine_OnSetValues(ARC.Scripting.JavaScript.JavascriptEngine javascriptEngine) {
// Create an instance of our custom extension class
var extensionClass = new ExampleCustomJavaScriptExtension(javascriptEngine);
// Assign the extension class to the engine and give it the javascript object class name
javascriptEngine.JintEngine.SetValue(extensionClass.JavaScriptObjectName, extensionClass);
}
}
}
The Extension Code
As noticed in the robot skill plugin code above, the custom object is initialized and passed to the ARC JavaScript engine. While you can pass any object to the JavaScript engine, only extending the "DynamicCommandTemplate" will include a parent object (i.e. MyCustom.xxx) and Intellisense compatibility. You will notice this user-defined extension method defines itself in the MyCustom parent object namespace.
using System;
using System.Reflection;
using System.Text;
using ARC.Scripting.JavaScript;
namespace CustomJavaScriptMethod {
[Obfuscation(Exclude = true, ApplyToMembers = true)]
internal class ExampleCustomJavaScriptExtension : DynamicCommandTemplate {
///
/// Array of characters we will use for the GetRandomCharacters() method
///
const string _chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
///
/// This is the intializer which configures the extension to the engine
/// The parameters passed to the base class are...
/// 1) Engine (this is the instance of the arc javascript engine. Leave it as "engine"
/// 2) Object Name (this is the parent object name that your methods will be avaible under (i.e. MyCustom.getRandomCharacters)
/// 3) Friendly Description (the friendly description that is displayed to the user in the intellisense syntax editor within ARC)
///
public ExampleCustomJavaScriptExtension(JavascriptEngine engine) : base(engine, "MyCustom", "My Custom Methods") {
}
///
/// If you need to dispose anything, do it in here
///
protected override void DisposeOverride() {
}
///
/// One of the custom methods that is exposed to the engine under the MyCustom namespace
///
public string GetRandomCharacters(int count) {
StringBuilder sb = new StringBuilder(count);
var r = new Random();
for (int x = 0; x < count; x++)
if (Engine.CancelRequested)
return string.Empty;
else
sb.Append(_chars[r.Next(_chars.Length)]);
return sb.ToString();
}
}
}
Running The Code
Once you have successfully created a robot skill with the above example, you may now test it. In order for your custom JavaScript extension method(s) to be registered, the robot skill must be added to the ARC project.
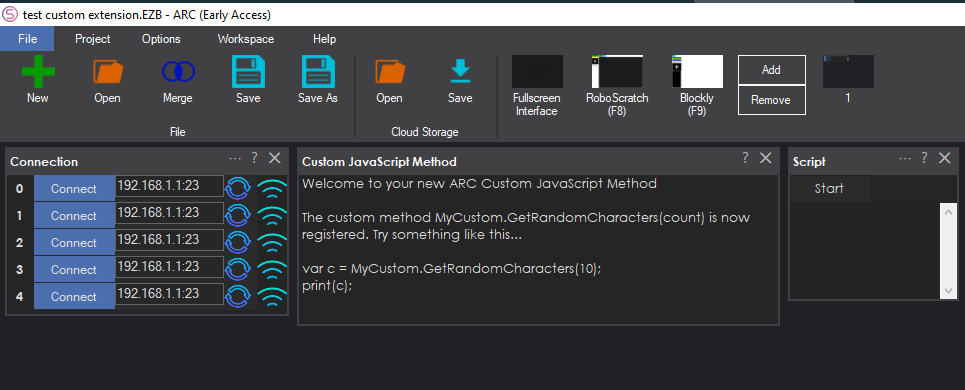
Now you can load a script robot skill and use the JavaScript tab to write JavaScript code. In the above example, you may call the custom user-defined method and see the results.
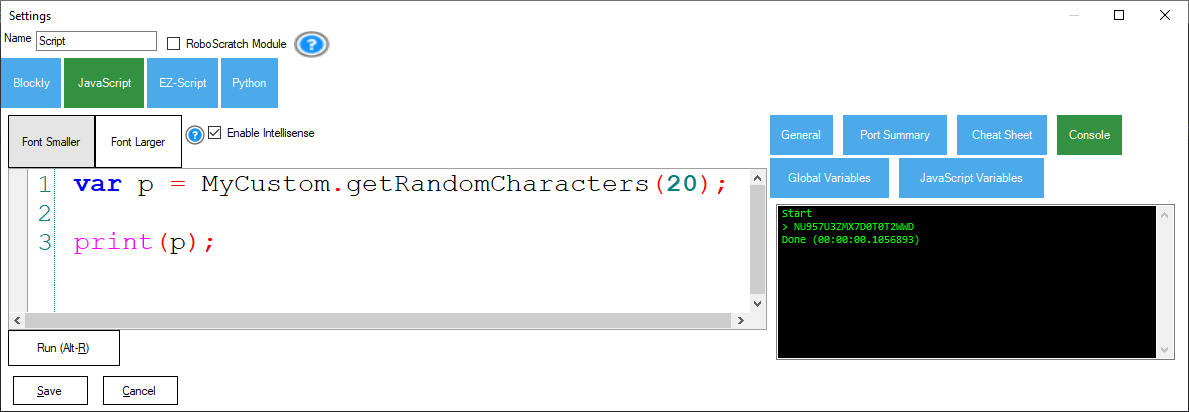