ControlCommand()
There are plenty of windows within the ARC project desktop, and each window is a robot skill control. A robot skill control is a little program that runs within ARC created by one of our many partners. For example, there are hundreds of available robot skill controls in ARC, such as gpt-3, vision detection (face, emotion, color, glyph, etc.), servo gait/animation, speech recognition, etc. Each robot skill control is a behavior that gives your robot more ability. The most common robot skill controls are Connection, Camera, and Auto Position. These robot skill controls are separate programs that do something specific, such as processing video image data from the camera or moving servos in animations. Because each robot skill control is a particular program, ARC provides a mechanism for robot skills to talk to each other. This mechanism is the script ControlCommand(). Using this command, an event of one control can instruct another robot skill to do something. For example, if the Speech Recognition control detects the phrase "Follow My Face," the respective code may be instructed to inform the Camera control to enable Face Tracking.
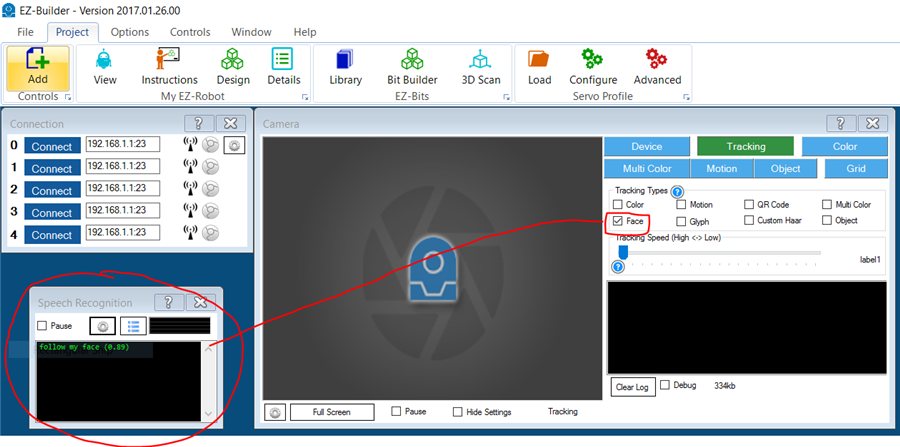
This detailed example detects speech recognition text and uses cognitive services for more extensive messages. The manuals for each robot skill will be essential to review when creating projects.
In The Code
In the above example, the "Follow My Face" phrase within the Speech Recognition robot skill control instructed the Camera to begin using Face Tracking. Let's look at the code within the Speech Recognition robot skill that made this happen. Below, you will see the Blockly and respective script assigned to the "Follow My Face" within the Speech Recognition robot skill.
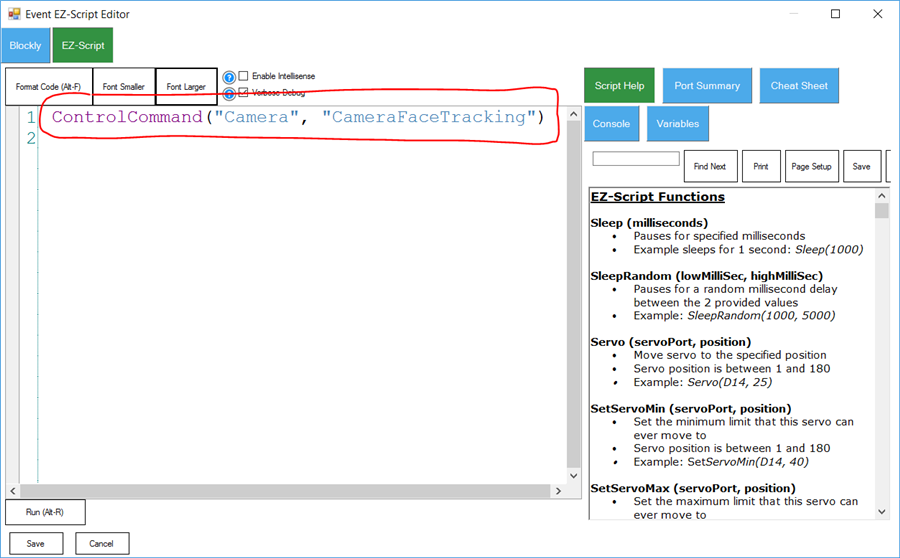
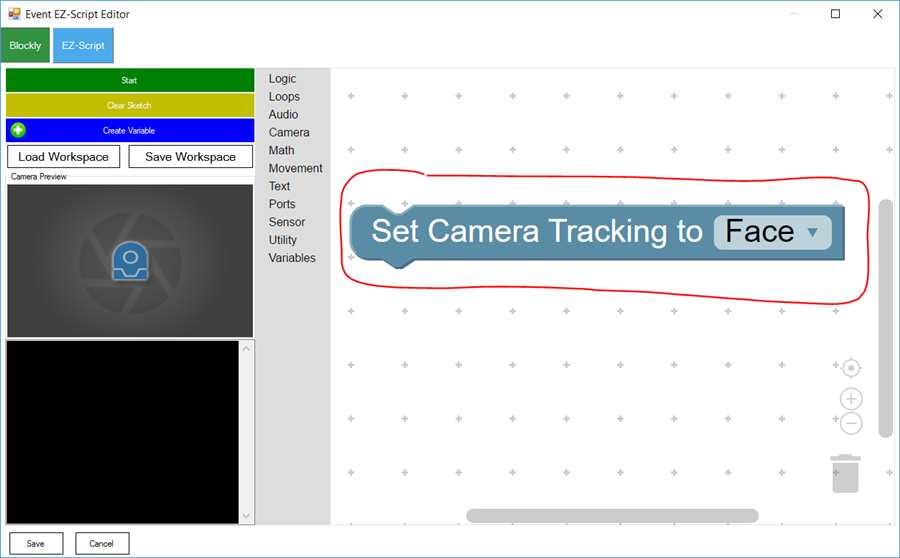
What Commands Are There?
Each robot skill will accept a certain number of ControlCommand()s. Not all robot skills take the same commands. ARC will query each robot skill and ask, "What control commands do you accept?" and create a list for you. The list is presented in three places. Here are screenshots of each location to find the available ControlCommands() for all robot skills within the project.
The Cheat Sheet tab is displayed when editing the script.
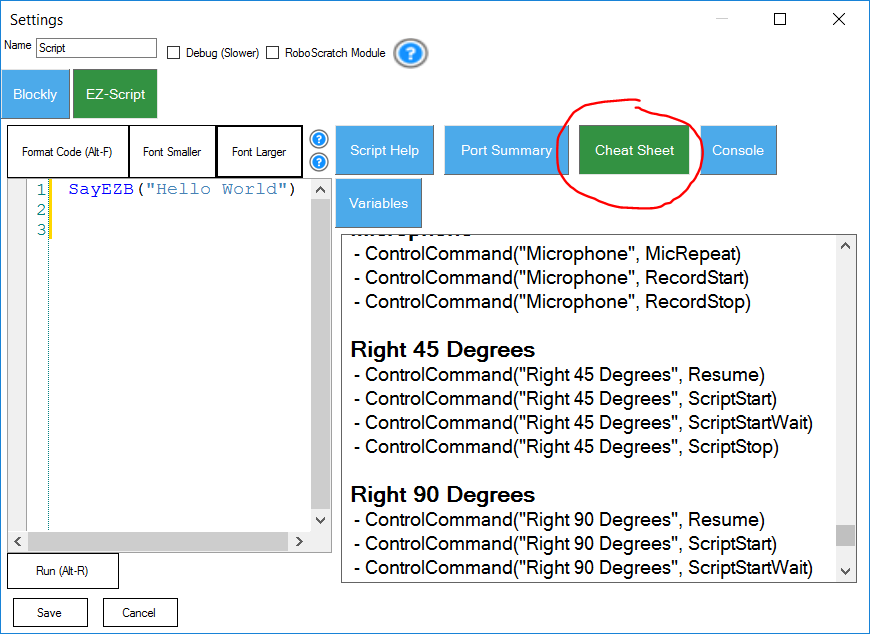
Right-Click in the editor when editing the script
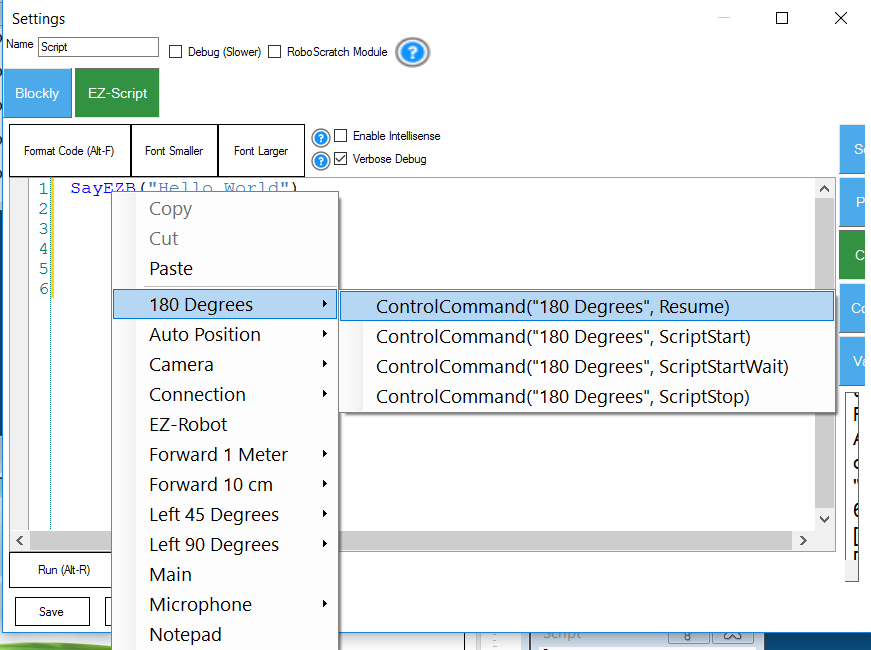
Blockly provides a block in the Utility category.
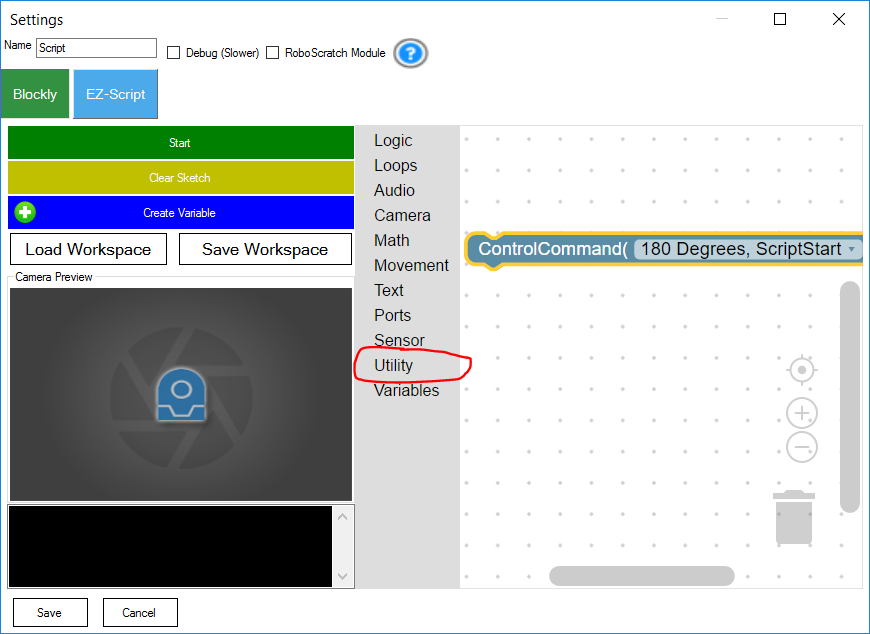
How Does It Work?
Behind the scenes, ARC has a Robot Skill Control Manager who knows all the robot skills within the project. The Robot Skill Control Manager knows each robot skill by its name. Robot skill controls are given a unique name by the Robot Skill Control Manager when added to a project. Although, you may also edit the name of robot skill controls within their configuration page. The ControlCommand() requires the name of the robot skill it will speak to as the first parameter. All other parameters depend on the robot's skill as presented in the previous step (Cheat sheet, right-click, blockly).
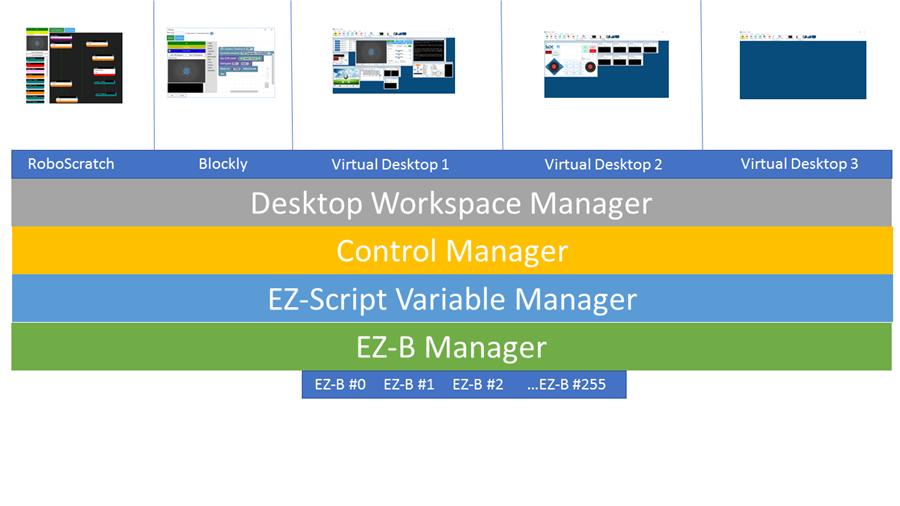
ControlCommmand() Never Waits
An essential piece of information regarding ControlCommand() is that it does not wait until the destination robot skill has completed executing the command. For example, imagine your script was instructing an Auto Position robot skill to begin the action "Wave." The ControlCommand JavaScript code may look like this...
controlCommand("Auto Position", "AutoPositionAction", "Wave"); Audio.SayEZB("I am waving");
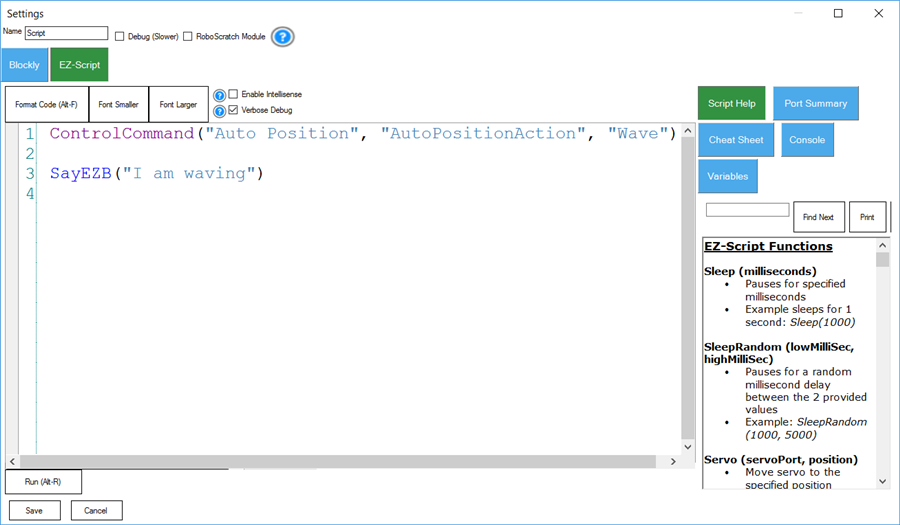
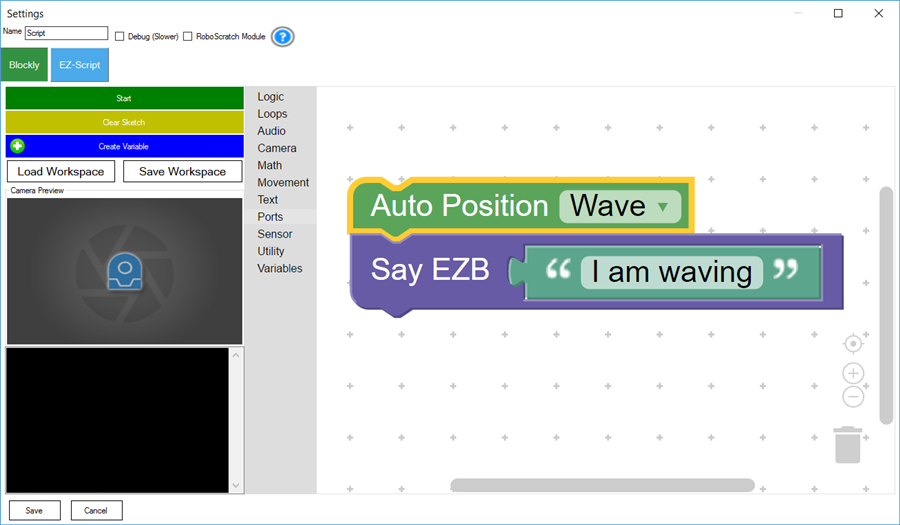
The above code instructed the Auto Position to wave and immediately spoke the phrase "I am waving" out of the EZ-B's speaker. The script did not wait for the Wave action to complete before speaking the phrase. This is because the Auto Position robot skill is a separate program. The script used ControlCommand() to instruct the Auto Position to execute the Wave action and immediately moved onto the following line of code without waiting for the Auto Position Wave to complete. This is because ControlCommand() is one direction. The ControlCommand() can only nudge another control by saying, "Hey, do this...." and does not receive a response.
What If I Want To Wait?
The ControlCommand() instructs another control to "do something" and does not wait for it to finish. The only exception to this rule is when the ControlCommand() parameter specifies that it waits. An example would be the Scripting control. The Scripting robot skill accepts a unique ControlCommand() parameter "ScriptStartWait," which will wait for the script to complete. Very few ControlCommands() behave this way, and those that do will have "Wait" specified in the parameter name. This is because ControlCommand() is generally a non-blocking method.
The Auto Position Wave action executes in the previous step, and the code is immediately executed. If you wanted to wait until the robot completed the Wave in the Auto Position, you could still do that. Some robot skills will populate variables that hold their operation status. Meaning some robot skills will create a variable (i.e., $AutoPositionStatus) which is TRUE (1) or FALSE (0) regarding the status. In the above scenario, we can wait for the Auto Position to complete by monitoring the variable. It is essential to notice that not all robot skills provide a status variable. The robot skill controls providing a status variable will generally display the variable on its configuration settings page. Otherwise, viewing all variables within the project can be done using the Variable Watcher.
Both RoboScratch and Blockly provide functions that demonstrate how to wait for the most common tasks, such as waiting for an Auto Position Action to complete or a Sound to complete. The RoboScratch and Blockly commands that wait will always explicitly specify "(Wait)" in the command title. Here is an example of Blockly and JavaScript waiting for an Auto Position to complete before speaking a phrase.
controlCommand("Auto Position", "AutoPositionAction", "Wave"); sleep(500); while (getVar("$AutoPositionStatus")); Audio.SayEZB("I finished waving");
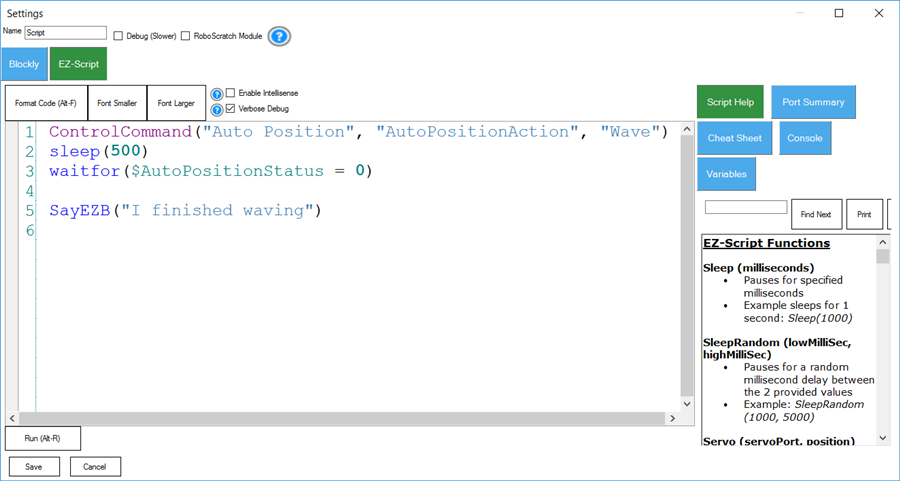
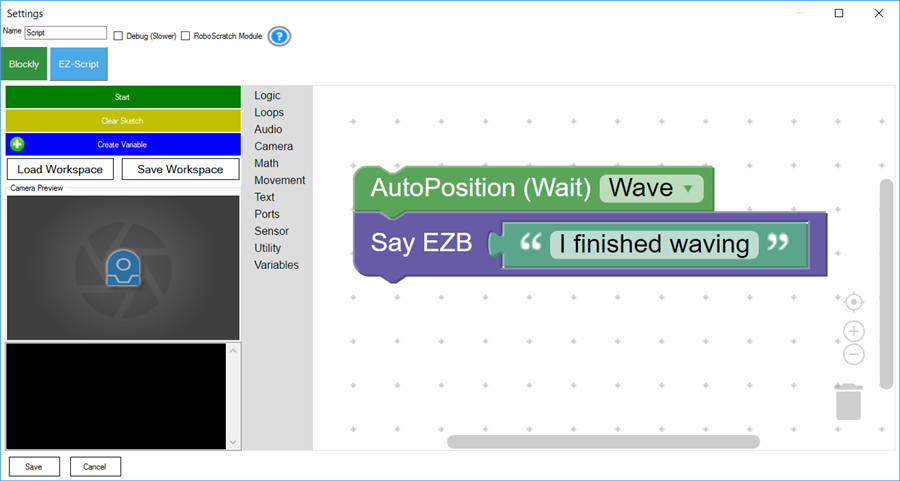
Examining the above code, one will notice the familiarity of the ControlCommand() instructing the Auto Position to execute the Wave action. However, the next two lines of code are new. The Sleep(500) provides a short delay to give the Auto Position robot skill enough time to execute the instruction. The following line WaitFor(getVar("$AutoPositionStatus")) will wait forever until the specified condition is met. In this case, the specified condition is $AutoPositionStatus = 0, which means the Auto Position robot skill control is no longer active. This while() will wait until the Auto Position has completed because the Auto Position will set the $AutoPositionStatus value to FALSE (0) when it has. Finally, the last line of the script will speak after the Auto Position has been completed.