5. Add Some Buttons
Visual Studio 2022 Warning
As of 2022/02/01, there is a bug with Microsoft Visual Studio 2022 edition. We recommend installing the Visual Studio 2019 Community edition as a replacement. Microsoft is aware of the bug and has not decided to fix it yet. This message will be removed when/if Microsoft fixes the bug. You can read more about the bug HERE. If this is an inconvenience, feel free to contribute your feedback to the bug report on the bug report link.
1) Locate the MainForm in the Solution Explorer and double click.
2) When the MainForm designer loads, click on the text and press Del to remove the label from the form. This will leave you with a blank form.
3) Locate the Button under All Windows Forms in the Toolbox. Drag two buttons anywhere onto your MainForm.
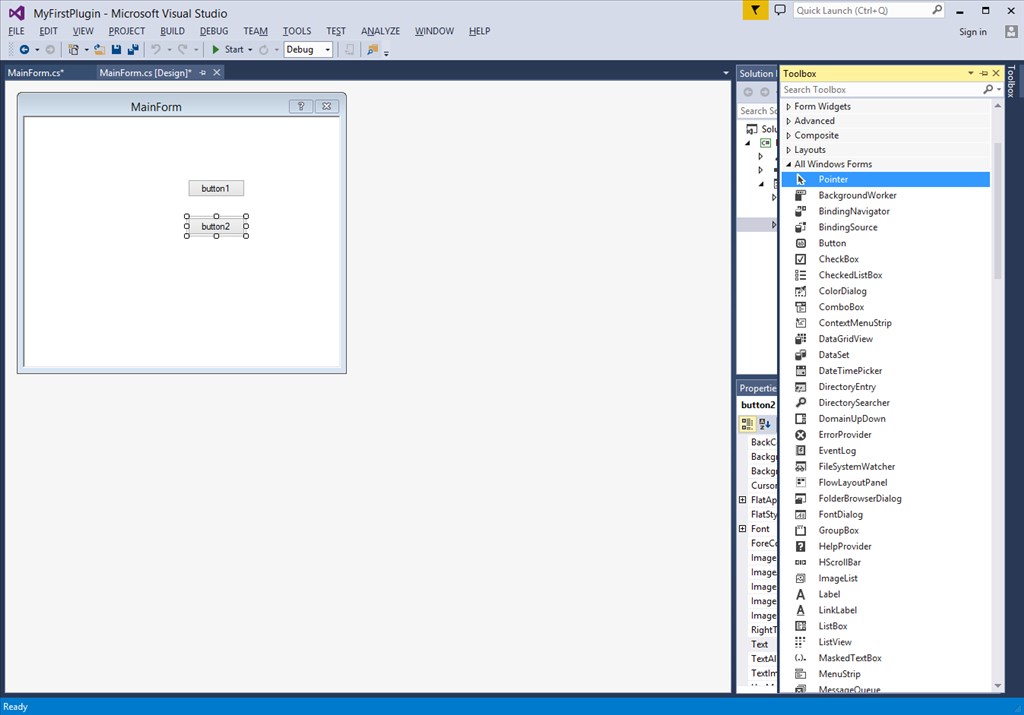
3) Give the buttons readable text that tells the user what they will do. In this tutorial example, we will be programming the buttons to move a servo between two positions. Click on each button and locate the Text field in the properties window.
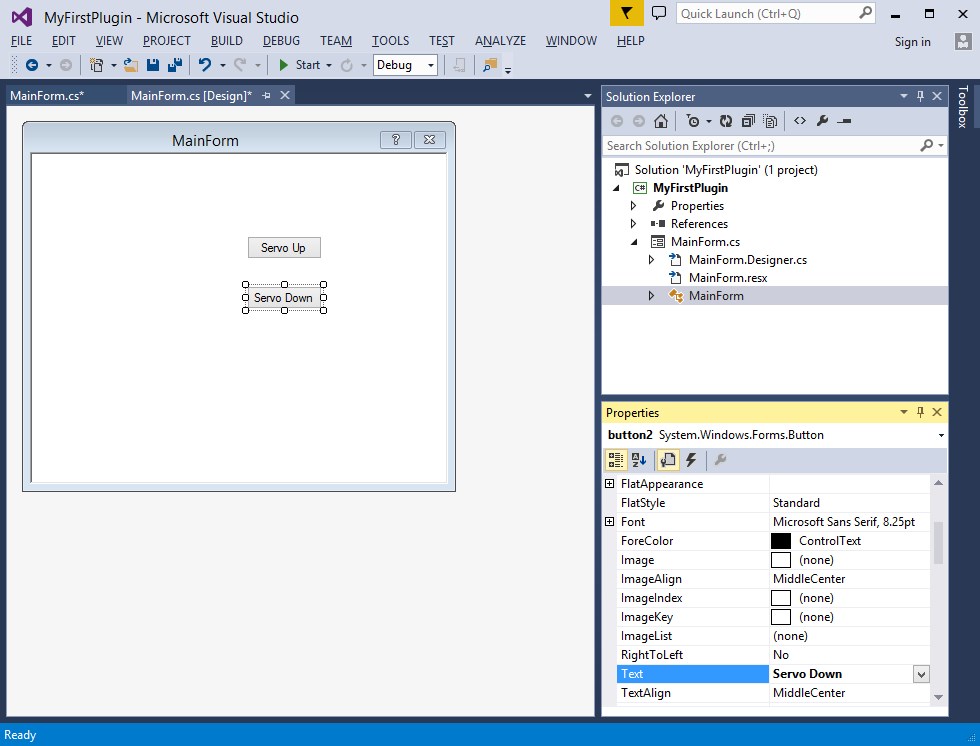
4) Double click on each button in the Designer and code will automatically be generated for the Click event of each button. This means that when a user clicks on the button, the code within the function will be executed. The functions are automatically inserted into your code when you double click on them from the designer.
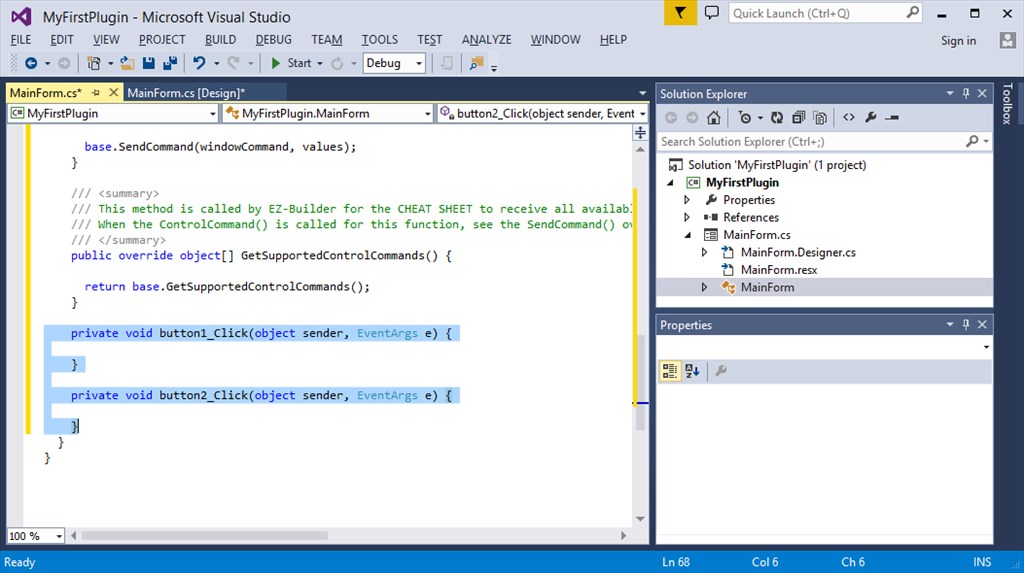
5) Insert code into each of the button click events to move a servo. The command to move a servo is located within an EZB class. Because ARC allows more than one EZB connection, the list of EZB's available is an array. It is safe to assume that the first EZB is used the most. Here is the code which will move the servo connected on the EZ-B port D0 between position 10 degrees and 170 degrees when the buttons are pressed.
Code:
private void button1_Click(object sender, EventArgs e) {
ARC.EZBManager.EZBs[0].Servo.SetServoPosition(EZ_B.Servo.ServoPortEnum.D0, 10);
}
private void button2_Click(object sender, EventArgs e) {
ARC.EZBManager.EZBs[0].Servo.SetServoPosition(EZ_B.Servo.ServoPortEnum.D0, 170);
}
6) When your code has been entered, it will now look like this.
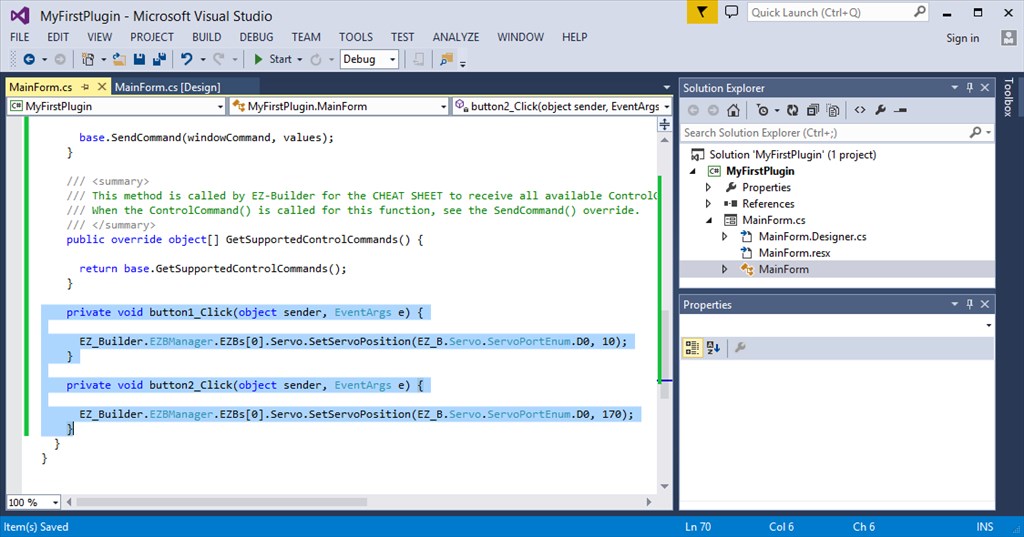
7) Let's compile your project to ensure there are no errors before continuing to the next step. Press CTRL-SHIFT-B and watch the Output window for any error messages. If everything compiles okay, you will see a similar message result to the screenshot below.
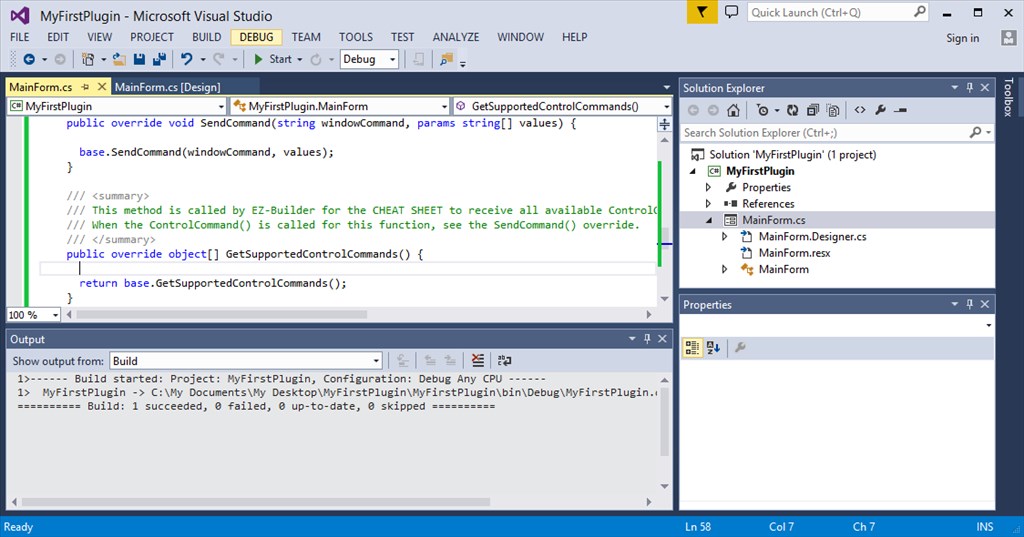
8) Assuming you have no errors and everything compiles fine, press F5, the project will compile and load ARC. Add the plugin to your workspace and test it out!