ControlCommand() Binding
OverviewThe ControlCommand() is a scripting function which enables users to send commands and parameters to supporting controls from another control. It is how controls communicate. Each control broadcasts a list of commands it supports. These commands are displayed in the Cheat Sheet while users are editing scripts (JavasScript or EZ-Script). When a ControlCommand() is executed that has a destination name matching your plugin, an event is triggered in which you will respond.
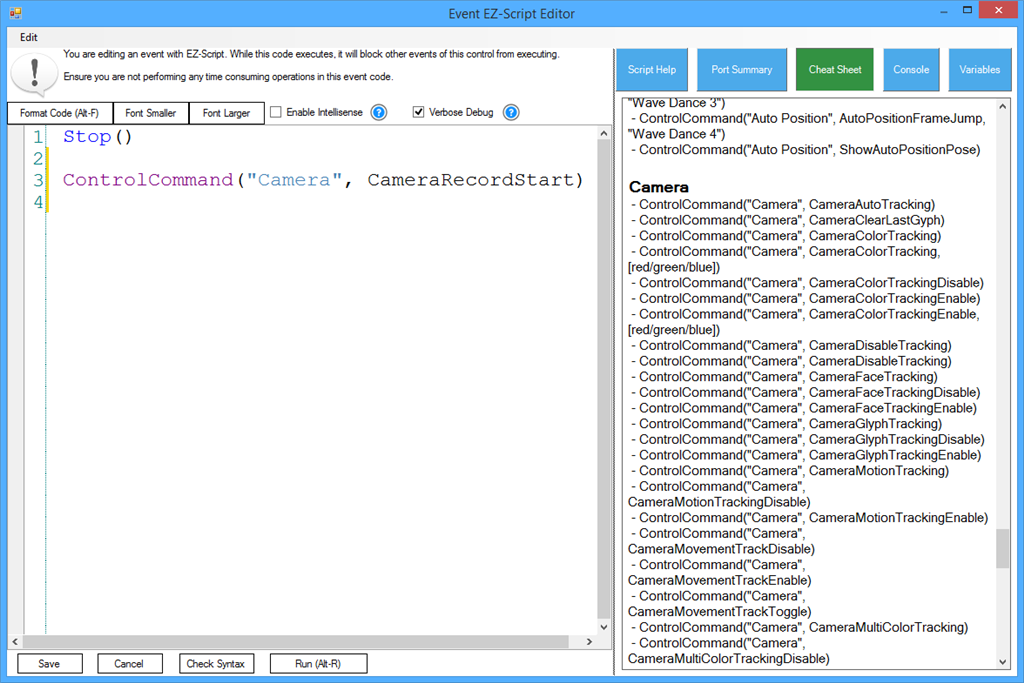
An Example
An example of a popular ControlCommand() is starting the video feed in the camera control. Users will add Script Control to their program which instructs the Camera Control to begin streaming video from the specified video source.
Code:
# JavaScript example to start the video feed on a camera control
controlCommand("Camera Control", "CameraStart");
You may notice that some ControlCommand() will accept optional parameters. The CameraStart also has an optional parameter, which is the device name.
Code:
# JavaScript example to start the video feed on a camera control specifying device name
controlCommand("Camera Control", "CameraStart", "EZB://192.168.1.1:24");
Bind To ControlCommand()
Now that the user is aware of the supported options available in your Cheat Sheet, we will bind to the script engine for any calls directed to your control. This is done through an override method which will be raised in the event that a ControlCommand() matches your control.
Some facts to note in this example code...
1) Comparison is case insensitive. We have no idea what case the text will be entered by the user.
2) If no commands match your syntax, the Base() method will notify the script engine.
3) If expected parameters are missing or incorrect, you may throw an exception which will be caught by the parent script engine.
4) To avoid cross-threading exceptions, there is a fancy helper class ARC.Invokers which contains methods to manipulate user controls from different threads. The SendCommand() event will always be called from a background thread. This is because the script engine will never execute threads on a GUI thread.
Code:
public override void SendCommand(string windowCommand, params string[] values) {
if (windowCommand.Equals("PauseOn", StringComparison.InvariantCultureIgnoreCase)) {
ARC.Invokers.SetChecked(checkbox1, true);
if (values.Length == 1)
ARC.Invokers.SetText(checkbox1, values[0]);
else if (values.Length > 1)
throw new Exception(string.Format("Only 0 or 1 parameters expected. You passed {0}", values.Length));
} else if (windowCommand.Equals("PauseOff", StringComparison.InvariantCultureIgnoreCase)) {
ARC.Invokers.SetChecked(checkbox1, false);
if (values.Length == 1)
ARC.Invokers.SetText(checkbox1, values[0]);
else if (values.Length > 1)
throw new Exception(string.Format("Only 0 or 1 parameters expected. You passed {0}", values.Length));
} else {
base.SendCommand(windowCommand, values);
}
}
Conclusion
By using the ControlCommand(), users can send commands to your plugin or configure settings, all from scripts. This gives your plugin the ability to be better customized for the users needs programmatically.
Blockly
ControlCommand() are usable in Blockly UI, with one exception. Because the Blockly UI does not contain the ability for user defined parameters of the ControlCommand() feature, they are limited to commands with no user parameters. This means that a ControlCommand() with parameters will not display in the blockly UI.
The ControlCommand() for blockly is found in the Utility category.
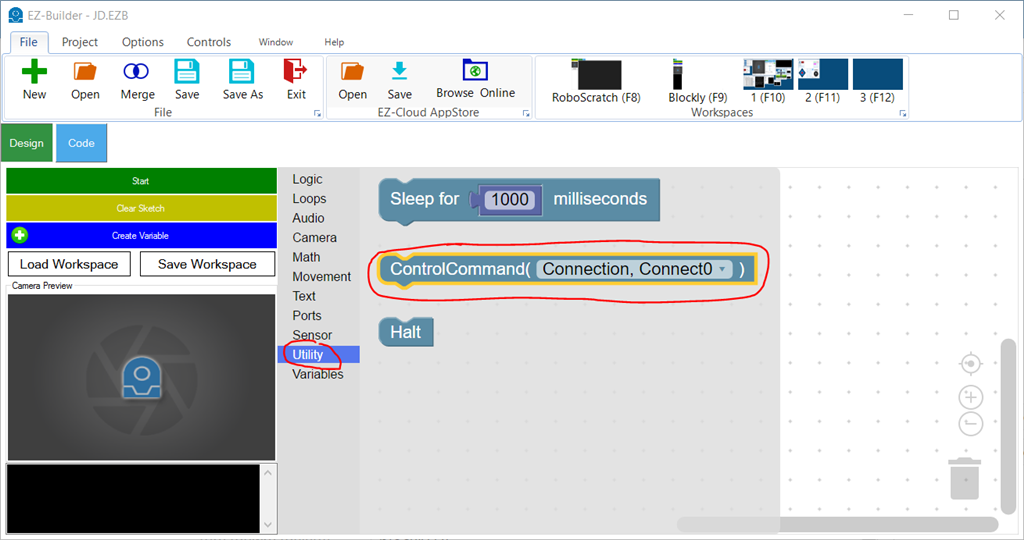
Viewing the available ControlCommand()'s within blockly, you will see that commands accepting user parameters are not displayed..
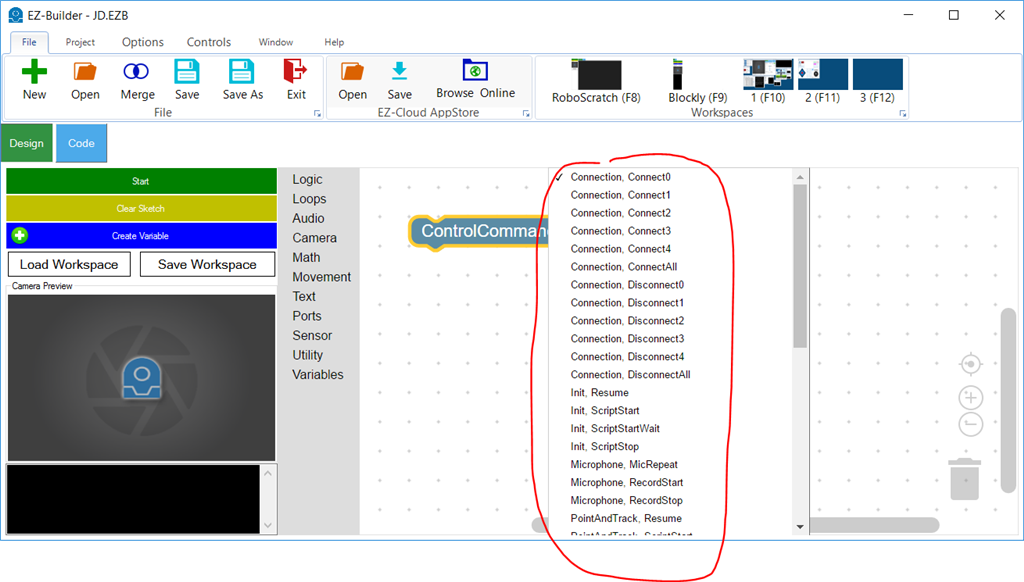
To further the example, here are two control commands in which one will be displayed, and one will not be
Code:
// This will be displayed in blockly
controlCommand("My Control", "SetColorRed");
controlCommand("My Control", "SetColorGreen");
// These will not be displayed in blockly because it accepts a user parameter
controlCommand("My Control", "SetColor", "Red");
controlCommand("My Control", "SetColor", "Green");