Slow Performance
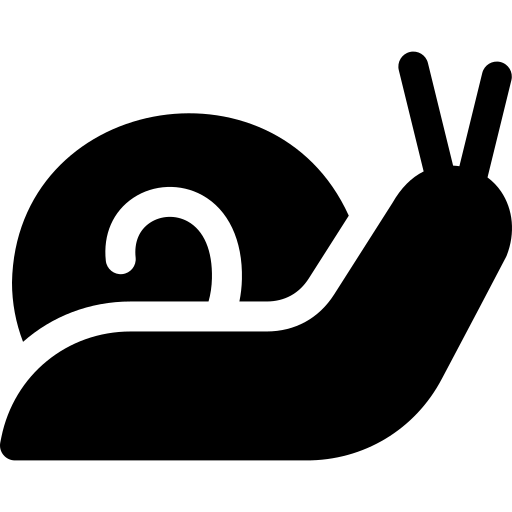
ARC was designed to prioritize robot skills and their respective compilers in a multi-threaded environment. This ensures that each robot skill and separate event scripts are executed in isolated threads. This approach provides robot skills, and scripts distribute CPU time for a responsive UI and robot program. Even with ARC's multi-thread architecture, users can create scripts and project configurations that cause sluggish behavior. This robot support document outlines common issues that will result in low performance.
Contents
- Tight loops
- Flooded EZB Communication Channel
- Sensor/Peripheral Timeout
- Abusing ControlCommand()
- High Camera Resolution
Tight Loops
A tight loop is a programming term. Such a loop heavily uses I/O or processing resources, failing to adequately share them with other programs running in the operating system. An example of a tight loop would be a script that loops indefinitely without sleep() to relieve the CPU and give other threads more CPU time. It is also worth noting that a tight loop may also affect EZB communication performance if the loop commands are reading from the EZB or the peripheral sensor is timing out (see Flood EZB Communication Channel and Peripheral Timeout).
JavaScript Example
while (true) {
// Read the ADC value of the pot
var adcVal = ADC.get(adc0);
// Map the ADC value (0-255) to the servo degrees (1-180)
var servoPos = Utility.map(adcVal, 0, 255, 1, 180);
// Move the servo into the position
Servo.setPosition(d0, servoPos);
}
while (true) {
// Read the ADC value of the pot
var adcVal = ADC.get(adc0);
// Map the ADC value (0-255) to the servo degrees (1-180)
var servoPos = Utility.map(adcVal, 0, 255, 1, 180);
// Move the servo into the position
Servo.setPosition(d0, servoPos);
// Give CPU time to other threads
sleep(100);
}
The primary question for resolving tight loops by adding a sleep() is, "How long should my sleep command be for?". The priority of your software loop determines the answer to that question. The loop only needs to run four times a second or less in many cases. For a script that runs four times per second, a sleep(250) command would suffice. The sleep() command parameter is milliseconds (MS), and there is 1,000 MS in a second.
TopFlooded EZB Communication Channel
EZBs can be connected through Serial USB or Wi-Fi. Generally, the Serial USB is fast with very low latency and rarely experiences a flooded communication channel. However, it is common for WiFi EZBs to slow ARC scripts and robot skills from flooding the communication channel if data is being read too often or read redundantly. When data is read too often with a WiFi EZB, the overhead of the TCP stack and WiFi protocol will cause slight delays. Because EZB read commands are blocking, they will block all other EZB communication until a response is returned. There are four common mistakes made that may flood the communication channel.
-
Redundant Robot Skills
A project contains many robot skills that pull data from the same port/peripheral. For example, two or more Read ADC robot skills may display ADC data from the same port. This will double the EZB communication and therefore double the latency. The solution would be to limit the number of robot skills querying data from the EZB by removing duplicates.
-
Multiple Robot Skills/Scripts Reading Same Port/Peripheral
There are visual robot skills for displaying ADC and Digital port statuses. These robot skills are excellent for debugging and educational use by providing visual feedback. However, it is common for ARC users to add a Read ADC robot skill and use Read ADC commands in scripts. In this case, both the robot skill and scripts are querying the same port, doubling the EZB read commands on the communication channel and latency. There are a few solutions for this scenario.
- You may remove the visual robot skill (i.e., Read ADC, Ultrasonic Distance) and continue using the script that references the port.
- Check if the robot skill (i.e., Read ADC, Ultrasonic Distance) populates a variable with the port value and uses that port value in your script. Many robot skills, such as Read ADC, Ultrasonic Distance, and Read Digital, will populate variables that can be referenced in scripts.
- If a GUI to present the port statuses is essential to the project, consider populating a global variable in a script and displaying the variable statuses on a custom User Interface Builder form.
-
Tight loops
A tight loop may be querying a port too quickly and not providing CPU time or EZB communication access. (See the Tight loops section.)
-
Slow Sensor Protocol
Some sensors and peripherals will have slow protocols. Two such examples are ultrasonic distance sensors and I2C devices. Ultrasonic distance sensors can take many milliseconds to respond with a valid value and even longer when experiencing a timeout. If an ultrasonic distance sensor does not detect an object because it is out of range, the sensor will timeout, which takes a long time. A timeout will block all other EZB communication until the timeout is completed. This scenario is further amplified when many robot skills and scripts attempt to read from the problematic sensor, as they will block all other communication.
The solution is to choose sensors that suit the performance requirements of your robot or use a dedicated EZB for low-latency sensors. If the performance is affected by ultrasonic sensors, consider adding all ultrasonic distance sensors to an affordable USB Arduino EZB or WiFi ESP32 EZB would allow the primary EZB not to experience blocking issues.
-
Sensor/Peripheral Timeout
A sensor or peripheral connected to the EZB may be timing out. (See the Sensor/Peripheral Timeout section.)
Sensor/Peripheral Timeout
Sensors and peripherals connected to the EZB may use a protocol that consumes time or times out if not connected. Two such examples are ultrasonic distance sensors and I2C devices. Ultrasonic distance sensors can take many milliseconds to respond with a valid value and even longer when experiencing a timeout. If an ultrasonic distance sensor does not detect an object because it is out of range, the sensor will timeout, which takes a long time. A timeout will block all other EZB communication until the timeout is completed. This scenario is further amplified when many robot skills and scripts attempt to read from the problematic sensor, as they will block all other communication.
The solution is to choose sensors that suit the performance requirements of your robot or use a dedicated EZB for low-latency sensors. If the performance is affected by ultrasonic sensors, consider adding all ultrasonic distance sensors to an affordable USB Arduino EZB or WiFi ESP32 EZB would allow the primary EZB not to experience blocking issues.
TopAbusing ControlCommand()
The ControlCommand() script command will send an instruction to another robot skill in the ARC project (read more here). In most robot skills, the available ControlCommands can also be accessed with GUI button presses. If a tight loop or script rapidly calls another robot skill via the ControlCommand, it would be similar to rapidly pressing the UI button. Consider how often your robot skill needs to call another robot skill to trigger an event. Specifically, ensure that your loop has given the other robot skill enough time to complete the task. Otherwise, you may interrupt the other job preventing it from ever completing.
TopHigh Camera Resolution
Machine vision and computer recognition are very highly CPU-intensive processes. The cameras for computer vision provide much less resolution than what you, as a human, would use for recording a birthday party. If you were to run computer vision to recognize objects and decode frames at HD quality, your computer response would grind to a halt. Let us examine how much data is contained in a video stream at varying resolutions.
- 160x120 = 57,600 Bytes per frame = 1,152,000 Bytes per second
- 320x240 = 230,400 Bytes per frame = 4,608,000 Bytes per second
- 640x480 = 921,600 Bytes per frame = 18,432,000 Bytes per second
*Note: at 320x240, your CPU is processing complex algorithms on 4,608,000 Bytes per second. Soon as you move to a mere 640x480, it's 18,432,000 Bytes per second.
To expand on this example, 4,608,000 Bytes per second is just the data, not including the number of CPU instructions per step of the algorithm(s). Do not let television shows, such as Person Of Interest, make you believe that computer vision and CPU processing areas are accessible in real time, but many of us are working on it! We can put 4,608,000 Bytes into perspective by relating that to a 2-minute MP3 file. Imagine your computer processing a 2-minute MP3 file in less than 1 second - that is what vision processing for recognition is doing at 320x240 resolution. Soon as you increase the resolution, the CPU has to process an exponentially more significant amount of data. Computer vision recognition does not require as much resolution as your human eyes, as it looks for patterns, colors, or shapes.The solution is to choose a camera device with video quality that suits the object's needs to be recognized. If your robot project operates correctly with a resolution of 320x240, it is advised to continue using that resolution.
Top