Example: Output Audio from EZ-B
This is an example with source code about how to play audio out of the EZ-B. The EZ-B will output audio as a stream or byte array.
This example can be run as a plugin by installing it here: http://www.ez-robot.com/EZ-Builder/Plugins/view/202
Source code as a plugin project is here: OutputAudioFromEZ-BSource.zip
*Dependency: Additional to adding EZ-Builder.exe and EZ-B.DLL, this plugin requires NAudio.DLL library to be added as a project reference. Remember to UNSELECT "Copy Files"!
This plugin provides the following examples:
- Load audio from MP3 or WAV file
// MP3
NAudio.Wave.Mp3FileReader mp3 = new NAudio.Wave.Mp3FileReader(openFileDialog1.FileName);
// WAV
NAudio.Wave.WaveStream wav = new NAudio.Wave.WaveFileReader(openFileDialog1.FileName);
- Convert audio file to uncompressed PCM data to supported EZ-B sample rate and sample size
NAudio.Wave.WaveFormatConversionStream pcm = new NAudio.Wave.WaveFormatConversionStream(new NAudio.Wave.WaveFormat(EZ_B.EZBv4Sound.AUDIO_SAMPLE_BITRATE, 8, 1), mp3);
- Compress PCM data with gzip to be stored in project STORAGE
using (MemoryStream ms = new MemoryStream()) {
using (GZipStream gz = new GZipStream(ms, CompressionMode.Compress))
pcm.CopyTo(gz);
_cf.STORAGE[ConfigTitles.COMPRESSED_AUDIO_DATA] = ms.ToArray();
}
- Play audio data from compressed project STORAGE
using (MemoryStream ms = new MemoryStream(compressedAudioData))
using (GZipStream gz = new GZipStream(ms, CompressionMode.Decompress))
EZBManager.EZBs[0].SoundV4.PlayData(gz);
- Supports ControlCommand() for Play and Stop of audio to be used in external scripts
public override object[] GetSupportedControlCommands() {
List items = new List();
items.Add(ControlCommands.StartPlayingAudio);
items.Add(ControlCommands.StopPlayingAudio);
return items.ToArray();
}
public override void SendCommand(string windowCommand, params string[] values) {
if (windowCommand.Equals(ControlCommands.StartPlayingAudio, StringComparison.InvariantCultureIgnoreCase))
playStoredAudio();
else if (windowCommand.Equals(ControlCommands.StopPlayingAudio, StringComparison.InvariantCultureIgnoreCase))
stopPlaying();
else
base.SendCommand(windowCommand, values);
}
- Changes the status of the button when audio is playing globally from anywhere in ARC on EZ-B #0
public FormMain() {
InitializeComponent();
EZBManager.EZBs[0].SoundV4.OnStartPlaying += SoundV4_OnStartPlaying;
EZBManager.EZBs[0].SoundV4.OnStopPlaying += SoundV4_OnStopPlaying;
}
private void FormMain_FormClosing(object sender, FormClosingEventArgs e) {
EZBManager.EZBs[0].SoundV4.OnStartPlaying -= SoundV4_OnStartPlaying;
EZBManager.EZBs[0].SoundV4.OnStopPlaying -= SoundV4_OnStopPlaying;
}
private void SoundV4_OnStopPlaying() {
Invokers.SetText(btnPlayAudio, "Play");
}
private void SoundV4_OnStartPlaying() {
Invokers.SetText(btnPlayAudio, "Stop");
}
Output Text to Speech You can output text to speech easily as well, using the following code example...
using (MemoryStream s = EZBManager.EZBs[0].SpeechSynth.SayToStream("I am speaking out of the EZ-B))
EZBManager.EZBs[0].SoundV4.PlayData(s);
Thanks for the quick response.
This what happens when you are working on robotics when its way past your bed time.
You miss the obvious
No problem - i get it
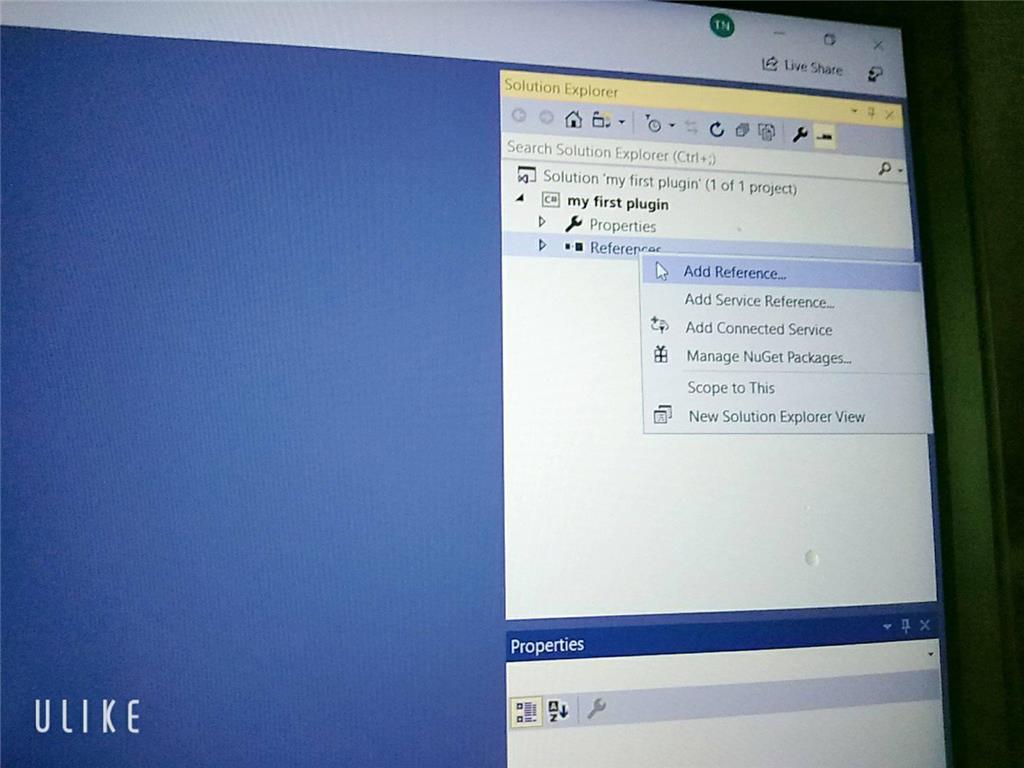
Sorry but can I ask you something why I didn't see the ARC library when I added visual studio even though I set up the C ++. DLL library and there's another way to execute it and send / receive console in out but I don't know how to do it with EZ_builder?Please follow the tutorial. It’s impossible to know why you’re plug-in isn’t showing up without asking you if you followed each step of the tutorial
. Reviewing your screenshots, it doesn’t appear as if any of the tutorial steps have been followed.
Hi i fixed it. thanks
Hello, I am trying the tutorial to get the robot to speak. I am using Visual studio. Currently, the sound is output from the pc instead of the robot. Is there a code I can attach so that the sound comes from the robot speakers instead of the pc?
Look in this tutorial for the step labeled "output audio from ezb". It’s lower down in the list. There’s instruction examples for either playing audio (ie mp3) or text to speech.
Error: the referenced component" EZ_builder,EZ_B" could not be found, DJ Sure i hope you can help me !
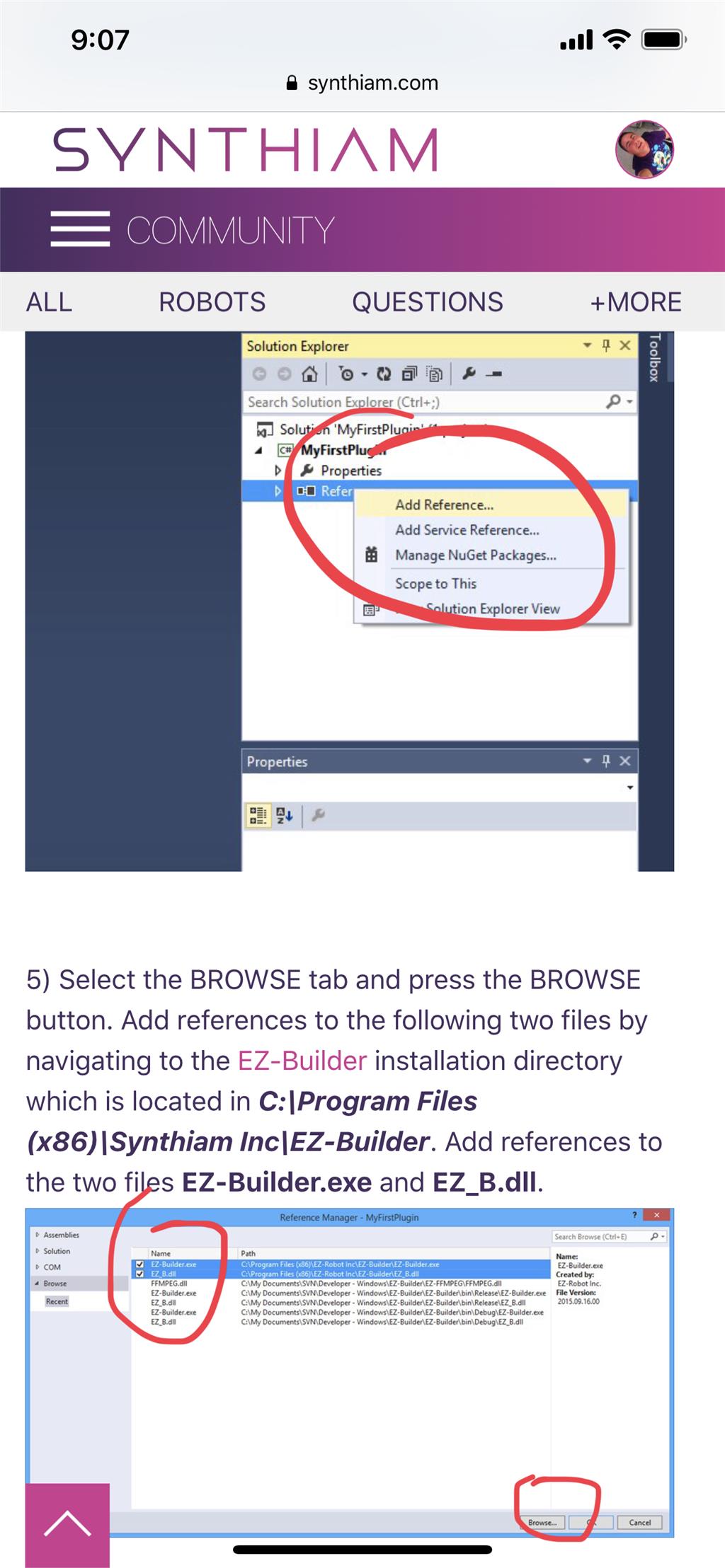
Joinny, you have to add the referencing by following the instructions in this tutorial. They are outlined with step by step to easily follow. Click add references, and browse to the appropriate files as directed in the tutorial. I can’t write anything clearer in response. The step to add references is incredibly clear but you’re skipping it.