Example: Global Script Variables
The ARC variable manager stores and retrieves global variables that are available within ARC plugins and controls. This allows you to Set and Get variables that other controls may be configuring or using.
Data Types The script variable stores dynamic data types. This means that there is no declaration between numeric or string values. For example in JavaScript you would type...
setVar("$a", 3);
setVar("$b", 3.123);
setVar("$c", "This is a string");
setVar("$d", 0x55);
And in C# plugin you would type...
ARC.Scripting.VariableManager.SetVariable("$a", 3);
ARC.Scripting.VariableManager.SetVariable("$b", 3.123);
ARC.Scripting.VariableManager.SetVariable("$c", "This is a string");
ARC.Scripting.VariableManager.SetVariable("$d", 0x055);
Single Variables ARC.Scripting.VariableManager.ClearVariable(string variableName) Clear the variable and remove it from memory.
ARC.Scripting.VariableManager.ClearVariables() Clear the entire variable memory.
ARC.Scripting.VariableManager.DoesVariableExist(string variableName) Returns a Boolean if the variable has been defined in memory.
ARC.Scripting.VariableManager.DumpVariablesToString() Returns a string with each variable and the corresponding value (one per line). This is similar to the Variable Manager control found in EZ-Builder->Add Control->Scripting->Variable Manager.
ARC.Scripting.VariableManager.GetVariable(string variableName) Get the value stored in the specified variable.
ARC.Scripting.VariableManager.GetVariable(string variableName, int index) Get the value stored in the specified index of the array variable.
ARC.Scripting.VariableManager.SetVariable(string variableName, object value) Set the value into the memory location of the specified variable. If the variable does not exist, this will create the variable and assign the value.
Array Variables ARC.Scripting.VariableManager.AppendToVariableArray(string variableName, object value) Append the value to the existing array.
ARC.Scripting.VariableManager.CreateVariableArray(string variableName, byte[] values) ARC.Scripting.VariableManager.CreateVariableArray(string variableName, string[] values) ARC.Scripting.VariableManager.CreateVariableArray(string variableName, object defaultValue, int size) Create an array variable and specify the default values. Use AppendToVariableArray() to add more items to this array.
ARC.Scripting.VariableManager.FillVariableArray(string variableName, object defaultValue) If a variable array already exists, this will fill every defined index of the array with the specified value.
ARC.Scripting.VariableManager.GetArraySize(string variableName) Returns an integer that is the index size of the specified array variable.
ARC.Scripting.VariableManager.IsVariableArray(string variableName) Returns a Boolean if the specified variable is an array.
ARC.Scripting.VariableManager.SetVariable(string variableName, object value, int index) Set the value into the index of the specified array variable. The array size must already be defined. If you attempt to set a value to an index outside of the index size, an exception will be thrown.
Monitoring Variable Changes The variable manager has an event which will be raised for any variable changes. If your plugin has a variable that you wish to watch for changes, subscribe to the event. Remember to unsubscribe from the event when your plugin closes, as per the plugin Compliance section of this tutorial. Below is an example of subscribing, checking for a variable change, and unsubscribing when the plugin closes.
If the variable is an array, the index will be populated with the array index that has changed. If the variable is not an array, the index will equal -1.
private MyForm() {
InitializeComponent();
// Subscribe to variable change event
ARC.Scripting.VariableManager.OnVariableChanged += VariableManager_OnVariableChanged;
}
private void FormMain_FormClosing(object sender, FormClosingEventArgs e) {
// Unsubscribe to variable change event
ARC.Scripting.VariableManager.OnVariableChanged -= VariableManager_OnVariableChanged;
}
private void VariableManager_OnVariableChanged(string variableName, object value, int index) {
if (!variableName.Equals("$myVariable", StringComparison.InvariantCultureIgnoreCase))
return;
// Do something because the variable has changed
}
Is this out of date? There doesn't seem to be a GetConfiguration function within EZ_Builder.Config.Sub.PluginV1
Look at the tutorial step titled [color=#ce3991][size=3][font=OpenSans, -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, "Helvetica Neue", Arial, sans-serif, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol", "Noto Color Emoji"]Code: Saving/Loading Configuration
[color=#ce3991][size=3][font=OpenSans, -apple-system, BlinkMacSystemFont, "Segoe UI", Roboto, "Helvetica Neue", Arial, sans-serif, "Apple Color Emoji", "Segoe UI Emoji", "Segoe UI Symbol", "Noto Color Emoji"]The get and set configuration methods are overrides of the form. There’s a great video on the first step of this tutorial that demonstrates the step by step of building a plugin. I recommend watching that because it helps fill in any steps that were missed. [/font][/size][/color]
[color=#ce3991][size=3][font=OpenSans, -apple-system, BlinkMacSystemFont, Segoe UI, Roboto, Helvetica Neue, Arial, sans-serif, Apple Color Emoji, Segoe UI Emoji, Segoe UI Symbol, Noto Color Emoji]When you’ve done it once, it makes sense and voila, you can rinse and repeat
[/font][/size][/color][/font][/size][/color]
Excellent, thanks - I will do. I really must learn not to just jump ahead in the process
Hey no problem - I do it all the time, and end up frustrated because I dont know what it was that I missed. Excitement gets the best of me
Trying to follow the tutorials but can't find where the plugin page has gone. How do I add a new plugin to the ez-robot / Synthiam site to get the XML?
Never mind. Just found the "Create skill control" link
I am trying to follow the instructions for adding my own plugin but I cannot seem to find the place to register the plugin based on the instructions.
Any help is appreciated.
Thanks
The new button to create a plugin skill control is less than an inch below the button you pressed to create this question.
Thanks for the quick response.
This what happens when you are working on robotics when its way past your bed time.
You miss the obvious
No problem - i get it
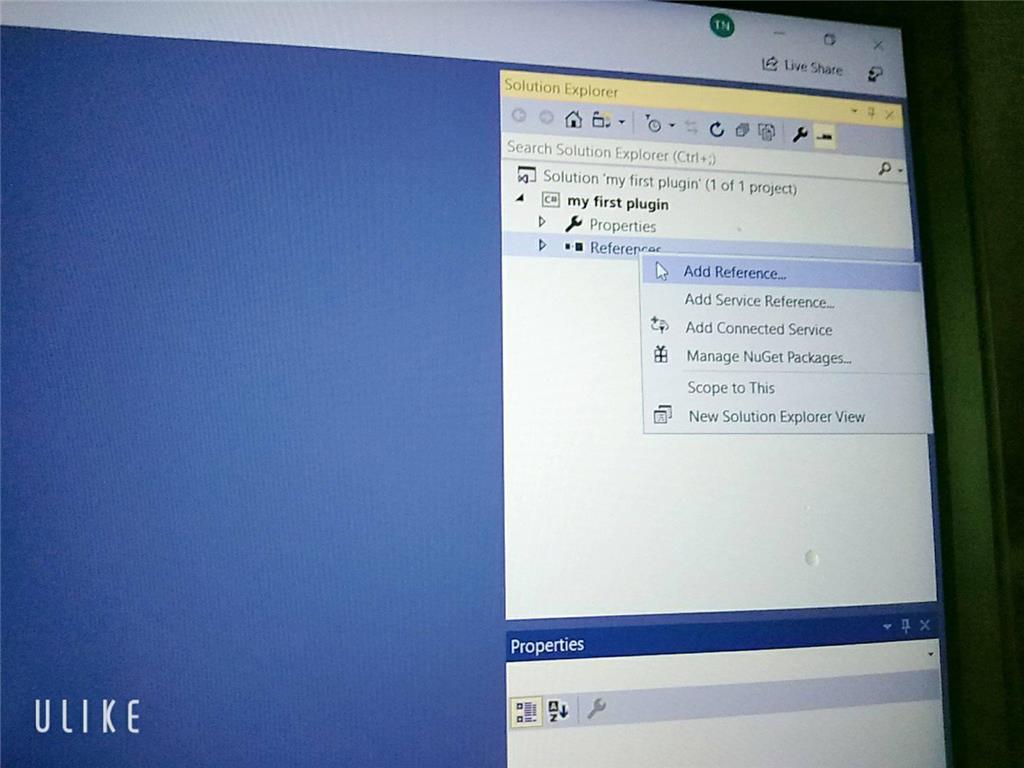
Sorry but can I ask you something why I didn't see the ARC library when I added visual studio even though I set up the C ++. DLL library and there's another way to execute it and send / receive console in out but I don't know how to do it with EZ_builder?Please follow the tutorial. It’s impossible to know why you’re plug-in isn’t showing up without asking you if you followed each step of the tutorial
. Reviewing your screenshots, it doesn’t appear as if any of the tutorial steps have been followed.
Hi i fixed it. thanks
Hello, I am trying the tutorial to get the robot to speak. I am using Visual studio. Currently, the sound is output from the pc instead of the robot. Is there a code I can attach so that the sound comes from the robot speakers instead of the pc?
Look in this tutorial for the step labeled "output audio from ezb". It’s lower down in the list. There’s instruction examples for either playing audio (ie mp3) or text to speech.
Error: the referenced component" EZ_builder,EZ_B" could not be found, DJ Sure i hope you can help me !
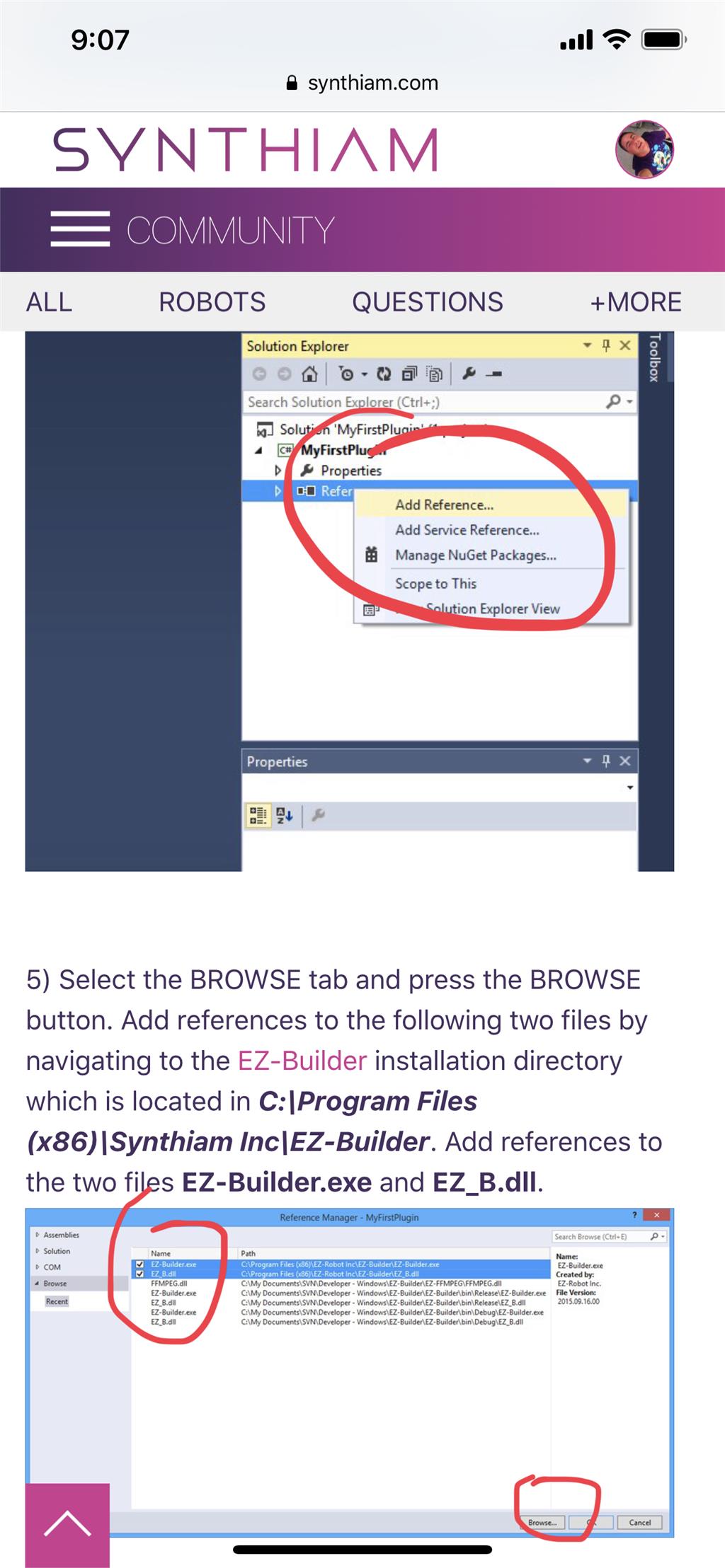
Joinny, you have to add the referencing by following the instructions in this tutorial. They are outlined with step by step to easily follow. Click add references, and browse to the appropriate files as directed in the tutorial. I can’t write anything clearer in response. The step to add references is incredibly clear but you’re skipping it.The error cannot read the COM file, I downloaded it and when I follow the instructions, I get an error, while other files read normally. .
sorry for me but i tried many different ways but still show the error,I couldn't find EZ_B.dll file even though I downloaded it
None of the required references are in your list. Please follow the tutorial. It explains exactly how to click the browse button and navigate to the folder and select the files.
Sorry, but the reason I can't reference is because there is no file in the EZ_B folder and there is an error : this folder is empty , I am trying to solve it. I would like to thank DJ sure for answering my superfluous questions and I'm sorry for bothering you
I need to playback 5 Serial Bus servos in sequence.
What protocol is it? A "serial bus" is a generic term for anything using a UART that's chained together sharing the same RX line. Also, why did you add a photo with the question text added in your response?
Are you planning on making a skill control to do this? You wrote the question in the skill control thread in a comment - I'd like to make sure your question is in the right place to help you out.
To begin, I would recommend starting with servo Script control so you can make the serial bus protocol work - then consider making a skill control only if you're planning on distributing the effort to others: https://synthiam.com/Products/Controls/Scripting/Servo-Script-19068
He seems rather demanding as well. I guess he needs the benefit of the doubt as English may not be his native language....
I have installed all the software dependencies and still ARC does not detect that I have Visual Studio installed. OS is Windows 10, .NET 4.8 or newer, Visual Studio Community 2019.
When the popup says it doesn’t detect visual studio, you can still skip and continue. I wonder why it’s not detecting it? We had a hard time trying to find a proper way of detecting - even Microsoft’s suggestion didn’t actually work eye roll
ill look into it a bit further and see if we can find a better way of detecting
@DJ: It's easy to find the Visual Studio 2017 and up: Microsoft: https://github.com/Microsoft/vswhere/wiki/Find-MSBuild
Some quick c# code to use with .NET: https://github.com/ppedro74/Utils/blob/master/FindVisualStudio/Program.cs
We went this route and it didn’t work on my computer - because I had a preview of visual studio installed which isn’t in that directory path. Microsoft had numerous suggestions of detecting visual studio. The one which worked for our various installations was a registry check.
apparently with the above individual, the registry didn’t work either. I’ll have to combine a few methods.
everything looks simple from the outside - until you have a hundred thousand+ installations of your software. That’s when you run into things like this lol
@DJ: I agree sometimes the things go out of script easily.
I avoid going through the registry keys, unless is recommended by the vendor. A lot of people blame the changes (keys, entries are renamed etc), but, that is normal if I own my product is my business and is part of the software evolution. Some products you can break the support contract agreement if you query directly the database, or if you look elsewhere outside of the public API.
Is true story some years ago a "rogue" developer on my team released a Sharepoint integration using a mix of APIs and database queries, everything worked well with multiple clients, until one day the Microsoft Black suits visit one of the customers to follow up on an unrelated support ticket, and they basically used "unsupported" card and left the client hanging, and we had problems too, unfortunately the Rogue developer went to another galaxy ... and the team suffered the consequences.
That does not mean I'm not tempted to do it...
I used the vswhere before and I would say is almost 99% bulletproof, is used with Xamarin, NVIDIA, Intel setups. If you add vswhere.exe to your project (nuget package) you cover scenarios where the tool is not present or have been deleted (broken uninstalls).
The other fallback could be ask the user the visual studio version.
The other reason to avoid registry is due to Visual Studio uses a private exclusive registry keys to store more stuff: http://www.visualstudioextensibility.com/2017/07/15/about-the-new-privateregistry-bin-file-of-visual-studio-2017/
So the things are getting more complex.
The above post is only part of the "Full solution" for example I have one setup with visual studio 2017 c# installed and Visual studio 2019 with Python and C++, vswhere will return 2019 version, but my c# is done with VS2017.
If you are generating customized vs version project files, maybe a fallback (ask the VS version) will cover more bases.
Yes - Microsoft has a few pages on how to identify visual studio and we tried them all during testing - the one we went with was with registry. I'm going to combine the two as using only one method apparently doesn't work for all cases.