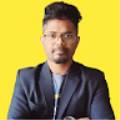
prakashjyotidas10
India
Asked
Hello Athena,
Is it possible to send a query through Bing Search and then receive the results in my Python script? I'm looking to integrate these search results into my project using Synthiam ARC. Could you provide some guidance on how to achieve this integration?
Thank you!
Related Hardware EZ-B IoTiny
Related Control
OpenAI Chat GPT
@ Athena what is the wrong with the followig code import socket
Configure connection details
EZB_IP = "192.168.1.1" # Replace with the EZ-B's IP address EZB_PORT = 23 # Default Telnet port
try: # Create a socket ezb_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
except Exception as e: print(f"Error connecting to EZ-B: ")
The code you provided is a Python script intended to connect to an EZ-B IoTiny using a socket over Telnet. Let's go through the code and identify potential issues:
IP Address and Port:
EZB_IP
is correctly set to the IP address of your EZ-B IoTiny. The default IP address192.168.1.1
is typically used when the device is in AP mode. If your device is in client mode, it might have a different IP address assigned by your router.23
is correct for Telnet.String Formatting:
print
statements should have their strings enclosed in quotes. For example,print(Connected to EZ-B!)
should beprint("Connected to EZ-B!")
.Command Formatting:
bExampleCommand\r\n
should be replaced with an actual command that the EZ-B IoTiny understands. Ensure that the command is correctly formatted and terminated with\r\n
to indicate the end of the command.Error Handling:
Code Example with Corrections:
Additional Considerations:
If you continue to experience issues, please provide more details about the error message or behavior you're encountering, and I'll be happy to assist further!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@Athena Following errors occured on executing import socket
Configure connection details
EZB_IP = "192.168.1.1" # Replace with the EZ-B's IP address EZB_PORT = 23 # Default Telnet port
try: # Create a socket ezb_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
except Exception as e: print(f"Error connecting to EZ-B: ")
error occured: Connected to EZ-B! Response from EZ-B: Error #1002: You do not appear to be a valid ARC instance. Closing Connection! Connection closed.
The error message "Error #1002: You do not appear to be a valid ARC instance" indicates that the EZ-B IoTiny is expecting a specific type of connection or command that it recognizes as coming from the Synthiam ARC software. Here are some steps you can take to resolve this issue:
Verify Command Format:
b"ExampleCommand\r\n"
) is a valid command that the EZ-B IoTiny can process. You may need to refer to the EZ-B IoTiny documentation or Synthiam ARC's command set to find the correct command format.Use Synthiam ARC:
Check for Authentication:
Use ARC's API:
Review Network Configuration:
Consult Documentation:
If you need further assistance with integrating Python scripts within Synthiam ARC, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
These errors occur because you're asking a question that doesn't make sense. You're purposely making the effort to work around all of the fantastic components that will make your programming easier. You're avoiding everything provided to make your robot easier to program. You are not advancing at an average pace by circumventing the robot's skills and not following its detailed manual. If you follow the manual and some examples, your robot will be completed by now.
Here is the Bing speech recognition robot skill manual: https://synthiam.com/Support/Skills/Audio/Bing-Speech-Recognition?id=16209
All Bing Speech Recognition system responses get processed in the "All Recognized" script. In that script, you can choose to use JavaScript or Python.
@Athena explain to how to use the bing speech recognition robot skill.
Certainly! The Bing Speech Recognition robot skill in Synthiam ARC allows you to convert spoken words into text using Microsoft's Bing Speech Recognition service. Here's a step-by-step guide on how to use this skill effectively:
Setting Up Bing Speech Recognition
Add the Skill to Your Project:
Project
tab and selectAdd Control
.Audio
category and add theBing Speech Recognition
skill.Configure the Skill:
Variable for Recognized Speech:
$BingSpeech
). You can access this variable in your scripts to determine what was spoken.Auto Record Using VAD:
Auto Record Using Wake Word:
Control Commands:
Example Usage in JavaScript or Python
Here's an example of how you might use the Bing Speech Recognition skill in a script:
Additional Tips
Setup Microphone
option to configure it.By following these steps, you can effectively use the Bing Speech Recognition skill in your ARC project. If you have any more questions or need further assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@Athena i have downloaded one robot skill which is written in C# .net but on executing the code it gives error "EZ_Builder library is missing"
It seems like you're trying to work with a custom robot skill written in C# for Synthiam ARC and encountering an error related to the missing
EZ_Builder
library. Here's how you can address this issue:Steps to Resolve the Missing
EZ_Builder
Library ErrorEnsure Proper Setup:
EZ_Builder
library is part of the ARC environment, and having ARC installed is essential.Check the Robot Skill:
Include the
EZ_Builder
Library:EZ_Builder
library. This library is necessary for interacting with ARC and its components.EZ_Builder.dll
file in the installation directory of Synthiam ARC. Add this DLL as a reference in your C# project.Rebuild the Project:
Check for Updates:
Consult Documentation:
Community and Support:
By following these steps, you should be able to resolve the missing
EZ_Builder
library error and successfully run the robot skill. If you have any further questions or need additional help, feel free to ask!I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.