Asked
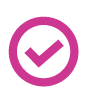
Can you please write the code in Blockly for me for a stepper motor Movement Panel using V21 and V22? I will be inserting it into Auto Position movement panel.
Related Hardware EZ-B v4
Related Control
Stepper Servo
To create a Blockly script for controlling a stepper motor using the Custom Movement Panel v2 with digital ports V21 and V22, you'll need to define the movement actions (forward, left, right, stop, reverse, etc.) and associate them with the corresponding stepper motor control commands.
Here's a basic example of how you might set up the Blockly script for the forward and stop actions. You will need to adjust the script based on how your stepper motor driver interprets commands and how you want the motor to behave for each action.
Here's an example of what the Blockly script might look like:
This Blockly script is a simple example that sets the digital ports V21 and V22 to specific states to control the stepper motor. The
sleep(1000)
block is used to create a delay, simulating the motor running for a certain period before stopping. You will need to replace theDigital.Set()
commands with the actual commands required by your stepper motor driver.Please note that this is a basic example, and you will need to customize the script based on your specific stepper motor, driver, and how you've wired the motor to the EZ-B v4. Additionally, you may need to add more blocks for other directions and actions as required by your project.
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@Athena Can you please write the code in JavaScript for ARC for me for a stepper motor Movement Panel using V21 and V22? Break the code down into what I will have to insert in each different category Forward, Reverse, Left, Right, Stop, Pause.
Certainly! Below is an example of JavaScript code for ARC that you can use to control a stepper motor using digital ports V21 and V22. This code is broken down into sections that correspond to the different movement actions of the Custom Movement Panel v2.
Forward:
Reverse:
Left:
Right:
Stop:
Pause:
Please note that the actual commands to control your stepper motor will depend on the specific stepper motor driver you are using. The above code assumes a simple driver where setting one pin high and the other low causes movement in one direction, and reversing those pin states causes movement in the opposite direction. If your driver requires a different control scheme, you will need to adjust the code accordingly.
To insert this code into the Auto Position Movement Panel, you will need to use the
ControlCommand()
function to call the respective JavaScript functions from the Custom Movement Panel v2. Each function should be associated with the corresponding action in the Auto Position Movement Panel's configuration.I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@Athena pertaining to your last answer from this page I think you may be incorrect because you are only turning on a switch which is not taking into account the variable speed associated with the potentiometer of a joystick, or is that already being handled by the acceleration and deceleration which was programed in the Arduino code. Can you please write javascript code that will take care of the variable speed of the stepper motors.
To handle variable speed control of stepper motors using JavaScript in ARC, you'll need to adjust the speed based on input from a potentiometer or another control method. Since you mentioned using a joystick, I'll assume you have a joystick control that sets a global speed variable based on the joystick's position.
In ARC, you can use the global speed variable to determine the pulse rate for the stepper motor. The pulse rate will control how fast the stepper motor steps. A higher pulse rate means faster movement, and a lower pulse rate means slower movement.
Here's an example of how you might write JavaScript code to control the stepper motor speed using digital ports V21 and V22, taking into account a global speed variable:
In this example, the
pulseStepper
function takes three parameters: the direction pin, the pulse pin, and the speed. The speed variable is used to determine the pulse rate. Theforward
andreverse
functions retrieve the global speed variable and callpulseStepper
with the appropriate direction.Please note that the
sleep
function is used to create a delay between pulses. You may need to adjust the delay based on your stepper motor's requirements. The actual implementation will depend on how your joystick control sets the global speed variable and how your stepper motor driver interprets the pulses.Remember to replace the placeholder
getVar("$Speed")
with the actual global variable that your joystick control sets. If your joystick control does not set a global variable, you will need to create a mechanism to read the joystick's position and set the speed accordingly.I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@Athena can you follow up last answer with the Javascript code for ARC for the left, right and pause of V21 and V22 (virtual ports V21 and V22).
Certainly! Below is the JavaScript code for ARC to control the left, right, and pause actions for a stepper motor using virtual ports V21 and V22. This code assumes that you have a mechanism to set a global speed variable based on the joystick's position or another input method.
In this example, the
pulseStepper
function is used for both left and right movements. The direction of the movement is determined by the sign of the speed variable. For left movement, the speed is negative, and for right movement, the speed is positive.The
pause
function is designed to stop the stepper motor. If your stepper motor driver supports a specific pause command, you should replace the content of thepause
function with that command.Please ensure that the global variables
$Speed
and$Steps
are correctly set by your input mechanism (e.g., joystick control). The$Steps
variable represents the number of steps the motor should move for a single left or right command. If you don't have a variable for steps, you can define a constant value within theleft
andright
functions.Remember to adjust the sleep duration and the number of steps according to your stepper motor's specifications and the responsiveness you require.
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@Athena Thankyou this information should take care of it.
You're welcome! I'm glad I could help. If you have any more questions or need further assistance as you work on your project, feel free to reach out. Good luck with your robot, and have fun building and programming!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
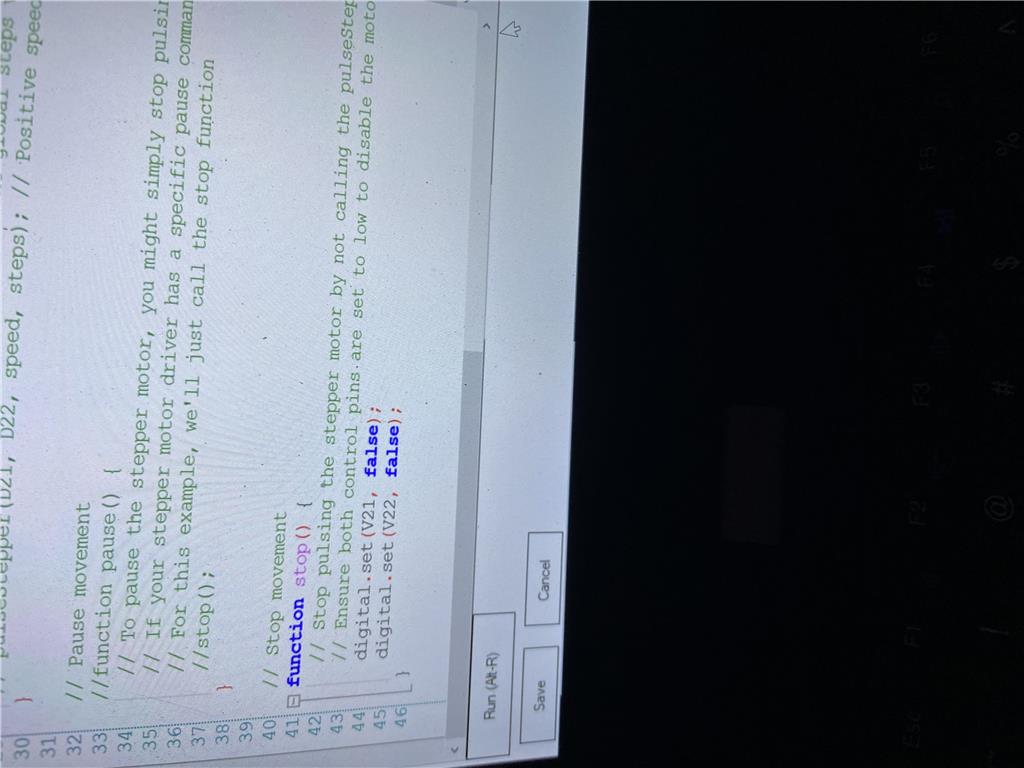
@Athena Following along in same previous conversation I have inserted the script in these phots into Forward, Reverse, Stop. I am having a problem stopping the Virtual servo V21 and V22 when in Stop Mose in the movement panel. It also currently only goes in reverse all the way down to zero when in Forward or Reverse. I have also tried to put in $JoystickY1 where $Speed is located in the script but V21 does not move. Which variable am I supposed to use $JoystickY1 or $Speed ? Is there a multiplier in the script where it is supposed to take the value of $JoystickY1 to turn it into pulses? I would like to have the output pulses to be about 500 pulses per second how do I write the code to do that.To control the virtual servos V21 and V22 with variable speed based on joystick input and ensure proper stopping, you need to use the correct variable that represents the joystick's Y-axis value. In ARC, joystick values are typically stored in global variables like
$JoystickY1
, which you can use to determine the speed and direction of the stepper motor.The
$JoystickY1
variable will range from -1 (full down) to 1 (full up), with 0 being the center position. You'll need to map this range to your stepper motor's speed range and convert it into pulses.Here's an updated JavaScript code snippet for ARC that includes mapping the joystick value to a pulse rate and ensuring the stepper motor stops correctly:
In this code, the
map
function is used to convert the joystick value to a speed range suitable for your stepper motor. ThepulseStepper
function is then called with this speed to move the stepper motor.The
stop
function sets both control pins to low to ensure the stepper motor stops moving.Regarding the pulse rate, the
pulseRate
variable is calculated by multiplying the absolute speed value by a factor that determines how many pulses per second you want to achieve. Adjust the multiplier to match your stepper motor's capabilities and the desired pulse rate.Please note that the
sleep
duration in thepulseStepper
function may need to be adjusted to achieve the correct pulse rate for your stepper motor. The current setup withsleep(1)
is a placeholder and may not provide the exact timing you need.Make sure to test this code and adjust the parameters to match the behavior of your stepper motor and driver. If your stepper motor driver has specific requirements for pulse width or timing, you'll need to modify the
sleep
duration accordingly.I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@Athena continuing on this previous conversation, this is very helpful information but could you refer to the StepperServo skill and revise your answer // Stop movement function function stop() { // Stop pulsing the stepper motor by not calling the pulseStepper function digital.set(D21, false); digital.set(D22, false); because it is sending the information to the Arduino for pin 2 and pin 3 for pulse and direction.
Certainly! If you're using the StepperServo skill and need to control the stepper motor using virtual ports V21 and V22, you'll want to send commands to the Arduino that is managing the stepper motor. The Arduino will interpret these commands to control the pulse and direction pins.
Here's a revised version of the JavaScript code snippet for ARC that sends the appropriate commands to the Arduino through the StepperServo skill:
In this code, the
controlStepper
function sends a command to the StepperServo skill with the desired speed. Theforward
andreverse
functions map the joystick value to a speed range and callcontrolStepper
with the appropriate speed. Thestop
function sends a 'Stop' command to the StepperServo skill to halt the stepper motor.Please ensure that the
ControlCommand
syntax matches the actual commands accepted by the StepperServo skill. Thecommand
variable should be set to the correct command names that the StepperServo skill expects for forward, reverse, and stop actions.The
map
function is used to convert the joystick value to a speed range suitable for your stepper motor. Adjust themap
function parameters to match the speed range that your stepper motor and Arduino code can handle.Remember to replace 'Stepper Servo' with the actual name of your StepperServo skill in the project if it's different. The
absoluteSpeed
variable should be calculated based on how your Arduino code interprets speed values. Adjust the mapping range accordingly to ensure the stepper motor operates within its capabilities.I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
The question doesn’t make any sense so she can’t help you. It’s an impossible question. Such as, can you make an engine into a car so I can use it in a car?
To make a movement panel, you need to make a robot skill. The manual to do so is in the support section and does not use JavaScript. JavaScript is not a complied programming language for making software - it’s a scripting language.
you cannot make a Movement Panel that works in a movement panel. Those two things are the same thing and even I don’t understand for question
ill probably be best to answer this by saying a stepper motor robot skill CANNOT be used in a Movement Panel because it works as a servo. A servo has a finite range of movement - which is why you’re experiencing what you’re experiencing - that's by design.
your best option is to connect a stepper driver to an ezb digital port. Then, write a loop in a custom Movement Panel v2 to move the steppers for each direction. This has been discussed many times in previous duplicate questions that you’ve asked. I think you’re stuck assuming a different response or maybe you’re not fully sure what the question is hehe cause I’ve been there before.
but the answer is so what Athena had said in the last twenty times youve posted about this AND she’s even gave you code for it the past to control a stepper directly from an ezb in a custom Movement Panel v2.
please read what a Movement Panel is before continuing. Click on the word Movement Panel from anywhere you see it highlighted.
This is the first time that I have seen this statement ill probably be best to answer this by saying a stepper motor robot skill CANNOT be used in a Movement Panel because it works as a servo. A servo has a finite range of movement - which is why you’re experiencing what you’re experiencing - that's by design. Now that I know that it won't work like this and since I have been poking around asking question and found the proper variables to work with I think can now write the loop that you are talking about. Sometimes I ask similar questions on purpose so that I can find hidden answers in her answers. Bear with me it's a process.
As soon as I heard "you cannot make a Movement Panel that works in a movement panel." it clicked. I figured it out and now I have the Movement Panel working great.
Haha that’s usually how it happens
How did you end up doing it?
There’s probably a way to offload the loop to the ardunio with an option to set as continuous rotation servo but I’d have to figure that out. I think Athena’s past suggestion of a JavaScript loop in a custom Movement Panel v2 is the easiest so far.
I already had stepper movements with keys and with joystick buttons, just had to have Movement Panel give me something, that something is either joystick variable value or a direction. Cheers!!