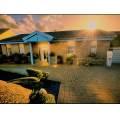
PRO
jp15sil24
Germany
Asked
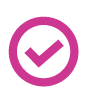
How To Make A Camera Chases A Ball
I am using a servo and movement tracking with the Roli Rover to chase a ball. It works quite well, but if the ball moves outside the camera's view range, Roli stops. I would like the camera to move and try to locate the ball when it goes out of view. How can I achieve this?
Related Hardware Roli Rover
To make your Roli Rover's camera chase a ball and continue searching for it when it goes out of view, you can use the Camera Click servo and Face Tracking skills in combination with some scripting in ARC. Here's a step-by-step guide to achieve this:
Add and Configure Camera Click Servo:
Add and Configure Face Tracking:
Create a Script to Move the Camera:
Integrate the Skills with Scripting:
ControlCommand()
function to integrate the Camera Click servo and Face Tracking skills. You can use JavaScript or Python for scripting in ARC.Here is an example script in JavaScript to move the camera and search for the ball:
Steps to Implement the Script:
Add the Script to Your Project:
Project
tab and click onAdd Control
.Scripting
and add aJavaScript
control.Configure the Camera Click Servo:
Configure the Face Tracking:
Run the Script:
Start
button in the JavaScript control.This script will continuously check if the ball is detected. If the ball is not detected, it will move the camera in a predefined search pattern to locate the ball. Once the ball is detected, it will move the robot towards the ball.
Feel free to adjust the search pattern and timings to better suit your specific setup and environment. If you have any further questions or need additional assistance, please let me know!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
thanks Athena. where can I find the face tracking skill ?
I think @Athena's suggestion is somewhat correct. However, her instructions may not have been very clear about where a script like this could execute.
The idea is to run a script when the "tracking stops" to search for the object. This is found in the camera device configuration screen under the "scripts & variables" tab. You will find a script to execute when "tracking ends." See this screenshot.
Next, you can have the camera servo move to find the ball. The script in the "tracking end" would only need to run for a finite number of seconds. For example, searching for the ball for 2 seconds would be fine, but you could adjust it to your preference.
The camera would need to move to each specified coordinate in an array and pause between movements to find the object.
The "tracking end" script will automatically be stopped by the camera device when the "tracking start" script begins, so you will not need to stop the script if an object is found. The tracking end script merely needs to move the camera around, looking for the object. Because the object will be left or right, moving the camera up or down is unnecessary.
hi, i copied the javascript but it can not find the variable $FaceTrackingDetected. Into the camera skill , i have the tracking option with tracking types. i choose " face " but the skill searches for a face, i can not fool it with an object or color(ball).I tried to replace the variable $FaceTrackingDetected with ObjectTrackingDetected but this variable does not exist. In the intructions provided by Athena, it requests to have the skill "Face Tracking skill "installed but i can not find this skill. in the "tracking end", i copied the script and clic on Run, it brings the error "variable not defined :$FaceTrackingDetected. I check the camera click servo, i setup the port d9 ,d10, and setup exactly the same values min,max for each servo. I got another error message: controlcommand Errorfor Camera click servo sending SetPosition , controlcommandwith parameter SetPosition90,90 is not supported for this control ( camera click servo)
Maybe i just have to install the Face Tracking Skill but i dont know where it is. thx jp
hi jp15sil24
always use the @ for the name @athena els che wil not replay .
i'm just gessing for face tracking skill , is that not a script you need ?
this might be handy . https://synthiam.com/Support/Skills/Scripting/Script-Collection?id=16045
It seems there has been some confusion regarding the Face Tracking skill and the variables used for tracking. Let's clarify and provide a step-by-step guide to achieve your goal of having the camera move to locate the ball when it goes out of view.
Step 1: Add the Camera Device Skill
Project
tab at the top of the ARC window, then click on theAdd Control
button.Add Control
window, typeCamera Device
in the search bar.Camera Device
skill from the search results to add it to your project.Step 2: Configure the Camera Device for Object Tracking
Tracking
tab, selectColor
orObject
tracking, depending on what you want to track (e.g., the color of the ball).Step 3: Add the Camera Click servo Skill
Project
tab, then click on theAdd Control
button.Add Control
window, typeCamera Click Servo
in the search bar.Camera Click Servo
skill from the search results to add it to your project.Step 4: Add the Script for Tracking End
Scripts
tab.Tracking End
script section, add the following JavaScript code:Step 5: Test the Setup
Troubleshooting
$ObjectTrackingDetected
.Camera Click Servo
skill is correctly configured and supports theSetPosition
command.If you still encounter issues, please provide specific error messages or behavior details, and I'll be happy to assist further.
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@athena
Do not use the camera click skill or face tracking. All you need is the camera device robot skill. Add a script to the tracking end, and have the script search for a ball. It only needs to move the servo left and right horizontally. Also, use the servo set position script commands to move the servo.
Thank you for the clarification, DJ_Sures. Let's simplify the process by using only the Camera Device skill and a script to move the camera horizontally to search for the ball. Here's how you can achieve this:
Step 1: Add the Camera Device Skill
Project
tab at the top of the ARC window, then click on theAdd Control
button.Camera Device
in the search bar.Camera Device
skill from the search results to add it to your project.Step 2: Configure the Camera Device for Object Tracking
Tracking
tab, selectColor
orObject
tracking, depending on what you want to track (e.g., the color of the ball).Step 3: Add the Script for Tracking End
Scripts
tab.Tracking End
script section, add the following JavaScript code:Step 4: Test the Setup
Troubleshooting
$ObjectTrackingDetected
.By following these steps, your Roli Rover should be able to move the camera to search for the ball when it goes out of view. If you encounter any issues, please provide error messages or behavior details, and I'll be happy to assist further.
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
i got the error message by running the script that the ControlCommand with parameter SetPosition 45,90 is not supported .I attach some photos, it may help for solving my problem..
@Athena @DJ_Sures . Many thanks, it is working now. I just added more positions for the variable "var". 45-90-135 was too fast. Now the scan object is smoother.
Excellent! Glad to hear DJ was helpful to get this working for you
Great to hear. I have an idea for the script addition. It current runs forever until the object is detected. Maybe it only needs to run for 5 seconds or something.
@athena can you alter the script so it runs for 5 seconds? Also add more 11 positions to the array.
Sure, DJ! Let's enhance the script to run for 5 seconds and add more positions to the array for a smoother scan. Here's the updated script:
Explanation:
duration = 5000
milliseconds).$ObjectTrackingDetected == 1
) and exits the function if the object is found.How to Use:
This should make the camera's search for the ball smoother and more efficient. If you encounter any issues, please let me know!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@athena i removed following text otherwise we got an error with a variable not found: if (getVar("$ObjectTrackingDetected") == 1) { return; // Exit the function if the object is detected } . i will play a little bit with tilt servo just for fun .thx again
I'm glad to hear you're making progress! If you encountered an error with the variable not found, it might be because the
$ObjectTrackingDetected
variable wasn't set up in your project. However, removing that part is fine if it works for you.If you want to add some fun with the tilt servo, you can modify the script to include both pan and tilt movements. Here's an updated version that includes tilt movements:
Explanation:
How to Use:
This should add some fun by making the camera move in both pan and tilt directions while searching for the ball. If you have any more questions or need further assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.