
PRO
afcorson
Australia
Asked
— Edited
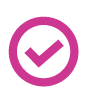
@Athena,
How can I use a variable in the WaitForSpeech
command? For example, I want to use $Exhibit = WaitForSpeech(30, $ExhibitList)
, where $ExhibitList
contains several options. How can I achieve this?
Related Hardware EZ-B v4
The manual for Audio.WaitForSpeech has an example: https://synthiam.com/Support/javascript-api/Audio/waitForSpeech
@Athena Sorry I haven't made myself clear. The list of values is stored in a text file. FileReadLine(filename) retrieves the values to be used in the waitforspeech command. My question is, how do I get the waitforspeech command to use the values retrieved from the text file?
@athena answer the question please.
To use values retrieved from a text file in the
WaitForSpeech
command, you can follow these steps:File.readLine()
function to read the values from the text file.WaitForSpeech
command: Pass the variable containing the values to theWaitForSpeech
command.Here's an example of how you can achieve this in JavaScript:
In this example:
File.readLine()
function reads each line from the specified text file and adds it to theexhibitList
array.Audio.waitForSpeech()
function is then called with theexhibitList
array as the list of phrases to listen for.Audio.waitForSpeech()
function is printed to the console.Make sure to replace
"path_to_your_text_file.txt"
with the actual path to your text file.If you have any further questions or need additional assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
This works thankyou. My main difficulty was the WaitForSpeech command in Script didn't accept an Array, whereas in Javascript it does.