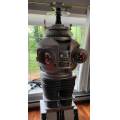
PRO
TerryBau
USA
Asked
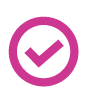
How to write python on pyCharm to connect to EZM using socket and then run a command, voice, script etc.?
I do not get any errors, just nothing happens lol
import socket
Define ARC system's IP address and port
host = '192.168.1.22' # Replace with the ARC system's IP address port = 23 # Replace with the ARC system's port (usually 23)
try: # Create a socket connection arc_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) arc_socket.connect((host, port))
# Specify the path to the ARC script
arc_script_path = "C:\\Users\\your_username\\Documents\\ARC\\My Projects\\test.ezb" # Replace with the correct path
# Send the ARC script execution command
arc_script = f"RunScript({arc_script_path})"
arc_socket.send(arc_script.encode())
# You may not receive a response immediately, but you can add a delay or loop to check for a response if needed
# Close the socket connection
arc_socket.close()
except socket.error as e: print(f"Socket error: ") except Exception as e: print(f"An error occurred: ")
Related Hardware EZ-B v3
I’m sorry but I can’t quite understand what you’re asking. Can you provide information as to what it is you’re trying to do?
Thanks DJ Sures... i use PyCharm to create applications, for there you can do lots of importing of packages and call APIs... but on the ARC side you can't (unless I don't know how to import new modules) so I was wondering if I can run my Python script from PyCharm and have it connect to my EZ Robot and run scripts it finds there, this way I can have both systems talk to each other...
You have a python program for a robot that uses some framework for interacting with hardware. You wish to integrate that existing python program with ARC.
Because I do not know what your program is or what framework you're using in python, I cannot guess on how to integrate the two. What I can recommend is persueing one of these two options...
Convert your existing python program into a library and add it to arc. The My Documents\ARC\Python Modules folder contains user added python scripts. Documented here: https://synthiam.com/Support/python-api/python-overview
Enable the connection control's TCP server and interact with hardware via that. https://synthiam.com/Support/Skills/Communication/EZ-B-Connection?id=16041
There's a few other robot skills that allow connecting to third party stuff for servos and camera. Specifically the unity robot skill. You can look into those as well.
thanks, i will check that out
If you enable the TCP Server for connection #0 in the connection control robot skill, you can run this in Python...
PS, this will also run in an ARC python script control because ARC runs Python within itself.
This Python code establishes a network connection to ARC TCP Server, sends a command, receives a response, and then closes the connection. Here's a breakdown of what the code does step by step:
Import the
socket
module: This code begins by importing the Pythonsocket
module, which provides functions and classes for working with network sockets.Define server IP, server port, and command: The IP address of the server you want to connect to is defined as '127.0.0.1' (localhost), and the server's port number is defined as 6666. Additionally, a command string is defined as "SayEZB("I am connected")\n". This EZ-Script command is intended to be sent to the ARC Telnet Server and it will be executed. It will speak out of the connected EZB with the phrase.
Create a socket: A socket is created using
socket.socket(socket.AF_INET, socket.SOCK_STREAM)
. This line initializes a TCP socket for communication with the specified IP address and port.Try to establish a connection to the server:
sock.connect((server_ip, server_port))
: This line attempts to establish a connection to the server using the IP address and port specified earlier.Receive and print the server's version:
version
is initialized as an empty bytes object.version
variable.\n
) in the received data. If found, it breaks out of the loop.Send a command to the server:
sock.sendall(command.encode())
: This line sends the command to the server after encoding it to bytes. This is necessary because network communication typically works with bytes, not strings.Receive and print the server's response:
response
is initialized as an empty bytes object.Close the socket: The code uses a
finally
block to ensure that the socket is closed, regardless of whether the operations succeed or fail. This is good practice to release resources properly.In summary, this code establishes a connection to the ARC TCP Server, sends an EZ-Script command, receives a response, and closes the connection. It's a basic example of performing client-server TCP Server communication using sockets in Python to Synthiam ARC.
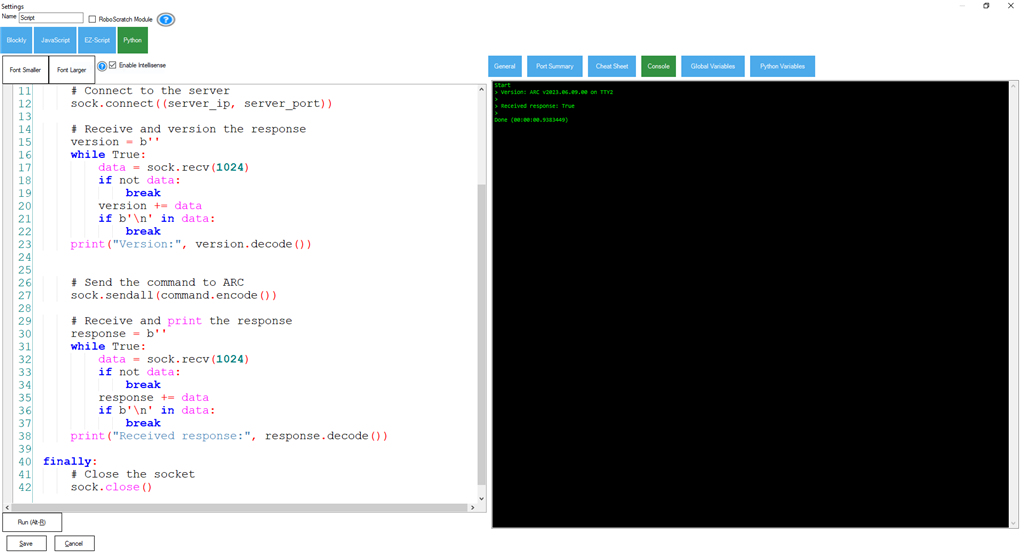
dLastly, you can test the TCP Server by simply telneting to 127.0.0.1 port 6666 and typing commands with the keyboard. This will familiarize you with the syntax. All TCP Server commands are responded with a "True" or their console output. So moving a servo Servo(D0, 2) will result in a response of True. But, sending Print("Hello") will result in "Hello."
PS, there's a python module that you can use in your existing python program that connects to ARC via the tcp server. Find it here..
https://synthiam.com/Support/python-api/add-custom-python-modules
Name this ARCClient.py
Here's how you can use it...
PS, the TCP server uses ezscript so the manual for the commands is here: https://synthiam.com/Support/ez-script-api/ezscript
And if you want to run a script, simply call the ControlCommand() method in the ARCClient python code for the script that you want to run. Replace the Say("") line with the ControlCommand()
that is amazing and it all worked like a charm esp the sample you sent... when i sway it out with a command = "controlCommand('Scripts', 'ScriptStart', 'Date')" it either says ControlCommand not defined or it just sits with a terminal prompt...