
stevehaze
United Kingdom
Asked
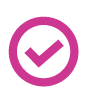
Hi,
I've been using the following tutorial and code to get data from an Arduino for a battery of sensors...
One of my sensors is a Barometer and is sending the pressure....but the value falls outside of the 255 that this tutorial seems to accept. The number for the pressure on my arduino is a float of currently 998.10 - but I don't mind losing the decimal and just having an integer.
Could one of you kind folks give me some advice on what script / command I can use to read this number?
Thanks
Steve Haze
Related Hardware EZ-B v4
Related Control
Script
A byte is 8 bits which has a maximum value of 255 (256 possible variations of the bits)
a float, or an int, or a decimal, or a short, etc... all consist of many bytes.
so an int16 is 2 bytes
an int32 is 4 bytes
a long (int64) is 8 bytes
the bytes are assembled together using bitwise operators to recreate the original intended value.
I don’t know what’s compatible with a float between windows an Arduino, since floats are considerably platform dependent. An int16, however, is easy
you’ll need to send your in on the Arduino as two bytes
and receive it on the ezb or what ever the receiver is, as two bytes
Here's JavaScript code from ARC that demonstrates how to receive and convert 2 bytes from the hardware UART into an INT..
(i don't use ezscript anymore
)
First, initialize your hardware UART...
Now, send your data from the Arduino...
I wrote this to receive 2 byte data ( this example for voltage) from the roomba (and charging state...single byte).... It kind of worked but strangely just for voltage it wigged out on any other 2 byte data request... maybe it will help you out a bit...
Richard, the reason it would wig out is because the uartread() returns a string. In ezscript, you’d have to use uartreadbinary().
but, JavaScript is more powerful and faster
oh, the new roomba plugin I just finished reads sensor data. And has variable turning and such. It’s real great. I’ll publish it this week with the new arc.
@DJ.... Fair enough. I need to revisit that. FYI I am in the process of learning JavaScript for ARC as I can see the benefits over ezscript.... A quick JavaScript (with the plugin) for the roomba sensors would be most welcome... I like learning from example code....
Cheers
Thanks DJ and Richard.....love the quick replies here.
Time to buy JavaScript for Dummies then?
Assuming I do switch to JavaScript....Is there a similar ControlCommand function in JavaScript to control the other plugins?
Hmmm....
So with that code.... (DJ's code)...sending the number 1021....the code gives me the value 65021 at the end.
scratches head confused
Oh, I might have confused the byte order. Either on the sender or receiver, change the order of the bytes
so like, on the sender. Change the order to be...
Serial.write(value & 0xFF00 >> 8); Serial.write(value & 0x00FF);