
stevehaze
United Kingdom
Asked
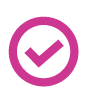
Hi,
I've been using the following tutorial and code to get data from an Arduino for a battery of sensors...
One of my sensors is a Barometer and is sending the pressure....but the value falls outside of the 255 that this tutorial seems to accept. The number for the pressure on my arduino is a float of currently 998.10 - but I don't mind losing the decimal and just having an integer.
Could one of you kind folks give me some advice on what script / command I can use to read this number?
Thanks
Steve Haze
Related Hardware EZ-B v4
Related Control
Script
Hmmm.....that didn't still didn't seem to work...
SO.... I did some googling and learning and this is what worked for me for sending the Float...SORT OF..... I'm cheating a bit....but for the 1 decimal place that I need for this sensor reading, I'm happy.
(PS: I know the Ezb could poll this particular sensor itself...but my arduino is handling all my sensors and then sending to Ezb.)
On the Arduino...
Then in ARC....JavaScript (modified from what DJ Sures posted)....
Thank you so much DJ for your ALWAYS quick replies and sharing of knowledge.
Oh - you were still trying to send a float? The float wouldn't work in my example, as it was only an in16. Your example would work, but you do lose one digit of resolution. It, the maximum range of a signed Int16 is -32,768 to 32,767. So with your solution, the maximum rage would be -2,768 to 2,767
If that range is acceptable for your application, it's golden
. Otherwise, use a signed Int 32 (4 bytes)
I tried that...but I couldn't find or figure out the decoding of the 4 byte array... I had the arduino variable that's being sent as a Long and the following...
I'm only just learning about shifting... So I'm guessing after receiving 4 bytes and not 2....the Javascript line here would need to be different.....but I'm not sure how. Can you advise?
4 byte (int32) would look like this...
On the Arduino...
Then in ARC....JavaScript (modified from what DJ Sures posted)....
Excellent....
I'm getting 'int32' was not declared in this scope but if I use LONG then it works.
Namaste!
Oh ya! Arduino is int_32 I think or long. Thanks for correcting my codez!