Hey guys...this Adafruit 16-channel PWM/Servo Shield integrates seamlessly to ARC now...
So if you are having one sitting on the shelf somewhere, give it a try and use some ARC functionality to drive your servos!!
By Mickey666Maus
— Last update
Other robots from Synthiam community
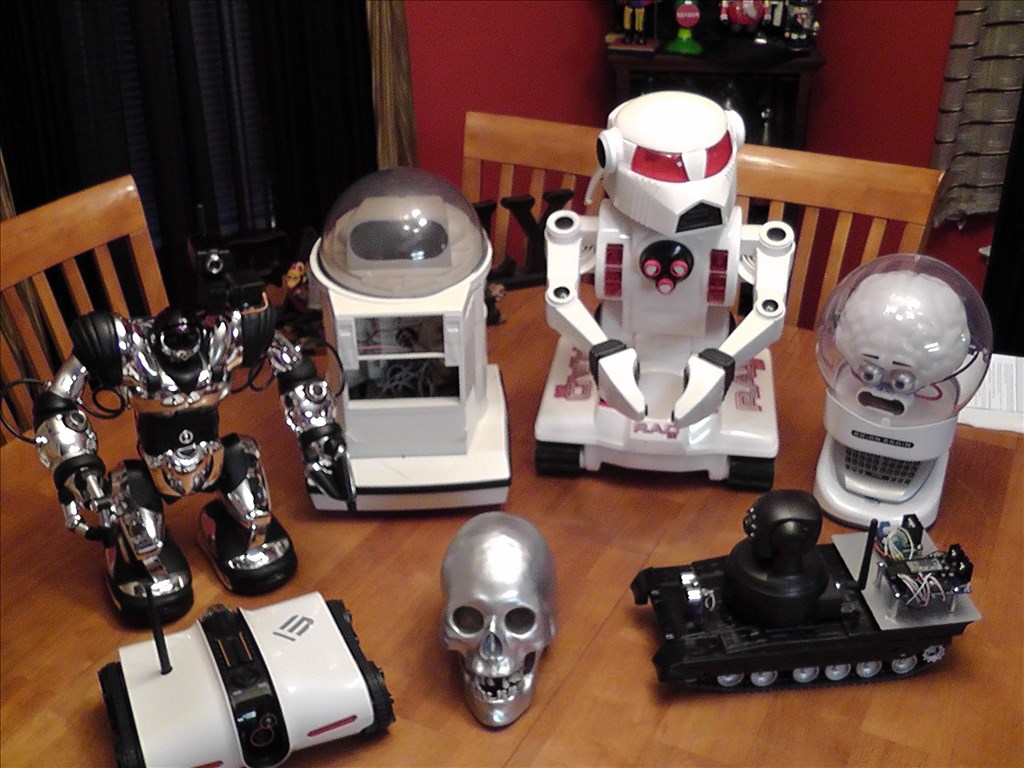
Joseph4760's Project X-Men
Project X-Men Project X-Men Over the last few months I have accumulated several EZ-Robot candidates. Two of the robots...
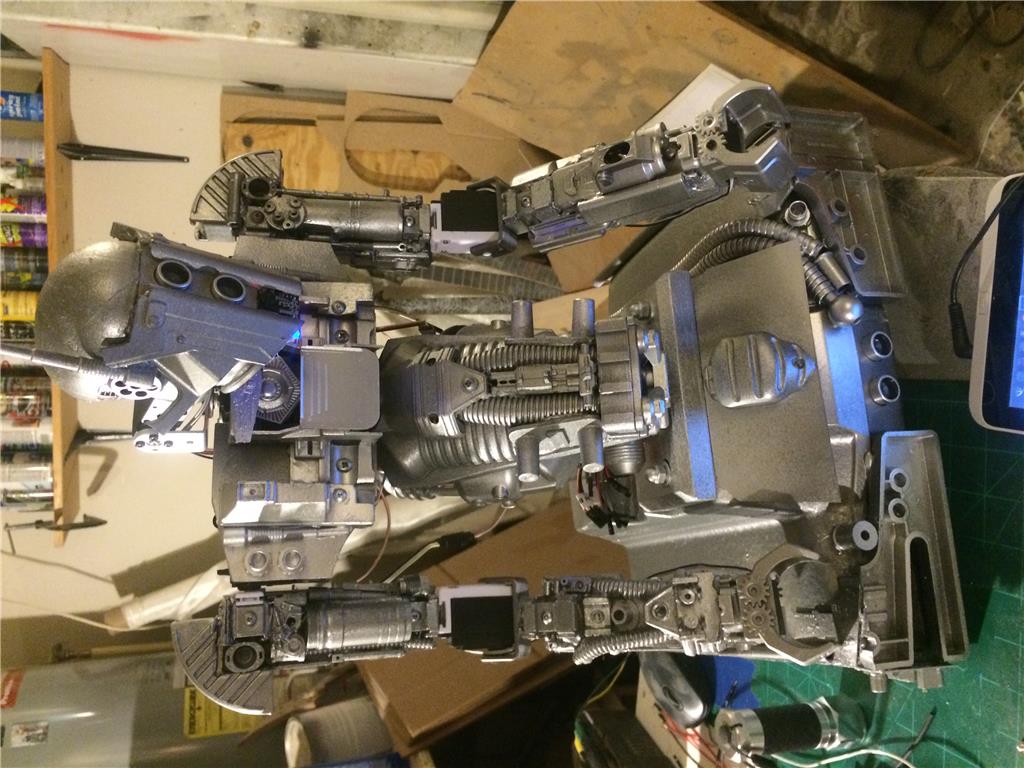
Doombot's Archetype Finally Complete
Well its never really complete right? Heres my first (whew!) fully working droid, Archetype. As you can see its somewhat...
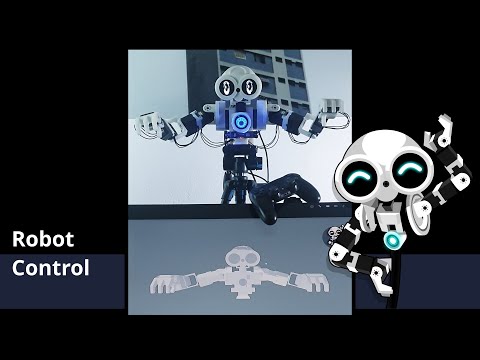
Mickey666maus's UNITY LINK / ARC
This is aimed towards anyone interested in how to link a 3D environment to the ARC software package... This example...
Awesome! I added your firmware with a few minor tweaks here: https://synthiam.com/Hardware/Hardware/Adafruit-16-Servo-Shield-17752
Someday soon you'll be able to edit and add your own firmwares... we're working on it
Sweet!! I am really happy with the progress!!
One thing where I am a little stuck is, since I connected my 16-Channel servo Driver (which basically has the same pinout and functionality than the shield) via I2C to my Raspberry Pi...how can I control the board by connecting to my EZBPi? It would be great if this could be possible!!
This is the board connected to my Raspberry Pi via I2C https://learn.adafruit.com/16-channel-pwm-servo-driver?view=all
I wonder if the Alamode would work if you needed a go between? I know that it interfaces between the world of Arduino and raspberry pi but I haven't looked at it in depth to see how it works. I believe it uses an I2C bootloader interfaced to the Pi. My thought was that you might be able to use the Arduino shield you posted at the beginning of this thread on top of the Alamode. Alternatively, you might be able to hook up an Arduino + above shield to the Pi USB and use serial communication functionality as well. Just ideas, not really a well thought out solution. Link to the Alamode at Seeed Studios
@Jeremie Thanks for the feedback!!
But unfortunately, I don't think this is an option! I am using the board for the reason that I already connected a shield to my Raspberry Pi...so I was hoping for a solution which allows me to connect straight out of ARC to the EZBPi and have it taking care of the connection the same way my Raspberry Pi is doing it. (I2C)
But if this is not possible for now, I can still use the EZBPi functionality to drive Serial Bus Servos which is also fun!!
The arduino connects to the raspberry pi via usb and becomes a serial port
you connect to that serial port in ARC.
@DJSures I was trying to do it this way but had no luck connecting while running ARC on my Raspberry Pi! But it could have been that I chose the wrong COM port or another PEBCAK...
I will try again later when I a back home!!
My Raspberry Pi is connecting to the Server Board using its I2C ports... If I have to use a Raspberry Pi as a bridge, I would need to change the wiring everytime I am changing setups... eg my Raspi only solution and the EZBPi solution.
Its not a big deal, I will just solder the I2C with open endings to the servo Board, and plug them either to the Arduino or to the Raspi... I was just hoping it could be done by the EZBPi talk to the servo Board directly!!
I am aware of the situation that I am probably the only guy here using this setup, so don't worry, I can plug and unplug a cable every now and then. It's great that I can run my robot on ARC now without too much re-wiring!!
I'm 100% confident that the reason you can’t get it to work is not connecting to the right serial port - and most likely the incorrect baud rate as well.
Both of those settings need to be selected that match the Arduino.