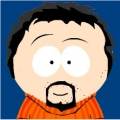
fredebec
France
Asked
— Edited
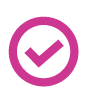
Hi,
I try to convert an old EZ scripts into a python scripts It is a script that reads a number from a txt file, makes a calculation, then rewrite the file with the new value.
The following EZ scripts works fine:
# Close the text file to make it accessible
FileReadClose("C:\Users\FredWin\Documents\ARC\Scripts\Calc.txt")
# Obtain a number from the text files and use it as variable
$calc = FileReadAll("C:\Users\FredWin\Documents\ARC\Scripts\Calc.txt")
# Show the number in the debug window<br />Print("old value:")
Print($calc)
# Calculation
$calc = $calc + 1
Print("---")
Print("new value:")
Print($calc)
FileDelete("C:\Users\FredWin\Documents\ARC\Scripts\Calc.txt")
FileWrite("C:\Users\FredWin\Documents\ARC\Scripts\Calc.txt", $calc)
But the following python script does not:
# Close the text file to make it accessible
File.ReadClose("C:\Users\FredWin\Documents\ARC\Scripts\Calc2.txt")
# Obtain a number from the text files and use it as variable
setVar("$calc2", File.ReadAllText("C:\Users\FredWin\Documents\ARC\Scripts\Calc2.txt") )
calc2 = (getVar( "$calc2" ))
# Show the number in the debug window
print("old value")
print(calc2, type(calc2))
# Calculation
calc2 = ( calc2 + 1)
print("---")
print("new value")
print(calc2, type(calc2))
File.Delete("C:\Users\FredWin\Documents\ARC\Scripts\Calc2.txt")
File.Append("C:\Users\FredWin\Documents\ARC\Scripts\Calc2.txt", calc2)
The problem seems to be with the "File.Append" function. Instead of putting a number for the new calc2 value in the txt file, it writes a special character. So after that, calc2 is considered as a string, and the scripts cannot make the calculation anymore.
Any idea ? Thanks
Related Hardware EZ-B v4
Related Control
Script
Take a look at this: https://www.freecodecamp.org/news/python-string-to-int-how-to-convert-a-string-to-an-integer-in-python/
str(calc)
Hey there, I hope I understood correctly what you are trying to achieve...this code should work!
Short mode:
@Fredbec and others: You are mixing different environments, so is important to understand each and the limitations.
@DJ: There is a bug
Next I'll breakdown in multiple posts.
Python World: There is Python 2 and Python 3, and they are not the same there are significant changes, Python 2 is deprecated.
ARC Python support is build on top of IronPython https://ironpython.net/ I've crossed paths a few years ago and we gave up due to lack of support, IronPython is on life support. Microsoft gave up, the main architect left to google and is being supported by volunteers.
IronPython is (Python 2).
The reason why Python is growing is due to the use on scientific, robotic and ITOps areas, also there are a lot of good libraries to do impressive stuff scipy https://www.scipy.org/ is one of them.
There is another player CPython: https://en.wikipedia.org/wiki/CPython, allows calling native methods from C, is used also to compile Python to C. That is the reason why some ROS scripts work well in embedded computers, the Python libraries are calling native code (c++).
When Microsoft released the IronPython, the community look to integrate different libraries with IronPython, but, there is a problem IronPython works on top of .NET so is managed not native code.
Anything with components written in C (for example NumPy, which is a component of SciPy) will not work on IronPython as the external language interface works differently. Any C language component will probably not work unless it has been explicitly ported to work with IronPython.
So basically all the python super powerful libraries are not available to IronPython.
Is important to understand there are a few different types in Python: bytes, bytearray, list and tuples, they look the same but they are different.
Python2 script:
Python 2 execution:
ARC (IronPython):
One of differences between Python 2 and Python 3 is the bytes type.
In Pyton2 bytes type is an alias to str type.
But that is not true in IronPython, bytes is not an alias. There are other differences between Python 2 and IronPython, and sometimes they can be relevant when using Python 2 code.
To summarize: Use Python 2 code Some Python 2 code may not work as expected on top of IronPython (ARC)
EZ-Script: EZ-Script has a few of limitations, and is easy to understand why DJ is pushing and motivating people to move to Javascript (Interpreted), Python2 (.NET Managed = .NET C# code).
One of the issues with EZ-Script is the lack of data types, initially everything was handled as string, and converted on demand (when needed) to integers (32 bits), later DJ changed the conversion to big ints (64 bits).
So basically when you set a variable in ARC, there is no type, so if you send a typed value from Javascript, Pyhon, plugin (C#) to EZ-Script and you try to get the value back you will lose the original type.
One example:
So if you send a string to ARC, and if that is string is similar to number ARC will return a float. So is important to understand you need to convert back to the original type and or validate if the value is not changed in between your calls. In my example I sent a string "10" and i got back a float, the script will fail if i use the value to concat with another string.
To summarize: Keep your calculations in one side (Python), and if you exchange values with EZ-Script pay attention to the types, don't assume the value data type is the same, validate and use conversion functions: str(x), int(x), float(x)
@DJ: There is a bug you can't call:
from python. I believe your implementation does not take in consideration the different Python types: bytes, bytearray, list, tuple
Python script:
None of the "array types" will work with File.Append
I did a small implementation here: https://github.com/ppedro74/Utils/blob/master/IronPythonTests/IronPythonTests.Common/PythonEngine.cs
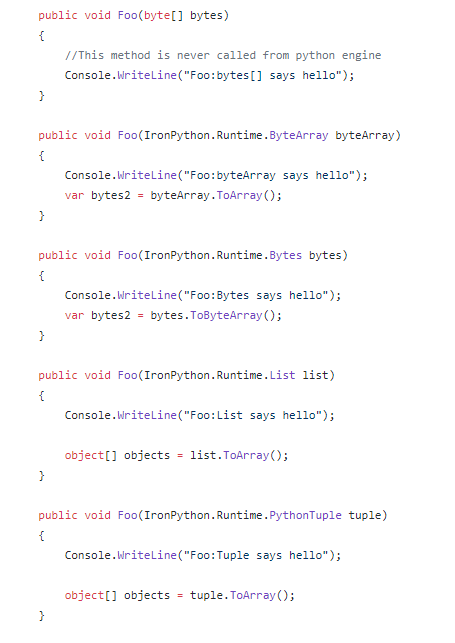
you will need different method signatures on File.Append to support the different Python types.@Fredebec:
I fixed your Python script:
I prefixed the variables with type to be clear the data type being used.
Right on - i fixed the file append and other byte array references for the next beta channel release