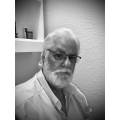
PRO
smiller29
USA
Asked
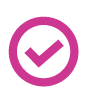
How to Control Tank Tracks Without Skid Turns?
I am planning to use an Arduino Uno with EZB firmware and two H-bridge controllers, one for each of the right and left track motors, with PWM speed control.
The way I want this to work is with a single joystick control:
- For a right turn: the left track moves forward, and the right track moves backward.
- For a left turn: the opposite of the above.
Can this be done with one joystick, or will it require a joystick for each track?
Related Hardware Arduino Genuino Uno
Controlling tank tracks without skid turns using a single joystick is definitely possible with the setup you have. You can achieve this by configuring the joystick to control the speed and direction of each track independently. Here's a step-by-step guide to set this up in ARC:
Step 1: Configure the Dual H-Bridge w/PWM
Step 2: Configure the Joystick (XInput)
Step 3: Custom Movement Panel v2
Forward Movement
Reverse Movement
Left Turn
Right Turn
Step 4: Integrate Joystick with Custom Movement Panel
Step 5: Testing and Calibration
Example Code for Joystick Integration
Here’s an example of how you can map the joystick movements to the custom Movement Panel scripts:
By following these steps, you should be able to control your tank tracks without skid turns using a single joystick. If you encounter any issues or need further assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
Absolutely all movement panels will do that.
Thank you for the information.
Here’s a list of movement panels: https://synthiam.com/Support/Get-Started/how-to-make-a-robot/Movement-Style
You can choose one that works with your type of motor. Whether hbridge or continuous rotation or motor controller etc