
chas9000c
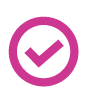
What is the recommended code (ideally in C#) for stopping the movement of a continuous rotation servo from a 3rd party manufacturer using the new EZ-B v4 controller?
Here's the code I have that's already working to spin a servo in one direction, then the opposite direction, and then stop: (The code for stopping the servo is a real kludge; seems like there should be something better.)
BodyConnection.EZB.Servo.SetServoPosition(Servo.ServoPortEnum.D16, Servo.SERVO_MAX); Thread.Sleep(1000); BodyConnection.EZB.Servo.SetServoPosition(Servo.ServoPortEnum.D16, Servo.SERVO_MIN); Thread.Sleep(1000); BodyConnection.EZB.Servo.SetServoPosition(Servo.ServoPortEnum.D16, 108); Thread.Sleep(100); BodyConnection.EZB.Servo.ReleaseServo(Servo.ServoPortEnum.D16);
Although this code works, it's a real kludge to use 108 to stop the servo. (That's just the magic number I found through trial and error that has the effect of stopping the servo.) Servo.SERVO_OFF doesn't work, because it's set to 1, not 108. It just spins the servo at a pretty fast speed in the same direction as Servo.SERVO_MIN. Servo.SERVO_CENTER also doesn't work, because it's set to 90 and spins the servo, albeit a little slower.
Is there a cleaner approach to stopping a continuous rotation servo? Something better than the code I have above?
Thanks.
Unfortunately the stop point of continuous rotation servos does vary by make, so "finding the magic number" is the way to do it.
Alan
Every modified servo's stop value will vary, with this in mind wouldn't it be better to use the "magic number" rather than setting a stop value which only works for some servos?
Edit: Alan beat me to it
Thanks. It works, so if that's the best/recommended approach, I'll use it. Just wanted to check in case there was a better approach. Thanks again.
In Example #1 of the EZ-SDK File, here is the code for modified servos..
Are you using the modified servos for making the robot move?
If so, use the Movement class. It's your ezb.Movement...
First you have to initialize the movement class
Now you can specify the directions in code
DJ Sures, thanks, but that's essentially the code I'm already using. Whether or not I set the Movement class's properties, the Servo.SERVO_OFF still doesn't stop this particular servo, which seems to need a value of 108 to stop. Of course, the Servo.SERVO_OFF code should work for any servos that use a value of 90 to stop.
If the servos do not stop when the PWM has stopped, then you can initialize the movement class for those values, like so... The "use stop value" parameter will send the specified stop PWM value
Cool, thanks!