Asked
— Edited
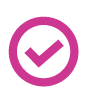
I am using the plug in for the XV-11 Lidar. The output of the plug in an array in which each value is a string that represents the distance at the angle and the signal strength. To use this for control of the robot, I need numbers at these angles. The format is shown in the attached example. How do I parse and convert this in EZ Scripting into an integer?
In C there is the string.split command
https://msdn.microsoft.com/en-us/library/b873y76a(v=vs.110).aspx
and then the string to integer convert.
Is there a way to do this in the EZ scripting?
Yeah it's split()
Look in the examples folder for syntax usage examples
Although, the makes more sense to sanitize the data before going into a variable and not have to reactively parse the variable.
I will look at making a change for this tonight. If I remember right, the first number is mm distance. The second is the intensity value and the ID of the array element is the angle. I don't swear by this as it has been a long time since I looked at it. I will break these out to 3 arrays each containing 360 elements.
Here is the question... Would you rather be able to get all of the information by querying one element in one array and then break apart the information, or would you rather get individual elements in 3 different arrays? I see this as a loss in both fronts.
Either you will have to take the variable and parse it to get the data when needed or you would need to get three elements and use more memory on the computer for this. I guess because these 3 arrays would be number values, each element would be smaller than string values, so maybe it wouldn't be more memory...
I am open for suggestions. I am good either way. The benefit to the second way is that it would be an integer that code wouldn't need to split on and then cast as an int.
Another option is to drop the intensity all together and just give you the distance with the angle being the ID of the item in the array.
Let me know which you would prefer. I am open to any ideas.
Data organizational practices, which applies to variables, is to store only the relevant data. Meaning, storing two types of data in a single variable is not good practice. This adds the responsibility on the user, which introduced high chance of error - and additional effort, such as demonstrated in this thread.
Put the data as separate array variable names for each respective attribute - and voila.
Sounds good DJ. I will do that. BTW, my memory isn't that bad. I just checked the code and I did remember what the values were. Sometimes I surprise my self
EZ_Builder.Scripting.VariableManager.VariablesArray["$LIDAR"].ElementAt(direction2).SetValue(distancetext + "|" + intensity.ToString());
I will break this out to 2 different variable arrays with the ID of the element being the angle. This will still allow the user to decide to use the intensity if needed and the ID is still the angle.
Thanks
Wow! this community is outstanding.DJ and Mr. Cochran I would like to have angle and distance even if it were in two related variables. The intensity number is probably not as useful even though it does tell you about the quality of the data. Just getting the data into variables that can be used would probably be sufficient as a first step. I can't wait to see where this goes with this collaboration!
This is completed and published. The array index is the angle. The $LIDARDistance variable is the distance in mm, and the $LIDARIntensity is the intensity reading from the LIDAR at that angle. $LIDARDistance[0] belongs with $LIDARIntensity[0].
Outstanding. I can't wait to try this out. I hope I get to fiddle with it tonight. Thank you David