
hawkeyedriver
USA
Asked
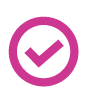
If we use these two commands back to back (and in either order) the second one executes, but the first does not.
EZBManager.FormMain.SendFormCommand("Auto Position", "AutoPositionAction", "Gorilla"); EZBManager.FormMain.SendFormCommand("Auto Position", "AutoPositionAction", "Bow");
We know we are missing something simple, but how do we issue a SendFormCommand that actually waits for an action to complete, or how can we check to see if the command is complete to build in a delay?
Thanks!
Related Hardware JD Humanoid
You must use pause between actions. Use a "wait until" perhaps?
Wait until the first action has completed. Remember, ControlCommand does not wait (from this manual page)
What I recommend doing is talking to the Auto Position directly rather than sending commands. Something like this, and makes sure the action doesn't repeat. Because you can't wait for something that will never end.
Alternatively, if you have to specify a timeout because the action repeats, do this...
Lastly, you can cover both bases with something like this...
Outstanding, thank you!!
I'll post back when I have this running but this is exactly what we needed!
Got your example code in and running, tried all variants.
It is oddly still doing the same thing with multiple calls to all of the variant runAction methods you suggested. It will run the second behavior, supporting what you and the documentation say is correct, in that it just won't wait even though the these methods tell it to.
I'm going to tinker around the edges with the delay structures, because the While...GetAutoPosition.IsRunning test doesn't seem to be doing anything or it is hanging up for some reason. A few debugs felt like they were stalling in an infinite loop especially in the version that does not use a timer.