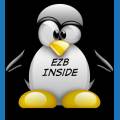
Darathian
USA
Asked
— Edited
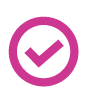
Is it possible to enable and disable for example the color tracking check box in the camera control in ARC via the SDK while conected via the telnet server?
Thanks
The EZ-SDK and ARC are different things. The ARC is a graphical interface to the EZ-SDK. The EZ-SDK is a collection of libraries and functions for programmers to create their own interface. For example, with EZ-SDK you can make your own ARC.
If you have a program that you would like to communicate to ARC - you can do so with commands sent over the selected TCP port. You will have to put the ARC Connection Server into Script Mode. You can do that by pressing the Config Button on the Connection Control.
The Script Mode will allow the incoming connections on the selected port. You can now send EZ-Script commands to the Script Mode Server's TCP Port.
For what you're asking - you can send the ControlCommand() syntax. Reference the EZ-Script manual to obtain the appropriate syntax for your question.
Hope that helps!
I enabled the TCP server and script mode is enabled and I identified the correct EZ-Script command to send. If I send it via just a normal telnet client the string works but via my code it does not.
I am pretty new to EZB and C# and haven't coded in ages.
Is the Method:EZ_B.TCPClient.WriteLine(System.String) method the correct method to use to send the string?
I can post the code if that would help.
Thanks
Correct me if I am wrong but looking at the control commands it seems like it is not possible (unless I missed something) to read an external variable value sent via the TCP port so it can be used in EZ-Script.
Thanks
As DJ stated if you are using ARC you do not need to use the SDK. It's one or the other. ARC is, in basic terms, a GUI for the SDK but has it's limits to the pre-defined controls.
You can send and receive variables and commands through TCP, I have it set up on Jarvis to do so. Your other application can use all of the control commands or anything within EZ-Script. If you wanted to send a variable to ARC you just need to send the code
in whichever language you are using to transmit via TCP.
To send the other way (ARC to Application) switch it around so
Where "variable" is the external applications variable name and "$x" is the ARC variable.
You could also use HTTPGet to pull data from other applications, web pages and sites or execute web based applications.
First let me say thanks to both of you for your answers. I value your input.
What I am trying to do is this:
Perhaps I need to phrase the question in a better way.
Is there a built in method in the SDK that I can use to send a Control string for example cc("Camera", CameraColorTrackingEnable) or $x = variable to the TCP server?
Thanks
Here's an example of setting and getting EZ-Script variables over the Script Interface. Also, I used a ControlCommand() to set the Face Tracking (notice the checkbox next to Face on the camera control)
I will whip up a quick little C# demo for you on connecting to ARC via script interface and post it here. But, I have to have lunch first
@DJ Sures That would be awesome and thank you very much. Your help is appreciated.
My App can wait, go have some lunch
I knew my connection to the TCP server was working using the EZ_B.TCPClient.Connect method to connect since I could read the response "ARC v2013.12.30.00 on TTY0" back into a c# variable in my app using the TCPCLient class and Networkstream method and I could see the connection come into the ARC debug window from the app.
I think my issue is how I am trying to send the string to the TCP server. An example would help very much indeed.
Here you go.. Make sure you have a Camera Control added to your project for the camera buttons in this example to work.
Tutorial52-ScriptInterfaceClient.zip