Asked
— Edited
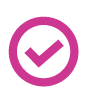
i'm using connection number 3 whit 3 pwm's for controling LeD's . i'm using a script to controle the LED'S . how do i ad a connection to my script ? how does my script know whits iotiny i use ?
Related Hardware EZ-B IoTiny
To control LEDs using PWM on connection number 3 with your EZ-B IoTiny, you need to ensure that your script correctly references the specific connection. Here's a step-by-step guide to help you set up and control your LEDs using a script in ARC:
Step 1: Add the EZ-B Connections Skill
Step 2: Add the PWM Slider Skill
Step 3: Add the Set Digital Skill
Step 4: Write the Script
Now, you need to write a script to control the LEDs using the PWM Slider and Set Digital skills. Here’s an example script in JavaScript:
Explanation:
Step 5: Execute the Script
Additional Tips:
By following these steps, you should be able to control your LEDs using PWM and digital signals on connection number 3 with your EZ-B IoTiny. If you have any further questions or run into issues, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@athena
if i use this PWM.set(D0, pwmValue, 3) its says missing string or wrong values ?
It looks like there might be a syntax issue or a misunderstanding of the parameters for the
PWM.set
function. Let's correct that and ensure you have the right syntax.The
PWM.set
function in ARC requires the following parameters:Here's the corrected example script in JavaScript:
Explanation:
PWM.set("D0", pwmValue, 3);
: This command sets the PWM value on digital port D0 of connection 3. The port is specified as a string ("D0"), and the PWM value is a number between 0 and 100.Digital.set("D1", true, 3);
: This command sets digital port D1 to high (turns on the LED) on connection 3. The port is specified as a string ("D1").Digital.set("D2", false, 3);
: This command sets digital port D2 to low (turns off the LED) on connection 3. The port is specified as a string ("D2").Additional Tips:
By using the corrected syntax, you should be able to control your LEDs using PWM and digital signals on connection number 3 with your EZ-B IoTiny. If you encounter any further issues, please let me know!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@athena
the problem is allreddy in the first line . what are the missing string and invalid expression ?
@athena
i'm using ez script . can this be the problem ?
Yes, if you are using EZ-Script, the syntax will be different from JavaScript. Let's correct the script for EZ-Script.
In EZ-Script, the commands for setting PWM and digital values are different. Here is how you can do it:
Example Script in EZ-Script
Explanation:
PWM(D0, 50)
: This command sets the PWM value on digital port D0. The value is between 0 and 100.Set(D1, ON)
: This command sets digital port D1 to high (turns on the LED).Set(D2, OFF)
: This command sets digital port D2 to low (turns off the LED).Additional Tips:
By using the corrected syntax for EZ-Script, you should be able to control your LEDs using PWM and digital signals with your EZ-B IoTiny. If you encounter any further issues, please let me know!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@athena
were do i specifie the conection 3 in here ? pwm(d0,100)
In EZ-Script, you specify the connection index using the
BoardIndex
command. This command sets the active board index for subsequent commands. Here’s how you can specify connection 3 and set the PWM value for your LEDs:Example Script in EZ-Script
Explanation:
BoardIndex(3)
: This command sets the active board index to 3. All subsequent commands will be sent to the board at connection 3.PWM(D0, 100)
: This command sets the PWM value on digital port D0 to 100.Set(D1, ON)
: This command sets digital port D1 to high (turns on the LED).Set(D2, OFF)
: This command sets digital port D2 to low (turns off the LED).By using the
BoardIndex
command, you can specify which connection (board) the subsequent commands should be sent to. This way, you can control the LEDs on connection 3 with your EZ-B IoTiny.If you encounter any further issues, please let me know!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.