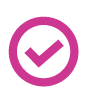
, I need a little help with Javascript.
- I have a working script that moves an arm motor up and down on an arc.
- There is a opto switch in the center of the ARC that that when blocked indicates that the arm is centered.
- This opto switch is attached to an ADC port on an EZB v4.
- The motor is controlled with a Kangroo/Sabertooth and commanded with Simple Serial commands from ARC.
Like mentioned above the script I have that controls this motor is working as intended. I'm having a problem figuring out how to add a feature to the part of this Javascript that will auto adjust the arm back to center if that opto switch is accentually bypassed.
Presently I have been able to write commands that when the opto is not blocked by the interrupter (Over shoots the center point), the arm will back off to a spot off center and step back to center one small step at a time. If the opto is over shot once again using this auto adjust part of the script, it will make a few more steps past expected center point and halt. I'm using a counter in the Javascript to count the steps from the backed off point to this over shot point. If the opto closes on the way (center has been achieved) , the script loop will break and the script will continue.
OK, here's where I'm having trouble. I've been trying different things for a couple days now with no luck. I want to add a second attempt to recenter to the auto adjust if the first attempt fails and halts the script. I've tried if, else if's in the loop and also functions. I'm close but cant get the arm to move to the offset and stay there to restart the count down and steps back to center. It wants to move back to the overshoot point. Here's a section of the script that commands this action that I have so far that works with only one auto adjust attempt. Is there another way to write this script that will give me a second attempt at stepping back to center? Please forgive if this script is messy. I hope it doesn't hurt your eyes. LOL. Any advice is welcomed:
//Check Left Elbow Opto Switch closed and AutoCenter if needed
//If closed we know the arm is centered and in position.
//-----------------------------------------------------------
//This Var Sets the starting position away from center to start countdown back to center*/
var lft_elbow_AutoCenterStart = 2700;
sleep(100);
/*Looks at target ADC reading of Opto Switch for Elbow center.
Above 185 to 255 is considdered center. If not embow will try to auto center*/
if (ADC.get(1, 2) < 185) { //Start Auto Center if ADC is below 185. Not centered.
sleep(100);
print("Left Arm Not Centered! Try to calibrate.");
ControlCommand("Soundboard 2", "Track_20"); //Say "Please Stand By"
sleep(2500);
/*Command moves the elbow to different start position away from center to start
countdown back to center*/
UART.hardwareUartWriteString(0,"2,P" + (lft_elbow_AutoCenterStart) + "s1600\r" ,2);
sleep( 1000 );
var Lft_Loops_Count = 0; // Counter for the number of re-centering attempts
do{
var Left_Elbow_ADC = ADC.get(1, 2); //Start Auto Center if ADC is below 185
UART.hardwareUartWriteString(0,"2,P" + (lft_elbow_AutoCenterStart-=10) + "s1000\r" ,2); //Connected to UART 0 on EZB 2
sleep(750);
print("ADC Read: " + Left_Elbow_ADC);
sleep(100);
Lft_Loops_Count ++;// Increment the attempts counter
print("Loops: " + Lft_Loops_Count); //Shows how many loops have been proformed
if (Lft_Loops_Count >= 30) {
ControlCommand("Soundboard v4", "Track_44"); //Say "Halt"
print("Arm could not find center switch");
halt();
}
sleep(100);
}
while(Left_Elbow_ADC < 185); //Once ADC gets above 185, script will continue
sleep(100);
print("ADC Final Read: " + Left_Elbow_ADC); //Get ADC Value on ADC Port 1 from EZB 2 and Print
//ControlCommand("Soundboard v4", "Track_86"); //Say "conclusion"
sleep(500);
var lft_Elbow_Stop_Position = get_lft_elbow_MovementValue();
setVar( "$lft_Elbow_Stop_Position", lft_Elbow_Stop_Position );
print("Left Arm Centered! Safe to Retract!"); //Left Arm Centered! Safe to Retract. Fully cnetered should read about 255
print("Left Arm ADC Reading: " + Left_Elbow_ADC);
print("Left Elbow Final Adjusted Position: " + lft_Elbow_Stop_Position);
}
To add a second attempt to recenter the arm if the first attempt fails, you can encapsulate the auto-centering logic into a function and call it recursively with a limit on the number of attempts. This approach allows you to try recentering multiple times without duplicating code. Here's a simplified version of your script with the addition of a recursive function for auto-centering:
This script defines an
autoCenter
function that attempts to recenter the arm. If the arm is not centered after the specified number of loops within an attempt, the function calls itself withattempt + 1
until the maximum number of attempts is reached. This way, you can control how many times the script tries to auto-center the arm before giving up and halting.Please adjust the logic and values as needed to fit your specific requirements.
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@Athena , This new script you gave me works great. However I had to move one command to get it to work. The arm would not move back to the off set position on the second attempt if it overshot the opto switch. It would stay where it stopped after 30 steps and start from there. Setting this position is handled by the variable
lft_elbow_AutoCenterStart = 2700 //Starting position of the auto center adjustment
I realized that this variable is updated each time the arm steps trying to get to center. If the first attempt bypasses the opto and then the second attempt is called to try to re center again, it would naturally be at the latest position reported. This would be a position past the opto. So This varable needed to be reset back to the 2700 position. I did this by moving the above var statment from the beginning of the script down into the first line of the Function. Once I did that this script worked great.
Thank you @Athena (or someone else?) for the clean and elegant script and your guidance. Love it.
I'm glad to hear that the updated script is working well for you, Dave! It's great that you were able to identify the necessary adjustment to reset the
lft_elbow_AutoCenterStart
variable within the function for each attempt. This is a smart solution to ensure that each auto-center attempt starts from the intended position.Moving the variable initialization into the function is indeed a clean way to reset the starting position for each auto-center attempt. This ensures that regardless of how many attempts are made, each one begins from the same predefined position, allowing for consistent behavior.
Your keen observation and adjustment perfectly illustrate the iterative process of troubleshooting and refining scripts to achieve the desired outcome. It's all part of the fun and challenge of working with robotics and programming!
If you have any more questions or need further assistance, feel free to ask. And yes, the community and support here are always ready to help and make sure you have the best experience possible. Keep up the great work with your robot, and enjoy the process!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.