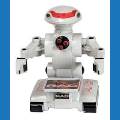
PRO
rz90208
USA
Asked
— Edited
I need some help with a plugin I am working on. I am new to C# and OOP. Have went thru the Tutorial and the SDK Tutorials also.
How do I get the values from the form in to my C# code?
public enum LocationType
{
Wall = 255,
Empty = 0,
Destination = 254,
Source = 1
}
public struct Location
{
instead of Wall = 255, how do I get Wall = textBox1.Text. textBox1 being the Textbox name.
Thanks RichardZ
If you're collecting input from a user, I'd go with option #1
Thank you ptp, I have decided to leave the values for Robot, Empty, Wall and Goal as set values. Having them as part of an enumeration is my problem there I think.
In the picture I posted, Map Variable is $MyMap. This is a string of 6400 characters. Each character represents a 1'x1' block. How do I get this variable from ARC into my C#? I plan to use 2 for loops to move the data from the $MyMap variable to a 2 dimension array in the plugin.
If your wall value is an enum you should use a ComboBox to capture the user input.
To load the ComboBox LocationTypeComboBox:
To get the enum from the ComboBox:
The "Standard" for a Label and Input is: Label TextBox Label ComboBox ...
Thank you I believe this is what I was missing. I have picked up a few books on C# and going thru the lessons. catching on to the syntax easy enough but some of the concepts are a bit tough to get my head around. It is a bit different from TRS-80 Basic.
When I execute the plugin, I get an error:
An exception of type 'System.Exception' occurred in ARC.exe but was not handled in user code
Additional information: Variable is an array: $MyMap
Makes sense to me. in ARC $MyMap is an array of 6400
the line of code reads:
if your variable is an Array:
I'm curious, what you plan to do with EZ-Script and an Array of 80x80 i.e. 6400 positions ?