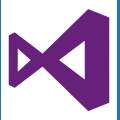
patrick_c#
Hello, i tried to program my EZ Robot with C# under Ubuntu 15.04.
using System; using EZ_B;
public class HelloWorld { public static void Main() { Console.WriteLine("Enter IP of EZ-Robot"); string ip = Console.ReadLine(); Console.WriteLine("IP: "+ip ); Console.WriteLine("trying "+ ip); Console.WriteLine("trying to connect with " +ip);
//connecting
EZB _ezb = new EZB();
_ezb.Connect(ip);
Console.WriteLine("connecting...");
if (_ezb.IsConnected)
{
Console.WriteLine("[+] EZB connected at ip: " + ip);
}
else
{
Console.WriteLine("[-] EZB NOT connected at ip: " + ip);
}
}
}
Thats my code, but if i execute it...
patrick@patrick-X781X-X782X:~/ezrobot$ mono bsp.exe Unknown heap type: #GUlD
Unknown heap type: #Blop
Enter IP of EZ-Robot 192.168.1.1 IP: 192.168.1.1 trying 192.168.1.1 trying to connect with 192.168.1.1 Missing method .ctor in assembly /home/patrick/ezrobot/EZ_B.dll, type System.Runtime.CompilerServices.ExtensionAttribute Can't find custom attr constructor image: /home/patrick/ezrobot/EZ_B.dll mtoken: 0x0a00000a
Unhandled Exception: System.TypeLoadException: Could not load type 'System.Runtime.CompilerServices.ExtensionAttribute' from assembly 'EZ_B'. at HelloWorld.Main () [0x00000] in :0 [ERROR] FATAL UNHANDLED EXCEPTION: System.TypeLoadException: Could not load type 'System.Runtime.CompilerServices.ExtensionAttribute' from assembly 'EZ_B'. at HelloWorld.Main () [0x00000] in :0
There must be a mistake with the "ez.Connect" command....
Thanks for help, Patrick.
There is no mistake, as it compiles and runs fine on my test. I would recommend using the universl bot library instead, as it also provides you access to the source code.
My problem is solved
"EZB _ezb = new EZB();" must stand before void main
But now I have this issue:
dmcs -r:EZ_B.dll bsp.cs bsp.cs(16,9): error CS0120: An object reference is required to access non-static member `HelloWorld._ezb' Compilation failed: 1 error(s), 0 warnings
in Line 16 is "_ezb.Connect("192.168.1.1");"
As you can imagine, it's difficult to assist when I only see a small fragment of the project. If you insist on moving forward with the mono library, please post your project or complete code and we'll get you running
Are you also using Linux? Which compiler do u use? How do you compile it? Thanks for your time
using System; using EZ_B;
public class HelloWorld { EZB _ezb = new EZB(); //this stands now before ""void main"
public static void Main() { Console.WriteLine("Enter IP of EZ-Robot"); string ip = Console.ReadLine(); Console.WriteLine("IP: "+ip ); Console.WriteLine("trying "+ ip); Console.WriteLine("trying to connect with " +ip);
//connecting
_ezb.Connect(ip); Console.WriteLine("connecting..."); if (_ezb.IsConnected) { Console.WriteLine("[+] EZB connected at ip: " + ip); } else { Console.WriteLine("[-] EZB NOT connected at ip: " + ip); }
} }
in simple form:
using System; using EZ_B;
public class HelloWorld { EZB _ezb = new EZB();
}
console output:
patrick@patrick-X781X-X782X:~/ezrobot$ dmcs -r:EZ_B.dll bsp.cs bsp.cs(12,9): error CS0120: An object reference is required to access non-static member `HelloWorld._ezb' Compilation failed: 1 error(s), 0 warnings
This is because the MAIN() is declared as static. The error message explains it. It says, an object is required to access a non-static member, and gives you the object, which is _ezb.
An error is written text to explain what the issue is. The error says the object _ezb is non-static but is being called from a static method.
As you can see, the method Main() is static, and the variable ez_b isn't.
Either make the _ezb static, or make Main() not static. I'm not sure if you can make Main() not static, but try and let me know
. Otherwise, make _ezb static.
Here's a document i found for you that discusses the difference between static and non-static declaration: http://www.c-sharpcorner.com/uploadfile/abhikumarvatsa/static-and-non-static-methods-in-C-Sharp/
Thanks for answering,
if i make the main void non static .... public void Main() .....
there is an error: error CS5001: Program
bsp.exe' does not contain a static
Main' method suitable for an entry pointso I think void main must be static.
Then i tried to make _ezb static:
using System; using EZ_B;
public class HelloWorld { static EZB _ezb = new EZB();
public static void Main() { Console.Clear(); Console.WriteLine("Enter IP of EZ-Robot"); string ip = Console.ReadLine(); Console.WriteLine("IP: "+ip ); Console.WriteLine("[+] trying "+ ip); Console.WriteLine("[+] trying to connect with " +ip);
//connecting
Console.WriteLine("connecting..."); _ezb.Connect(ip); Console.WriteLine("[+] connecting process finished"); Console.WriteLine("wating for result...");
if (_ezb.IsConnected) { Console.WriteLine("[+] EZB connected at ip: " + ip); }
else { Console.WriteLine("[-] EZB NOT connected at: " + ip); }
} }
it compiled
but if I execute it....
patrick@patrick-x781x-x782x:~/ezrobot$ mono bsp.exe Unknown heap type: #GUlD
Unknown heap type: #Blop
got wrong token: 0x6d22a7bf
Unhandled Exception: System.TypeInitializationException: An exception was thrown by the type initializer for HelloWorld ---> System.TypeInitializationException: An exception was thrown by the type initializer for EZ_B.EZB ---> System.BadImageFormatException: Bad method token 0x6d22a7bf on image /home/patrick/ezrobot/EZ_B.dll. --- End of inner exception stack trace --- at HelloWorld..cctor () [0x00000] in <filename unknown>:0 --- End of inner exception stack trace --- [ERROR] FATAL UNHANDLED EXCEPTION: System.TypeInitializationException: An exception was thrown by the type initializer for HelloWorld ---> System.TypeInitializationException: An exception was thrown by the type initializer for EZ_B.EZB ---> System.BadImageFormatException: Bad method token 0x6d22a7bf on image /home/patrick/ezrobot/EZ_B.dll. --- End of inner exception stack trace --- at HelloWorld..cctor () [0x00000] in <filename unknown>:0 --- End of inner exception stack trace --- patrick@patrick-x781x-x782x:~/ezrobot$
In Visual Studio under Windows it worked perfectly...
If i get this running, I will make a Tutorial on your Website to help other users
I think you cannot make _ezb static...
I updated instructions on the mono ez-sdk homepage for you. Here is the link: http://synthiam.com/Products/ARC
I also re-compiled the SDK with a signature, which may help your platform as well. There is additional information i added to help you.
Also, please start wrapping your code that you paste in this forum using the code tags. There are UBB Code instructions to the right of the entry form. It is difficult to read without being properly formatted. It's also a good habit if you are set out to advance robot skills.
Thank you very much

It works
In the future I will wrapp my code
Should I make a Tutorial for it? It would be the least thing for your help
one more question blush
why dont´t this work? How do you use servo fine tune? It also don´t worked under Windows...
Please write a tutorial! That would be wonderful.
Alan
ok, I´ll start tonight
Fine tune offsets every servo position by the number provided.
This is to make a servo calibrate.
It does work in Windows.
Hi, I know what Fine Tune is
But it don´t work...
with this command
I just ran that command with no errors - are you executing that method before or after the ez-b is connected?
Setting the fine tune after the servo position is set is backward approach.
Try this...
Set the fine tune before you use the servos - because that's an offset which the servos will require.
PS, thanks for wrapping your code
Much easier for my eyes!
I tried exactly the same code as you posted, but the same error:
[u]bsp.cs(12,14): error CS1056: Unexpected character
­' bsp.cs(12,21): error CS1056: Unexpected character
' bsp.cs(12,25): error CS1056: Unexpected character­' bsp.cs(12,31): error CS1056: Unexpected character
' bsp.cs(12,31): error CS1525: Unexpected symbolFine', expecting
,',;', or
=' bsp.cs(12,36): error CS1056: Unexpected character­' bsp.cs(12,42): error CS1056: Unexpected character
' bsp.cs(12,49): error CS1056: Unexpected character­' bsp.cs(12,55): error CS1056: Unexpected character
' bsp.cs(12,60): error CS1056: Unexpected character­' bsp.cs(12,70): error CS1056: Unexpected character
' bsp.cs(12,70): error CS1525: Unexpected symbol5', expecting
,',;', or
=' Compilation failed: 12 error(s), 0 warnings [/u]Not sure where those errors are coming from - but looks like something broken in Mono (not surprising). Try reinstalling mono? Ensuring you have the latest version and dependencies? There are no known issues with the entire EZ-Robot mono library - i ran the entire test last night.
When googling the error "error CS1056", it could be caused by a European character in code: https://msdn.microsoft.com/en-us/library/59k0x971.aspx
Mono, like most programming languages, it not friendly to all character sets. Given that it's complaining about the commas "," and semicolons ";" or something.
It indeed looks like strange unicode characters in your code. For example, when i copy and paste the errors that you posted into Notepad.exe, this is what i see.
Notice how after filtering the code that you posted through notepad (Which removes any bits outside of the ascii-7 charset), displays different errors.
Somehow, your keyboard is putting strange characters into the code while you type.
Here is additional information regarding the error. It appears using a non-us keyboard character-set will cause problems with mono. I know that it will cause problems with GCC C/C++. I guess mono is included in that list..
Such as this: https://teamtreehouse.com/community/compiler-error-in-if-else-statement
I believe the issue is with the character-set on your pc.
Hi
i googled and found that somebody installed Mono 4.0 and it worked. On the Mono homepage i found "Mono develop". A very useful program.
Could be "Visual Studio Lite" for Linux
But there are still the same errors. With the newest Mono version....
I´ll try something else tomorrow, by me it´s 11:48 PM
Good night, and thanks for your time
Hi,
I am using Ubuntu 15.04. With german keyboard layout.
I am woundering about, that if i delete this line
it compiles without no warnings.
if i use this code, there are the errors i posted....
I think it's because the line was typed vs copy and pasted. It's going to be difficult for me to diagnose this one because I don't have a German keyboard or am I on the mono development team. They sadly do not have a great forum for assistance. Can you change the character set of your Linux installation to USA keyboard? That seems to be mono's solution to this.
There's an update to the mono library, which includes a more efficient camera thread, audio thread, and Auto Position thread.
Hi,
I have also no English keyboard... :/ But I found an old (very old) Panasonic Toughbook CF29 with Englisch keyboard. I´ll try later with an englisch installed Kali Linux. Thanks for your time!
Anytime
It might also be the selected character set in the operating system - not just the keyboard.
lol, if I set the keyboard layout to "EN" and write the
with EN settings, it compiles. So I have to set only the keyboard layout.
Difficult to find the right keys

Thanks for your great time and help
Lol, I want to work at ezrobot