Asked
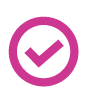
How to write the code in Python for "audio.say" to instead "print(text)"?
import time import random
def SayWait(text, wait_time): print(text) time.sleep(wait_time)
Story 1
def story1(): SayWait("Once upon a time, there was a dear little girl...", 1)
Story 2
def story2(): SayWait("What a tender young creature.", 1)
Randomly choose a story
chosen_story = random.choice(["Story1", "Story2"])
if chosen_story == "Story1": story1() print("\n--- Story 1 Ends ---\n") elif chosen_story == "Story2": story2() print("\n--- Story 2 Ends ---")
Related Hardware EZ-B v4
To use the
Audio.say
command in Python within ARC, you would typically use theAudio.say
method provided by the ARC Python API. However, since you want to replace theAudio.say
with aprint
statement, you can simply use theprint
function in Python to display the text in the console or log window of ARC.Here's how you can modify your existing Python code to use the
print
function:Please note that the
print
function will output the text to the console or log window within ARC, and it will not be spoken out loud. If you want the text to be spoken by the robot, you would need to use theAudio.say
method provided by ARC's Python API, which would send the text to the speech synthesis engine to be spoken out loud by the robot.If you do want to use the
Audio.say
method to have the robot speak the text, you would need to use the ARC Python API to send the command to the speech synthesis engine. However, since you've asked to replaceAudio.say
withprint
, the above code should meet your requirements.I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
Thanks a lot!