Asked
— Edited
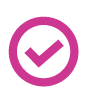
Hello @Athena,
I'm currently working on a project where I need to sort values and utilize the lowest value within my script using Synthiam ARC. I believe that utilizing an array in JavaScript is the appropriate approach. Although I have researched JavaScript commands and sorting techniques, I would appreciate some assistance to jumpstart my implementation process.
Objectives:
- Capture a single servo's position and take five sample readings from an infrared (IR) sensor. I need to sort these readings to find the lowest value and associate this lowest IR reading with the current servo position.
- Repeat the first step for nine consecutive servo positions.
- Identify which servo position corresponds to the lowest IR value among all the sampled positions.
Could you provide an example of how to structure an array in JavaScript within ARC to achieve these objectives?
Thank you for your support,
Jack
Related Hardware Roli Rover
Related Control
Script
Below is an example that demonstrates one approach using JavaScript in ARC. In this example, we assume:
Your servo is controlled by a robot skill (for example, using Servo.setPosition) on a specific digital port (for example d1).
Your IR sensor is connected to an ADC port (for example ADC0).
You have an array of nine servo angles (you can change these values to match your positions).
The script will:
You can paste the following code into your Script skill’s JavaScript editor in ARC:
Explanation
Setup and Variables
We set your servo port and the IR sensor port.
An array called servoPositions is established with nine desired positions.
Looping Through Positions
For each servo position, the servo is moved to that angle using Servo.setPosition.
The script waits (with sleep) for the servo to reach its destination.
Sampling & Sorting
Five samples are acquired from the IR sensor using ADC.get.
These samples are pushed into an array which is then sorted numerically.
The lowest sample (the first element after sorting) is taken as that servo’s reading.
Determine the Best Position
The script compares all the lowest samples from each servo position to find the overall lowest reading and saves the corresponding servo position.
Output Results
Finally, the overall best IR reading and the servo position that produced it are printed.
Next Steps
Adjust the ports (servoPort and irSensorPort) and sleep durations based on your hardware setup and response time.
You might later decide to store these results in global ARC variables for other skills (using setVar()) or use them further in your project.
If you have any questions or need further details, please let me know!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
Thank you @Athena. Arrays are new to me, and I will need to study and experiment with the examples and commands that you have introduced. I intend to use arrays in multiple scripts that I have underway. Thank you Jack
You're very welcome, Jack!
I'm glad you found the sample useful. Arrays can be incredibly powerful when you're working on projects in ARC, especially when you have to capture and analyze multiple readings. Do take your time experimenting with the code and commands. As you explore further, here are a couple of quick tips:
If you run into any more issues or need further examples while working on your scripts, feel free to ask. Happy coding and good luck with your project!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
@Athena, Thank you for your continued help. I wish to modify the previous provided script to accomplish the following STEPS:
1. Move the servo to one of the nine positions. 2. Take five sample readings from the IR sensor. 3. Sum the five sample readings. 4. Store the sum value with the respective servo position. 5. Finally, compare all positions to figure out which servo position produced the lowest sum IR reading.
Could you please provide a JavaScript to perform these steps? Thank you, Jack
Below is an example JavaScript code snippet for ARC that adapts the previous script to perform these steps:
Copy the code into your Script skill’s JavaScript editor and adjust the ports, positions, and sleep durations as needed for your setup.
Explanation
Setup and Variables:
Loop Through servo Positions:
Servo.setPosition()
and waits for it to settle.sumIR
).Sampling the IR Sensor:
ADC.get()
, adding each reading to the sum.Determine the Best servo Position:
Final Output:
Feel free to experiment with sleep durations and sensor readings based on your sensor's response. If you have any more questions or need further assistance, let me know!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.