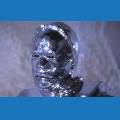
PRO
RoboHappy
USA
Asked
— Edited
Im a little confused here. I'm simply trying to read the value coming from my IR sensor (one of the Sharp IR ones). The script I wrote should should be a simple loop and display the value, can continue to display the value until I tell it to stop.
goto(sensor)
:sensor
$IRSensor = GetADC(ADC4)
# Sharp IR Sensor
print($IRSensor)
sleep(1000)
Return()
It seems to run only a couple of times then stops. That's my issue, why it displays the values twice then stops. What am I missing? confused
script to perform a loop reading:
your code only prints two readings and the behavior is correct, let's investigate how return works:
let's follow the execution:
conclusion 2 readings
@ptp ok, I think I see it now.I was following a method I've used when programming a Basic Stamp. next test will be to add a ping sensor, then to add a specific value to each to cause some action to happen.
@ptp
This was my next test, which am happy to say works!
Next, since I have 3 stationary ping sensors to add in, and want to run the IR on a servo to sweep.
So I've added a few more sensors, and am able to read the values. But now I wanted to add a couple of mechanical bumper switches. The swicthes work fine in a seperate script, but not quite sure how to add it into this script. Im sure it has something to do with it being in the proper spot within the code,but not quite getting it. Any ideas? Thank you
confused confused confused
it's important to understand how ADC_Wait works and the impact in a loop script.
is similar to:
it's easy to understand each ADC_Wait blocks the script until the condition is met, the delay is used to define how frequent ARC pulls an ADC reading from the EZB.
The question is this what you want ?
if you don't want ADC_Wait to block the script you should change to :
@ptp Morning! yes that is correct, I don't want the ADC to Wait. I want to run and loop just like the sensors are doing. In most cares the bumpers will act as a secondary if any of the other sensors failed to stop the robots forward motion.
The ultimate goal will be to have the IR sensor on a servo to sweep, but will only sweep after one of the other sensors have been triggered. Then the robot performs another task,etc. Thank you again. I will try some more experiments tonight,love to see what happens!
So I've made alt of progress with adding all the sensors in my test routine here. My newest attempt was the IR sensor as a radar (is servo on SSC32- V0). I figured out how to use the ControlCommand("Sharp IR Radar", PauseOn) and pauseoff commands. What i really would like to figure out now is if there is a way to make the Sharp IR Radar command only be activated when called upon to do so, then made to stop when told to do so. I didn't see anything usuefull within the "cheat sheet". Is there another command to use I may have missed? or a script to use?
eek
Hi, RoboHappy. Why are you using the analog ports for the bumpers. It is a simple 1 or 0 contact, use the digital ports and save the analog for future use. Regards.
@proteusy That is true, I could use the digital ports. At least now I could since I've ported all the servos over to the SSC32 and in fact freed up the digital ports. At the time I did not have any more ports left except the adc, so wanted to learn best way to use with the switches since I've seen it done before on the forums earlier. I still may do the digital route again, but for now I'm sticking with the adc, and the best, for me, at least it is getting some more programming experience out of it the best part with the help of this great community here.