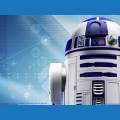
PRO
Charel
Netherlands
Asked
— Edited
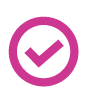
I would like to read the signal incoming from an RC receiver. How do I do that? Use-case: I drive my robot with radio-control, animations are automatic and controlled by EZ-B. Still want to pass on radio signals to EZ-B to trigger certain animation scripts. How can I read the radio signal I get in the receiver. From a receiver I can steer a servo by just plugging it in, how can I get that signal to EZ-B in an easy way. Maybe I am overlooking something very simple but spend some time searching and still no hint.
Related Hardware EZ-B v4
So let's brainstorm the idea:
you want to connect a RC receiver to EZ-B, so EZ-B has digital ports 0/1 values, analog ports can read 0-255, and serial ports (RX). How do you plan to connect this RC signal ? Let's imagine you hook it to an ADC port.
ARC software: you can read ADC port, how frequent you want to read the ADC port ? To start each second, so your ARC logic reads a value (0-255) each second what you plan to do with it ?
Bear in mind you are not streaming the values, you are polling the values from the EZ-B so the reaction time is constrained to specific intervals e.g. 1 second, 1/2 second, 1/4 second or less if you use a wired connection.
Deconstructing a "simple idea" can help you understand the limitations and the possibilities.
If you want to implement your idea you will need extra hardware and/or code/copy-paste some arduino code and then you will need to code your logic on ARC.
What @PTP said is spot on. Just to add to his assessment, is that you will need to decode the signals coming from the RC Receiver in order to use those specific commands to trigger events. There are Arduino projects out there that could do it, all that's required is to decode a PWM servo signal. It's likely that ARC could do this job (with any EZ-B) but it would have to be added as a feature request, as I don't think it exists yet.
ADC is an option too as different PWM servo signals will also be different in their analog voltage representation.
You could also simply use the EZ-B to trigger events/actions when a certain channel goes high, this would just require a bunch of channels and some scripts running in ARC.
Thank you for the quick feedback and time to give an answer. The short summary is: not a simple solution that can be handled in SW currently. Looks like pwm to voltage converter is the best option, will do some internet searching. Thanks!
ADC pins (EZ-B v4 has 8) do automatic PWM to voltage conversion, give it a shot!
That is what I tried first but if I connect RC signal to an ADC pin I didn't detect a signal (eg different voltages) when I operated the remote control?
Hmmm....you are correct @Charel, it seems that the average voltage is quite low and the EZ-B's ADC has a hard time picking it up. The voltage is between 0.16V and 0.4V (0-180 servo signals). You could likely use a DAC chip or an RC Low-pass filter to make this work.
I have solved it the following way, I left a note for others to find it and hopefully provide a solution.
I bought a simple Arduino which is reading the PWM RC signals and sending the results via I2C to EZ-B. Arduino: Arduino Pro Mini 328 - 5V/16MHz.
I need to read 6 RC channels, 2 channels for a joystick (throttle, rudder) and 4 channels for levers (SC, SF, SA, SD). The levers can have 2 or 3 positions. The joystick I wanted to have a range from -25 to + 25.
Arduino code
And the follwing code is running in the EZ-B
Hope it is useful for you!
The Arduino code above worked perfectly for an EZ-B connected over wifi, but somehow does not work if your EZ-B is connected over serial/USB. The problem is if the RC has not yet been connected, the Arduino transmits "INVALID" (by design) and serial/UCB connected EZ-B hangs itself after some time. It reads numerous "INVALID"'s correct but after some time it hangs. the Arduino code below solves that problem (but it does not work for an wifi connected EZ-B), sigh.