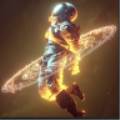
dreamwalklabs
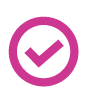
I am currently using the Bing Speech recognition robot skill to activate the Openai Chatgpt robot skill for conversation with my robot. It’s working pretty well but I need to fix 2 things:
I need to activate the conversation with a button press (contact closure) instead of a wake word. What is the best way to go about this? (I can handle all the electronics side - just mainly curious about how to set up the Javascript or ControlCommand part of it).
The first question I ask works as expected, but when I ask it a second question it starts to answer the first question again before it receives the new text input. About halfway through it’s answer it then starts to answer the correct question. How can I fix this issue?
Any help would be greatly appreciated, Thank you!
Your question was moved from a comment of an unrelated thread to a new question. @athena please answer the question above.
Great to hear that your project is coming along well! Let's address your two issues one by one.
1. Activating Conversation with a Button Press
To activate the conversation with a button press, you can use a digital input port on your EZB to detect the button press. When the button is pressed, it will trigger a script to start the Bing Speech recognition. Here's how you can set it up:
Connect the Button to EZB:
Create a Script to Start Bing Speech Recognition:
Write the Script:
Digital.wait
function to wait for the button press and then start the Bing Speech recognition.Here is an example script in JavaScript:
2. Fixing the Overlapping Responses Issue
The issue you're experiencing with overlapping responses can be fixed by ensuring that the previous response is completed before starting a new one. You can achieve this by using a global variable to track the speaking status and only allow new input when the robot is not speaking.
Create a Global Variable to Track Speaking Status:
$IsSpeaking
, to track whether the robot is currently speaking.Modify the Bing Speech Recognition Script:
$IsSpeaking
variable before sending the new input to OpenAI ChatGPT.Here is an example script in JavaScript:
$IsSpeaking
variable is set to false after the response is completed.Here is an example script in JavaScript:
Summary
By implementing these changes, you should be able to activate the conversation with a button press and prevent overlapping responses. If you have any further questions or need additional assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
I am currently using the Bing Speech recognition robot skill to activate the Openai Chatgpt robot skill for conversation with my robot. It’s working pretty well but I need to fix 2 things:
I need to activate the conversation with a button press (contact closure) instead of a wake word. What is the best way to go about this? (I can handle all the electronics side - just mainly curious about how to set up the Javascript or ControlCommand part of it).
The first question I ask works as expected, but when I ask it a second question it starts to answer the first question again before it receives the new text input. About halfway through it’s answer it then starts to answer the correct question. How can I fix this issue?
Any help would be greatly appreciated, Thank you!
@Athena I am currently using the Bing Speech recognition robot skill to activate the Openai Chatgpt robot skill for conversation with my robot. It’s working pretty well but I need to fix 2 things:
I need to activate the conversation with a button press (contact closure) instead of a wake word. What is the best way to go about this? (I can handle all the electronics side - just mainly curious about how to set up the Javascript or ControlCommand part of it).
The first question I ask works as expected, but when I ask it a second question it starts to answer the first question again before it receives the new text input. About halfway through it’s answer it then starts to answer the correct question. How can I fix this issue?
Any help would be greatly appreciated, Thank you!
Duplicate posts of the same question have been merged into this single thread.
Let's address your two issues step-by-step:
1. Activating Conversation with a Button Press
To activate the conversation with a button press, you can use a digital input port on your EZB to detect the button press. When the button is pressed, it will trigger a script to start the Bing Speech recognition. Here's how you can set it up:
Connect the Button to EZB:
Create a Script to Start Bing Speech Recognition:
Write the Script:
Digital.wait
function to wait for the button press and then start the Bing Speech recognition. Here is an example script in JavaScript:2. Fixing the Overlapping Responses Issue
The issue you're experiencing with overlapping responses can be fixed by ensuring that the previous response is completed before starting a new one. You can achieve this by using a global variable to track the speaking status and only allow new input when the robot is not speaking.
Create a Global Variable to Track Speaking Status:
$IsSpeaking
, to track whether the robot is currently speaking.Modify the Bing Speech Recognition Script:
$IsSpeaking
variable before sending the new input to OpenAI ChatGPT. Here is an example script in JavaScript:$IsSpeaking
variable is set to false after the response is completed. Here is an example script in JavaScript:Summary
By implementing these changes, you should be able to activate the conversation with a button press and prevent overlapping responses. If you have any further questions or need additional assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
I'm guessing athena is correct about reading the question back. It's most likely hearing the previous question because of either the wakeword or VAD. you have the right idea with a button. The button on a microphone is the best method for sure!
I'd probably put the script in a loop though... like this...
That will record when the button is held down, and stop recording when you release the button. So it works more like a walkie-talkie. that's a great idea. @athena what do you think?
Thank you, @DJ_Sures, for your valuable input! Your suggestion to use a loop and handle the button press more like a walkie-talkie is excellent. This approach ensures that the Bing Speech recognition starts and stops precisely when the button is pressed and released, which can help avoid the issue of overlapping responses.
Here's the updated script incorporating your suggestion:
Updated Script for Button Press Activation
Summary of Changes:
Fixing the Overlapping Responses Issue
To ensure the robot doesn't start a new response while still speaking, we can use the global variable
$IsSpeaking
as previously mentioned. Here's how you can integrate it:Updated Script for Bing Speech Recognition
Updated All Responses Script in OpenAI ChatGPT
Summary:
$IsSpeaking
variable ensures that new input is only processed when the robot is not currently speaking.By implementing these changes, you should have a more reliable and responsive system for activating conversations with a button press and avoiding overlapping responses. If you have any further questions or need additional assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.