Asked
— Edited
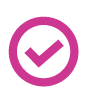
Hi all,
I'm trying to get serial feedback from a device. Does anyone know where on the V4 I should attach to read back serial information from device? I know I send a serial command to through one of V4's digital signal pins but where do I read it back from?
I thought it would be read from the same digital pin I sent the command. However @Toymaker made a comment in another thread that it may have to be read through one of the i2c ports.
Any insight is welcomed.
The V4 (like the V3) can only send serial on the digital pins... You'll need to use the V4's UART port for bi-directional serial transfer... I hope DJ puts up a tutorial soon on how to use the UART port...
I thought it would be read through on of V4 EZB's digital ports. I thought this because we send serial commands through the signal pin of a digital. Then Toymaker said it should read serial feedback from the i2c port. Now you say the UART port. confused I guess I need to get this cleared up.
Rich also mentioned using i2c... We all hoped for basic serial (send and receive) through the digital pins of the V4... To my knowledge you can still only send... DJ instead provided the v4 with UART ports... UART is what we can use for bi-directional serial data transfer... I myself am not quite sure how to use the UART port but devices like the iRobot Create and I am guessing the Kangaroo too should work with 2 way serial communication using this port... DJ said that tutorials on how to use the port are coming soon...
Looking in the "Learn" section in the Data Sheet for V4 I see the UART port. It shows a RX & TX connection along with a 3.3v and Gr. Looks like you may be right. Like you I'm unsure how to use this port. I guess I'll muck around in the script commands to see if I can come up with something.
Good thing the Kangaroo doesn't need power as the V3 only supplies 3.3 and not the 5v it will get from the Sabertooth.
That's the port Dave (Black port on the lower right of the board)... I am sure it will be straight forward to use... After all... It is made by EZ Robot, right?...
We (I am) are probably over thinking things here anyway...
Here's something else that confuses me. In the specs EZ Robot states:
3 x i2c Port and 3 x High Speed UART Ports. I see the i2c ports. Where are the UARTs? They must be hard coded and be accessed through that mystery connection I'm looking for. confused
@Dave - UART 1and 2 -
Hope this helps.
There are script commands for the UART port; UARTInt UARTAvailable UARTRead UARTWrite
Examples and help are in the EZ-Script manual to the right of the script dialogue. There is also a sample project in the examples folder of ARC which shows how to use it.