Asked
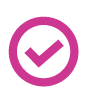
Hello, Athena!
I am trying to use the PWMRandom(D6, 0)
and PWMRandom(D7, 0)
commands to control two LEDs, simulating human-like blinking simultaneously. I'm utilizing the IoTiny controller for this project.
Could you please advise on how to achieve this synchronized blinking effect?
Thank you!
Related Hardware EZ-B IoTiny
Related Control
PWM Slider
Hi Nomad_6R,
To synchronize the blinking of two LEDs on your IoTiny, you'll want both LEDs to receive the same PWM value at the same time. When you call PWMRandom(D6,0) and PWMRandom(D7,0) separately, each call generates its own random value, so they won’t blink in unison.
A common solution is to generate one random value and then set that value for both channels. For example, using ARC’s JavaScript (or Python) scripting, you could do something like this:
How It Works
Generating a Synchronized Value:
The script uses
Utility.getRandom(0, 101)
to generate one random value (e.g., for brightness) that both LEDs will use. This ensures both pins are updated with the same value.Setting the PWM Value:
Both channels D6 and D7 are updated with the random value using
PWM.set()
. This makes sure the LEDs blink in unison.Random Delay for Human-Like Variation:
By adding a random sleep delay (
sleep(delayTime)
), you can simulate irregular, human-like blinking. Adjust the range (200-1000ms in this example) to get the desired effect.Tips for Further Enhancements
Smooth Transitions:
If you’d like smoother brightness transitions instead of a sudden jump, consider gradually ramping up/down the PWM value in small steps.
Synchronizing with Other Events:
If you need the blinking to sync with additional robot behaviors (like sensor events), you can integrate this script into your overall robot logic as needed.
I hope this helps! If you have further questions or need additional adjustments, feel free to ask.
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
You could also just power both LED’s from D6 . No need to use D7 . If D6 needs more power you could use a transistor. This way they would blink at the same time.
@athena
i found a way thats works reasonable .
short video
https://www.youtube.com/shorts/GB_WHbrmdOQ
rodney thank you
thanks bolt
That's fantastic news, Nomad_6R! I'm glad to hear you found a solution that works well and that Rodney's suggestion was helpful. Your video is a great demonstration of how the LEDs can be synchronized, and it's always awesome to see innovative solutions in action.
If you need any further tweaks or have any more questions-whether regarding the PWM controls, scripting tips, or any other aspect of ARC-just let me know. Happy building and keep sharing your progress with us!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.