
aume
Australia
Asked
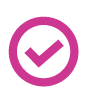
I started working with a JD robot a couple of days ago. I am looking to create fluid motion from pose to pose.
I am programming the motion via the Script Collection (Python). This is resulting in a relatively smooth motion at a constant speed, but I would like to create acceleration or motion profiles to make the motion look more lifelike. Below is my python code to wave
shoulder = 3
bicep = 4
elbow = 5
grip = 6
def moveServo(port, pos, speed=0):
Servo.SetSpeed(port, speed)
Servo.SetPosition(port, pos)
def wave(iter=0):
moveServo(elbow, 50, 1)
moveServo(grip, 70, 1)
moveServo(bicep, 70 if iter == 0 else 110, 3)
sleep(400)
moveServo(elbow, 0, 1)
moveServo(grip, 90, 1)
moveServo(bicep, 90, 3)
sleep(400)
def armhome():
moveServo(elbow, 90, 2)
moveServo(bicep, 20, 2)
moveServo(grip, 90, 2)
moveServo(shoulder, 90, 2)
moveServo(shoulder, 0)
moveServo(elbow, 0)
moveServo(grip, 90)
moveServo(bicep, 90)
sleep(1000)
wave()
wave(1)
sleep(1000)
armhome()
sleep(3000)
I have also messed around with creating motion in a "Robot Skill" via a c# workflow. I've noticed the motion from servos in this is very jerk though. Below is a snippet of my code from that:
private void button1_Click(object sender, EventArgs e)
{
ARC.LogManager.Log("clicked the button!");
ARC.EZBManager.EZBs[0].Servo.SetServoPosition(EZ_B.Servo.ServoPortEnum.D0, 10);
Thread.Sleep(1000);
ARC.EZBManager.EZBs[0].Servo.SetServoPosition(EZ_B.Servo.ServoPortEnum.D0, 170);
}
My questions are:
- Can you adjust the speed of the servos with numbers with decimals in either python or c#? i.e. 1.5 speed instead of 1 or 2?
- Is there a better way to control servos in C# such that the motion will be more smooth?
- Are there some other ways to control the servos which may result in more fluid, life-like motion that I haven't thought of?
Thank you in advance.
Related Hardware JD Humanoid
Related Control
Servo Speed
The Auto Position is created for this purpose. Check out the manual for it here: https://synthiam.com/Support/Skills/Movement-Panels/Auto-Positioner-Gait?id=16057
Essentially, the Auto Position allows you to create animations that can be executed programmatically. There's more detail about it on the Auto Position manual page.
Thank you for the help! This works