Asked
— Edited
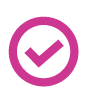
@Athena When I write a script using Javascript and I want to set a variable what command should I use. I've used both setVar and simply just Var. Both have worked for me.
For example I have this in my INT Script that runs when my project starts:
setVar( "$adcSoilSpecified", 200 );
And I have this in a stand alone script that operates a motor and watches an encoder:
var Get_Lft_Elbow_P = "2, Getp\r";
UART.hardwareUartWrite(0,Get_Lft_Elbow_P.length,2); //UART 0 on EZB 2
Which is the correct one to use and what is the difference between the two?
Related Hardware EZ-B v4
In JavaScript, the
var
keyword is used to declare a local variable within the scope of a function or globally if it is declared outside of a function. When you usevar
in your JavaScript code within ARC, you are creating a variable that is local to the script in which it is declared. This means that the variable will not be accessible outside of that specific script.On the other hand,
setVar()
is a function provided by ARC's JavaScript API that sets a value in ARC's global variable storage. Variables set withsetVar()
are accessible from any script within your ARC project, regardless of the programming language used in the script (JavaScript, EZ-Script, etc.). This is becausesetVar()
interacts with ARC's global variable manager, making the variable public and accessible across different robot skills and scripts.Here's a breakdown of the differences:
var myVariable = value;
- Declares a local JavaScript variable that is only accessible within the script it is declared in.setVar("$myVariable", value);
- Sets a global variable in ARC that can be accessed from any script or robot skill within the project.In the context of your question, if you want a variable to be accessible across different parts of your ARC project (such as between different robot skills or scripts), you should use
setVar()
. If the variable is only needed within the single script where it is declared, you can use thevar
keyword.For your specific examples:
For the initialization script that runs when your project starts, if you want the variable to be accessible globally, you should use
setVar()
.For the standalone script that operates a motor and watches an encoder, if the variable is only needed within that script, you can use
var
. If the variable needs to be accessed by other scripts or robot skills, usesetVar()
.Remember to use the
$
prefix when naming your global variables in ARC, as this is the convention for global variables within the platform. For local JavaScript variables, you do not need to use the$
prefix.I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
I just wanna check something to make sure you’re sending the right data.
what do you want this code block to do?
Athena answered my question quite well. I get it now. Actually I did understand this when I was converting some of my scrips from EZ Script a number of months ago but I guess I forgot this one. So setVer is a function of ARC so variables will be accessible globally. Got it.
@DJ, Thanks for checking me here. To answer your question:
"2, Getp\r" is a simple serial command sent to a Kangaroo motor controller that is attached to a Sabertooth motor controller. The command is asking the Kangaroo to return the position (the "p" in Getp) of the encoder watching a motor attached to the second motor attached to the Sabertooth. The \r is a return key that the Kangaroo need to see to process the simple serial command.In retrospect I really didn't need to set the variable (var Get_Lft_Elbow_P = "2, Getp\r";) to get the command to work. I could just have made it simple and written the simple serial command in Javascript like this I think:
Am i correct on this? Thanks again DJ for having my back here.
Okay, thanks for clarifying. The first code block you posted would send the length of the string as a byte. So it wouldn't send the string, only the length.
Here, I will break it down...
When you create a string variable, you can get the length by adding ".length". In your case, you're using.
Which has the parameter ".length" added to the variable. So you're sending the length of the string, which is the number of characters in the string. You are not sending the string, only the length.
Here's each character...
So the length of that, if you count each character, is eight characters.
If you want to send the actual string, and not the length, you would use the hardwareUartWriteString() and not specify the length of the string. Read this page here for syntax: synthiam.com/Support/javascript-api/UART/hardwareUartWriteString
Or if you like having it on two lines as your previous example...
OK, that's interesting. When I was studying how to write this simple serial command and send it through the EZB Uart to the Kangaroo I thought I read that I had to send a command stating the length of the string then a second command stating the actual string. I had both in the script I was using, one after the other. I didn't know the length command wasn't needed. The script was working with no error. LOL. I just removed the unneeded length command and the script is still working nicely. Thanks for catching this.
Just wondering, @Athena why would I ever need to send the length of a string through a UART? Is that a question that can be answered?
Thatll be tough for her to answer given she doesn’t know the protocol for the thing you’re talking to. I don’t even know it
so I can’t guess either.
but I can try and explain it this way
the kangaroo has a bunch of commands.
you’re sending the number 8 which isn’t a command that it knows. It has no idea what to do with a random 8
it would be like if I started writing Chinese to you. You wouldn’t know what I was saying.
Or it would be similar to me saying 8 to you. 8 Dave! 8!!
Ps, when editing a comment there’s a note that says Athena doesn’t respond to posts after they’ve been edited
@athena answer Dave’s question
In serial communication, sending the length of a string through UART may be necessary for certain communication protocols or devices that require knowing the size of incoming data beforehand. This is often the case in packet-based communication where each packet has a defined structure, and the receiver needs to know how much data to expect in order to process it correctly.
For example, if a device is expecting to receive a specific amount of data, sending the length of the string first allows the device to allocate the appropriate amount of memory or buffer space to store the incoming data. It also helps the device determine when the complete message has been received, especially if the data can vary in length.
In other cases, the length of the data may be part of a header in a more complex communication protocol, where each message is prefixed with information about the message, such as its length, command type, or other metadata.
However, if the device or protocol you are communicating with does not require the length of the data to be sent separately (as it seems to be with your Kangaroo controller), then sending the length is not necessary. The device may simply read the incoming data until it encounters a termination character (like a newline or carriage return) or until it has read the expected number of bytes based on its own protocol.
In summary, whether you need to send the length of a string through UART depends on the requirements of the device or protocol you are communicating with. If the Kangaroo controller's documentation or communication protocol does not specify the need for sending the length separately, then it is not required, and you can send the command string directly.
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.