
smondal
Australia
Asked
— Edited
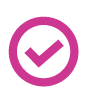
Hi I am trying to write a code for JD Humanoid where I can track the data coming from the servo. First I tried to write the following ode with EZ-Script. It serves my initial purpose but I also need a proper timestamp in "ddmmyyyyhhmmssffff" format. Apparently it seems that I can't do it with EZ-Script. So, I am thinking to write a similar code in Python as I can make the required timestamp formatting there. So, can anyone help me with this? Thanks.
ControlCommand("Graphy", RemoveAllSeries)
:loop
$pos0 = getservo(d0)
ControlCommand("Graphy", AddData, "Servo D0", $pos0)
$pos1 = getservo(d1)
ControlCommand("Graphy", AddData, "Servo D1", $pos1)
controlCommand("Camera", "CameraMovementTrackEnable")
ControlCommand("Graphy", AddData, "Servo D4", $pos)
FileWriteLine("c:\temp\mylog.txt", $time + ", servo D0: " + $pos0 + ", servo D1: " + $pos1)
sleep(10)
goto(loop)
Related Hardware JD Humanoid
Related Control
Graphy
Is there a reason for setting the movement tracking enabled for the camera every 10 ms in the loop? Or is it an accident? Because that's like bashing the mouse button on the option over and over and over and over and over. It'll use up a ton of cpu and slow down your computer. You only need to set it once. Set it outside the loop at the beginning of the program - just like you do when clearing the graph.
ez-script will only give you a second resolution. You can do it with this, but you only get 1 second resolution from the date object. It's documented in the ezscript manual when editing ezscript.
Is there a reason the date format absolutely needs to be ddmmyyyyhhmmssffff? Because you will have to use extra processing to ensure padding on single digit numbers (i.e. 5 must become 05). It would be ideal to separate each date value with a : like how clocks and such do. So it would be dd:mm:yyyy:hh:mm:ss:ffff OR just use a space between each one like other date parsers do. So it would be dd mm yyyy hh mm ss ffff
I'm sure JavaScript has a ToString() that might accept a formatting specification. So you can output the exact format you desire. I imagine it would be documented in the JavaScript manual from the link above
It appears Graphy has a SAVE TO CSV option as well...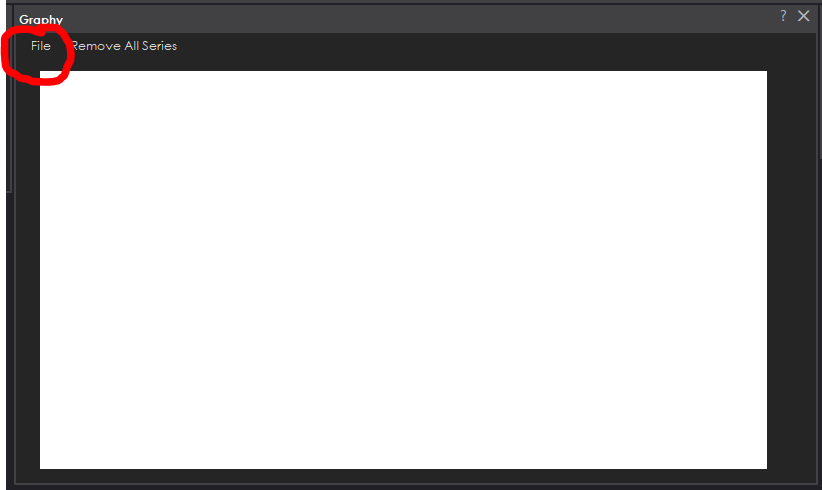
Oh - one more thing... a 10ms loop is considerably fast. Have you considered calculating an optimized loop delay? Or even keeping the last state of the previous servo positions in an array and only writing if a servo has actually changed position?
Something like... (ps, line 30 should use File.appendStringLine)
Thank you very much DJ Sures for your prompt response. I was not very sure about the delay but I needed it the range of a few milliseconds. So, I guess around 100 ms will also work for me. I'll try that. Also, thank you for pointing me to JavaScript as it has better documentation. I mainly need the hh:mm:ss:ffff type for saving the timestamps, because I want to observe the histogram of the inter-arrival times. This is why it is problematic so save directly as a CSV file from Graphy cannot log the timestamps.
Okay - tomorrow the web dev is going to fix the bug with posting code. That means I can repost that code with some improvements - specifically a sleep() in my loop would be useful. I accidentally left that out.
the code is a pasted image because of the web forum bug. Once it’s fixed I can repost the code for you to copy and paste
Oh I see.. that's really good news. Thanks a lot. Much appreciated for your help. Cheers!
With a slight modification to fix the single digit issue in printing timestamps. Thanks.
you'll benefit with a sleep(10) in there to be friendly on the cpu thread of the script
Here you go - this is a good script that will run faster than calling a user defined method every time for padding. There's only two things necessary to modify in this script.
Thanks a lot. Now I can extract the data just as I wanted!