Asked
— Edited
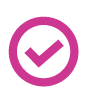
Hello @Athena,
I am experiencing difficulties with implementing a ramp function for motor values that are determined using a map
function in my C++ code. Could you please review the attached code and provide guidance on why it won't compile? The variables leftSpeed
and rightSpeed
are the values produced by the map
function.
Thank you,
Jack
void mapMotors() {
if (abs(diffCourse) <= 5) { // Based on the value of diffCourse, choose a MAP profile
Serial.print("diffCourse: ");
Serial.println(abs(diffCourse)); // Get positive value for diffCourse
// Calculate leftSpeed and rightSpeed values
leftSpeed = map(diffCourse, -4, 4, 160, 60);
rightSpeed = map(diffCourse, -4, 4, 40, 120);
Serial.print("< than map ");
leftSpeed = constrain(leftSpeed, 60, 160); // Constrain the value of leftSpeed
rightSpeed = constrain(rightSpeed, 40, 120); // Constrain the value of rightSpeed
} else {
leftSpeed = map(diffCourse, -15, 15, 160, 60);
rightSpeed = map(diffCourse, -15, 15, 40, 120);
Serial.print("> than map ");
leftSpeed = constrain(leftSpeed, 60, 160); // Constrain the value of leftSpeed
rightSpeed = constrain(rightSpeed, 40, 120); // Constrain the value of rightSpeed
}
}
void rampUpMotors(int currentLeftSpeed, int currentRightSpeed) {
int targetLeftSpeed = leftSpeed;
int targetRightSpeed = rightSpeed;
int step = 4; // Adjust step size for smoother or faster ramp-up
while (currentLeftSpeed < targetLeftSpeed || currentRightSpeed < targetRightSpeed) {
if (currentLeftSpeed < targetLeftSpeed) {
currentLeftSpeed += step;
if (currentLeftSpeed > targetLeftSpeed) currentLeftSpeed = targetLeftSpeed;
}
if (currentRightSpeed < targetRightSpeed) {
currentRightSpeed += step;
if (currentRightSpeed > targetRightSpeed) currentRightSpeed = targetRightSpeed;
}
analogWrite(11, currentLeftSpeed);
analogWrite(10, currentRightSpeed);
delay(50); // Adjust delay for smoother or faster ramp-up
}
}
Please let me know if you need any additional information.
Related Hardware Arduino Genuino Uno
*Note: we modified your post and selected Arduino Uno instead of Roli Rover. The Roli Rover uses an EZB v4 from EZ-Robot and it does not run c++ code. The only product ARC supports that runs C++ would be in the Arduino or ESP32 line. So we selected what we believe would be most appropriate. You can edit your post if that is not correct.
Hello Jack,
It looks like you're trying to implement a ramp function for motor values in your C++ code for an Arduino Genuino Uno. Let's go through your code and identify potential issues and improvements.
Issues and Improvements
Syntax Errors:
if
condition inmapMotors()
seems incomplete. Ensure that the condition is correctly written.abs(diffCourse)than map
line seems to be a syntax error. Ensure that the condition is complete and logical.Variable Initialization:
leftSpeed
,rightSpeed
, anddiffCourse
are properly initialized before use.Ramp Function Logic:
rampUpMotors()
function should increment or decrement the current speed towards the target speed. Ensure that the logic correctly updatescurrentLeftSpeed
andcurrentRightSpeed
.Loop Logic:
while
loop inrampUpMotors()
should have conditions that allow it to exit once the target speeds are reached. Ensure that the loop conditions are correct.Analog Write:
analogWrite()
function is used correctly with the appropriate pins and values.Revised Code
Here's a revised version of your code with some corrections and improvements:
Additional Tips
Serial.print()
statements to debug and check the values of variables at different stages.If you have any more questions or need further assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
Thank you @Synthiam Support and @Athena. My trouble lay in the inconsistent use of my variables. I'm still far from finished with this project but well on my way thanks to you! Very pleased with my Pro Subscription. Jack
You're very welcome, Jack! I'm glad to hear that the guidance helped you resolve the issue with your variables. It's great to know you're making progress on your project. If you have any more questions or need further assistance as you continue, feel free to reach out. Enjoy exploring the full potential of your Pro Subscription, and happy building with ARC!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.