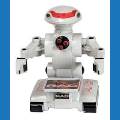
rz90208
Creating A Simple Robot Brain
Building on Justin's thread on creating a brain. There are many ways to create artificial intelligence and this is just what I have come up with using the tools ARC has currently available.
I have designed my project to be Service/Agent based. When the Robot is powered up the connection script launches the init script.
ControlCommand("Script Manager", ScriptStart, "Init")
This initializes all variables and launches the Voice Service and the Auditory Service and unpauses the Speech Recognition plugin. The only Speech Recognition phrase I have entered here is the robot's name and the is UNAS (meaning First One) Stargate SG1 fans will have known that. And that script looks like this:
ControlCommand("Speech Recognition", PauseOn)
ControlCommand("Bing Speech Recognition", StartListening)
As you can see when the Robot hears his name "UNAS", it pauses the speech recognition module and starts Bing Speech listening.
Here is the Bing Speech Script
ControlCommand("Bing Speech Recognition", StopListening)
WaitFor($IsListening = 1)
ControlCommand("Speech Recognition", PauseOff)
As again you can see after the speech has been captured the script stops Bing speech listening and unPauses Speech Recognition.
The WaitFor($IsListening = 1) we will see later in other scripts for now we can just ignore that line.
Now to the fun part:
The Auditory Service's first line is waiting for the $BingSpeech variable to change and when it does that is when the magic happens.
:AuditoryService
#The Auditory service monitors the $BingSpeech variable for speech and then routes this speech to
#the proper Agent for processing.
#
#***************************************
WaitforChange($BingSpeech)
$HumanSaid = $BingSpeech
$BingSpeech = ""
$IsListening = 0
#***************************************
#Convert the first letter of the captured speech to lower case
$Trash = $HumanSaid
ControlCommand("Agents",ScriptStartWait,"ConvertToLowercase")
$HumanSaid = $Trash
#***************************************
When the $BingSpeech variable changes, its contents are saved in the $HumanSaid variable and the $BingSpeech variable is cleared. Here is where we see the $IsListening variable set to 0 letting the other scripts know that the robot is currently processing and not listening.
The next few lines converts the first letter of the spoken speech to lowercase, you will see why later.
Next the script goes thru a bunch of if statements.
#BlankCanBlank
if(Contains($HumanSaid," can ") & !Contains($HumanSaid,"What can "))
ControlCommand("Agents",ScriptStartWait,"BlankCanBlank")
#CanBlankBlank
elseif(Contains($HumanSaid,"can ") & !Contains($HumanSaid,"What can ") & !Contains($HumanSaid," can "))
ControlCommand("Agents",ScriptStartWait,"CanBlankBlank")
#BlankHaveBlank
elseif(Contains($HumanSaid,"have") & !Contains($HumanSaid,"Do "))
ControlCommand("Agents",ScriptStartWait,"BlankHaveBlank")
#BlankIsInBlank
elseif(Contains($HumanSaid,"is in"))
ControlCommand("Agents",ScriptStartWait,"BlankIsInBlank")
#BlankIsThePluralOfBlank
elseif(Contains($HumanSaid,"is the plural of") & !Contains($HumanSaid,"what "))
ControlCommand("Agents",ScriptStartWait,"BlankIsThePluralOfBlank")
I did not include the entire script just enough for a good example So the if statements look at the speech phrase for specific words in specific orders and calls the corresponding Agent. For example we will use the following speech phrase "dogs can run" The if statements will match this and call the CanBlankBlank Agent
:BlankCanBlank
$IsListening = 0
$Junction = "can"
$SpeechLength = Length($HumanSaid)
$JuncIndex = IndexOf($HumanSaid,$Junction)
$FirstPart = SubString($HumanSaid,0,$JuncIndex-1)
$LastPart = SubString($HumanSaid, $JuncIndex + Length($Junction) + 1,$SpeechLength - ($JuncIndex + Length($Junction))-1)
$SearchPhrase = $FirstPart + "_:::_" + $LastPart
$MemoryType = "BlankCanBlank"
ControlCommand("Agents",ScriptStartWait,"WriteMemory")
if($WriteMemoryCheck = 0)
$SayWhat = "I knew that!"
else
$SayWhat = "I now know that " + $FirstPart + " " + $Junction + " " + $LastPart
endif
$IsListening = 1
The Agent Parses the captured speech takes what is before the word can and stores that in $FirstPart and what comes after the word can and stores that in the variable $LastPart Then loads the variable $SearchPhrase and $MemoryType then calls the Write Memory Agent.
This Agent opens the file and searches the file for the SearchPhrase. I will go into detail of the read and write memory Agents in the next exciting episode of Creating a Simple Brain.
I've been using the one word speech recognition engine with ARC to start the Bing speech recognition engine ever since it became available to us. I can confirm that it is a wonderful way to have your robot start listening to you. For me it completely removed any false positive responses from my robot. I'm also sure that it's Cuts way down on unneeded process time from my robot. Bing is no longer always listening for something. It only starts when my speech recognition engine tells it to. It totally resembles Amazon's Alexa. Only difference is I set the wake up word. In my case it's "Robot".
This is a brilliant tutorial. Thanks for putting it together. It's a real fun read. Can't wait for your next excitement filled episode. Same Bat time! Same Bat Channel! eek lol
Episode 2 - Read / write files:
At the end of episode 1, we had just stored in $SearchPhrase dogs_:::_run and in $MemoryType we stored BlankCanBlank and called the WriteMemory Agent
First I set the file name. I put the x in the string to get around the problem of the backslash. As you remember we stored BlankCanBlank in $MemoryType so this will be our actual file name, plus the x of course (xBlankCanBlank.txt). In The ReadMemory Agent I have created, I believe at this time there is 4 read types
1 = compare complete memory line with $SearchPhrase
2 = Compare first part with $SearchPhrase and return first match
3 = Compare First Part with search phrase and return all that match
4 = Compare last part with search phrase and return all that match
Results are returned in an array $ReadResultsArray[x]
So we set the ReadType to 1, because we want to compare with the entire memory line. We will go deeper into the ReadMemory Agent shortly for now all we need to know is that the read memory agent sets the variable $ReadResultsCount = 0 if no entry is found. I then reset the file read to the beginning of the file and loop thru every line until I reach the end of the file. I then Write the line dogs_:::_run to the file Set the $WriteMemoryCheck flag to 1 and return to the calling script.
The if statement checks the WriteMemory flag to see if a new memory was written and if so, stores I knew that in the variable $SayWhat In eposode 1 one of the services that init started was the Voice Service.
A simple few lines of code but vary powerful as all I need to do to have the robot speek is put the words I want spoken into the $SayWhat variable and done. Another thing I should mention about this routing is the $IsTalking variable. If I have a few lines of speech I want the robot to speak this code whould not work.
you would get the first line and that would be it. The rest would go unspoken because the script did not wait until each line was done before sending the next. But this code works perfect.
Now lets go back to that ReadMemory Agent This script is a little bigger.
For this example I am only going to go thru the read type 1. If you recall the read memory agent was called for the write memory agent to check if a specific memory exists. The first thing I do is define the delimiter sence this never changes I might move this to the init script but and save me one line of code here and there. My thinking was mabe later I might want to use different delimiters, for what I dont know. back to the script. Set my delimiter, set my file path and reset the file to the begining. Now using an if statement goto the proper read routine in this case read type 1 Read a line from the file and because the if statement is case sensitive on its compare, I convert the first letter of the memory to lowercase (if its not already). I then build the entire memory just as it is stored in the file. In the actual read memory routine, it breaks it apart. This is so I can support multiple read types. Now that we have built our memory just as it is stored in the file, I compare it to the $SearchPhrase we are looking for. If the compairison fails, read the next line until we reach the end of the file. If the compare finds a match, we set the ReadResultsCount flag and return to the calling routine (WriteMemory). If we do not find a match $readResultsCount remains 0 and we again return to the calling routine. This covers the basic routines we need for our brain. So in the next episode we will expand on what we have so far. For those of you that are coping the code for study or use, here is the full ReadMemory Agent code. Remember this is a work in progress and not might change but will change in the future. But my plan is to update this thread when I make changes. So stay tuned !
Here is also the Convert the first letter to lowercase routine.
@rz90208, Wow, thanks for committing so much time doing this.
I'm lost, but my programming skill is in comparison to a 1st grader.
Examples like this help me a lot. My favourite way to learn.
@rz90208,
Thanks so much for putting this together. Very impressive, and will be a big help to a lot of users.
Alan
You are welcome, I enjoyed put it together. I will continue to post as the simple brain evolves.
Episode 3 - Creating our Memory Agents and Memory Files.
Now that we have our Voice Service, Auditory Service and our Read and write Agents, we can make a few memory agent templates to make the creation of the agents much easier. Lets start out with the getting the robot to understand "dogs can run" If you recall from episode 1 the robot or brain was able to understand "dogs can run"
Going thru this script line by line we first see that we set the IsListening flag = 0 so the robot will not listen while it is processing the current speech. Next to set what I call the Junction. This is the word or phrase that separates the subject from the object, in this case the word "can". We then Find the total spoken speech length and store this in the variable $SpeechLength. Next we need to know where in the speech string the junction is located. So for this we use the IndexOf function. Instead of using the variable $Junction I could have used "can", but then the script would not be as easily changed later when we want to reuse it for another Memory Agent. Now to get the first part.To do this we use the SubString function. The $HumanSaid is the source string to search, the zero is where to start and the JuncIndex -1 is the number of characters. The reason for the -1 is because we do not want the space between the first part and the word can. To get the last part we start at the junction index instead of 0. But this will give us the word "can" also, so we move our start point ahead the length of the junction, and don't forget the space. Now to calculate the remaining characters. To do this we just take how many characters were in the entire string, subtract all the characters up to the junction, plus the length of the junction, oh and again don't forget the space and what is left is our total remaining characters. The script now calls the WriteMemory Agent that we went over before. Now to change this script into a BlankHaveBlank script, all we need to do is: Change the first line of the script from :BlankCanBlank to BlankHaveBlank, change out $Junction assignment from "can" to "have", change our $MemoryType from "BlankCanBlank" to "BlankHaveBlank".
And now we have a new Agent.
I think I failed to mention that at this point I am manually creating the text files initially. After that the scripts read and write to them. So in your file path create a file for each memory type: xBlankHaveBlank, xBlankHaveBlank. You dont have to create a file for CanBlankBlank or DoBlankHaveBlank because they will search the other files for the answer.
I am currently working on possibly adding a ! to the memory for an entry such as dog can not fly - dos_:::_!fly. This way if an entry was not present the robot could say "I don't know" instead of "no".
Well that's all for this post.
Please fell free to post comments, questions or recommendations that might improve the project.
List of Agents I currently have completed.
BlankLikeBlank HowManyBlankDoBlankHave DoBlankHaveBlank BlankLikeBlank CanBlankBlank BlankIsPluralOfBlank BlankIsInBlank IsBlankBlank BlankIsBlank BlankHaveBlank BlankCanBlank
If anyone would like me to post the code just let me know.
I would certainly like you to post the code!