
aivanouski
Indonesia
Asked
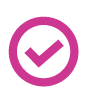
Hi , I'm trying to play mp3 from robo head:
try
{
using (NAudio.Wave.Mp3FileReader mp3 = new NAudio.Wave.Mp3FileReader(filePath))
{
using (NAudio.Wave.WaveFormatConversionStream pcm = new NAudio.Wave.WaveFormatConversionStream(new NAudio.Wave.WaveFormat(EZ_B.EZBv4Sound.AUDIO_SAMPLE_BITRATE, 8, 1), mp3))
{
using (MemoryStream ms = new MemoryStream())
{
using (GZipStream gz = new GZipStream(ms, CompressionMode.Compress))
EZBManager.EZBs[0].SoundV4.PlayData(gz);
}
}
}
}
NAudio was addeg with nuget package manager, but it provides error:
Visual Studio Community Edition 2019Quote:
Could not load file or assembly 'NAudio, Version=2.2.1.0, Culture=neutral, PublicKeyToken=e279aa5131008a41' or one of its dependencies. The located assembly's manifest definition does not match the assembly reference. (Exception from HRESULT: 0x80131040)
How to create plugin with NAudio or play mp3 from robohead?Quote:
package id="NAudio" version="2.2.1" targetFramework="net48"
Related Hardware EZ-InMoov Robot Head
To play MP3 files from your EZ-InMoov Robot Head and resolve the "Could not load file or assembly NAudio" error, you can follow these steps. It seems like you're trying to create a plugin or script that utilizes NAudio for audio playback within the Synthiam ARC environment. Here's a general approach to troubleshoot and achieve playing MP3 files:
1. Ensure NAudio is Correctly Installed
First, make sure that NAudio is correctly installed in your project. Since you've mentioned using NuGet Package Manager, you can verify the installation by checking the
packages.config
file in your project or looking at the References list in the Solution Explorer to see if NAudio is listed.2. Check for Assembly Binding Issues
The error "Could not load file or assembly" often indicates a version mismatch or that the assembly cannot be found. You can try the following:
3. Using NAudio to Play MP3
Here's a simple example of how to use NAudio to play an MP3 file. Ensure you have NAudio installed and then use the following code snippet:
4. Integrating with Synthiam ARC
If you're creating a plugin for Synthiam ARC, you'll need to ensure that your plugin correctly references and packages NAudio. When you build your plugin, make sure the NAudio DLL is included in the output directory or packaged with your plugin.
5. Alternative: Using Synthiam ARC's Built-in Audio Features
Synthiam ARC has built-in support for playing audio files. If you're looking to play MP3 files as part of a robot action or behavior, you might not need to use NAudio directly. Instead, you can leverage ARC's audio playback capabilities through its scripting or graphical interface.
Troubleshooting
By following these steps, you should be able to resolve the assembly loading issue and play MP3 files from your EZ-InMoov Robot Head using NAudio or Synthiam ARC's built-in features.
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
All the libraries you need for the project are located in the ARC installation folder.
it’s c:\program files (86\arc by synthiam or something like that
Oh and just make sure you mark the resource libraries to not copy as a build action
Thank you, DJ Sures!
.dll from
Works.