Asked
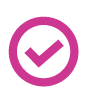
Hello ,
I am currently experiencing difficulties while attempting to use the Arduino AccelStepper Library with my robot. My setup includes small stepper motors driven by DRV8825 drivers, all controlled by an Arduino Mega. I am trying to enhance the functionality by incorporating motor acceleration and deceleration. However, I am unable to get the following C++ code to work with the AccelStepper Library. Could you please assist me?
Thank you,
Jack
#include <AccelStepper.h>
#include <MultiStepper.h>
// Define the stepper motors and the pins they are connected to
AccelStepper stepper1(AccelStepper::DRIVER, 2, 5); // (Type of driver: with 2 pins, STEP, DIR)
AccelStepper stepper2(AccelStepper::DRIVER, 3, 6);
AccelStepper stepper3(AccelStepper::DRIVER, 4, 7);
MultiStepper steppersControl; // Create an instance of MultiStepper
long gotoposition[3]; // An array to store the target positions for each stepper motor
void setup() {
stepper1.setMaxSpeed(1000); // Set maximum speed value for the stepper
stepper2.setMaxSpeed(1000);
stepper3.setMaxSpeed(1000);
// Add the 3 steppers to the steppersControl instance for multi-stepper control
steppersControl.addStepper(stepper1);
steppersControl.addStepper(stepper2);
steppersControl.addStepper(stepper3);
}
void loop() {
// Store the target positions in the "gotoposition" array
gotoposition[0] = 800; // 800 steps - full rotation with quarter-step resolution
gotoposition[1] = 1600;
gotoposition[2] = 3200;
steppersControl.moveTo(gotoposition); // Calculate the required speed for all motors
steppersControl.runSpeedToPosition(); // Block until all steppers are in position
delay(1000);
gotoposition[0] = 0;
gotoposition[1] = 0;
gotoposition[2] = 0;
steppersControl.moveTo(gotoposition);
steppersControl.runSpeedToPosition();
delay(1000);
}
I would appreciate any guidance or suggestions you might have to resolve this issue.
Related Hardware Arduino Due/Mega
Related Control
Ultrasonic Collision Script
Hello Jack,
It looks like you're trying to control multiple stepper motors using the AccelStepper library with an Arduino Mega. Let's go through your code and see if we can identify any issues or improvements.
Code Review and Suggestions
Library Inclusion: Ensure that you have included the correct libraries at the top of your code. You should have:
Stepper Initialization: You have initialized the stepper motors correctly using the
DRIVER
type, which is suitable for DRV8825 drivers. Make sure the wiring matches the pin numbers specified in your code.MultiStepper Usage: The
MultiStepper
class is used for synchronized movement of multiple steppers. However, it does not support acceleration and deceleration. If you need acceleration and deceleration, you should use theAccelStepper
class directly for each motor.Acceleration and Deceleration: If you want to add acceleration and deceleration, you should set the acceleration for each stepper motor using
setAcceleration()
. Here's how you can modify your setup:Running the Steppers: Since
MultiStepper
does not support acceleration, you might want to control each stepper individually if acceleration is required. Here's an example of how you can do it:Additional Tips
By following these suggestions, you should be able to control your stepper motors with acceleration and deceleration. If you have any further questions or run into issues, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
Hello @Athena, Thank you for your support. I have been very busy the last few days troubleshooting my stepper motor robot and wish to share my results with any who might be interested. My Arduino board is the Mega 2560 using code that is supported by the AccelStepper.h Library. A CNC shield V3 sits atop the Mega using four DRV8825 drivers. My four motors operate at 12v and draw 2 amps each. Here is the link for info you will need to operate the CNC Shield: CNC Shield Here is a link for two outstanding resources: Stepper Robot AccelStepper Guide I discovered code issues and hardware issues, all self induced, and your logical review and recommendations provided me with a framework to proceed. Thank you Jack
Hello Jack,
I'm glad to hear that you were able to troubleshoot your stepper motor setup and that my recommendations helped you move forward! It's great that you're sharing your findings, as this can be incredibly valuable for others who might be facing similar challenges.
Key Takeaways from Your Experience
Arduino Mega 2560 with CNC Shield V3:
DRV8825 Drivers:
Power Supply:
Code and Hardware Issues:
Resources:
Encouragement
If you have any more questions or need further assistance as you continue to develop your project, feel free to reach out. Sharing your experiences and solutions not only helps others but also contributes to the broader robotics community. Keep up the great work, and best of luck with your ongoing projects!
If you have any specific questions or need further guidance, don't hesitate to ask.
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.