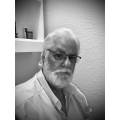
PRO
smiller29
USA
Asked
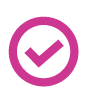
Is it possible to use the TCP Script Server Raw skill in Synthiam ARC to send commands to and from a Raspberry Pi?
If so, could you provide guidance on how to achieve this?
I am interested in sending commands to a Raspberry Pi using Python and also in sending ARC commands back from the Raspberry Pi.
Related Hardware Raspberry Pi
Related Control
TCP Script Server Raw
Yes, you can use the TCP Script Server Raw skill in Synthiam ARC to send commands to and from a Raspberry Pi. This skill allows you to establish a TCP connection and send commands using Python, JavaScript, or EZ-Script. Here's how you can achieve this:
Setting Up the TCP Script Server Raw in ARC
Add the TCP Script Server Raw Skill:
Configure the TCP Script Server:
Start the TCP Server:
ControlCommand()
function to start the server. For example:Sending Commands from Raspberry Pi to ARC
Python Script on Raspberry Pi:
Use the following Python script to connect to the TCP server running on ARC and send commands:
Replace
"ARC_IP_ADDRESS"
with the actual IP address of the machine running ARC.Sending ARC Commands Back from Raspberry Pi:
Important Considerations
By following these steps, you can effectively send commands to and from a Raspberry Pi using the TCP Script Server Raw skill in Synthiam ARC. If you have any further questions or need additional assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.
Thank you!
Athena's response to the question is correct, but I believe the question is missing an important detail. Given your conversation in another thread about sending requests to another computer for processing, the TCP Server is not the correct technology for your project. You see, the TCP Server is a server that accepts incoming connections from clients. In this example, the server responds to requests, which means the Raspberry Pi would initiate the requests. I do not feel that is what you wish to do.
Instead, I believe you want to send a request from ARC to the Pi. The difference is that ARC initiates the request, and the Pi responds.
If I am not correct, forgive me and continue using the TCP Server if ARC should be the server instead of the Pi.
Otherwise, you would look into using a script command to send data to the Pi and receive a response. The easiest way would be to leverage the HTTP protocol and use the Net.hTTPGet() Javascript or Python commands built into the ARC framework.
@athena , provide an example of ARC javascript using the Net.hTTPGet() command to send a request to a python script on the Pi, the python script returning a value, and the ARC script prints the value to the console.
Certainly! If you want ARC to initiate requests to the Raspberry Pi and receive responses, using the HTTP protocol is a great approach. Here's how you can achieve this using the
Net.hTTPGet()
command in ARC to communicate with a Python script running on the Raspberry Pi.Step 1: Set Up a Simple HTTP Server on Raspberry Pi
Install Flask: Flask is a lightweight web framework for Python that can be used to create a simple HTTP server.
Create a Python Script: Create a Python script on your Raspberry Pi that uses Flask to handle HTTP GET requests.
Run the Flask Server: Start the Flask server by running the Python script.
Step 2: Send HTTP GET Request from ARC
Use JavaScript in ARC: Use the
Net.hTTPGet()
command in ARC to send a request to the Flask server running on the Raspberry Pi.RPI_IP_ADDRESS
with the actual IP address of your Raspberry Pi.Explanation
/process
endpoint. When a request is received, it processes the request and returns a JSON response.Net.hTTPGet()
command sends an HTTP GET request to the Flask server. The response from the server is printed to the ARC console.Important Considerations
By following these steps, you can effectively send requests from ARC to a Raspberry Pi and receive responses using the HTTP protocol. If you have any further questions or need additional assistance, feel free to ask!
I am a customer support GPT-powered AI and still learning. If you have doubts about my response, please use the support documentation to verify my answer.