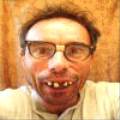
Herr Ball
Need some help with a little programming problem. Have given it my best shot but just can't seem to figure it out! My basic logic class is not enough for me and Google just got me lost TOO many times... LOL.
This program will be in a microcontroller that controls two 8x8(64) LED matrix for my robot eyes. The EZB will send it the serial commands to control the eye movements.
A little about the code below. I didn't post the entire code as I thought it really wasn't needed. The eyes have a neutral position, blinking, up, down, right, etc, etc. When EZB is started it will also start the microcontroller. I have been able to send serial commands from EZB to the controller, that works great. I can do this so far but not all together.
Problem that I'm lost at? How do I get the program to loop through "void scene"... take in any serial "incomingByte", which in turn does that "void" (ex. void down())... and then returns to "void scene" to continue.
Hope I explain this right and isn't confusing ... LOL.
Any help would be appreciated.
Herr
#include "LedControl.h"
LedControl lc=LedControl(12,11,10,2);
#include
int incomingByte; // a variable to read incoming serial data
void setup() {
// initialize serial communication:
Serial.begin(9600);
lc.shutdown(0,false);
lc.setIntensity(0,1);
lc.clearDisplay(0);
lc.shutdown(1,false);
lc.setIntensity(1,1);
lc.clearDisplay(1);
}
void loop() {
intake();
}
void intake() {
// see if there's incoming serial data:
if (Serial.available() > 0) {
// read the oldest byte in the serial buffer:
incomingByte = Serial.read();
// if it's a capital S (ASCII 83), go to void scene:
if (incomingByte == 'S') scene();
}
// if it's a capital D (ASCII 68), go to void dizzy:
if (incomingByte == 'D') dizzy();
// if it's a capital V (ASCII 86), go to void suchen_V:
if (incomingByte == 'V') suchen_V();
// if it's a capital 1 (ASCII 49), go to void upward:
if (incomingByte == '1') upward();
// if it's a capital 2 (ASCII 50), go to void right_upward:
if (incomingByte == '2') right_upward();
// if it's a capital 3 (ASCII 51), go to void right:
if (incomingByte == '3') right();
// if it's a capital 4 (ASCII 52), go to void right_downward:
if (incomingByte == '4') right_downward();
// if it's a capital 5 (ASCII 53), go to void downward:
if (incomingByte == '5') downward();
// if it's a capital 6 (ASCII 54), go to void left_downward:
if (incomingByte == '6') left_downward();
// if it's a capital 7 (ASCII 55), go to void left:
if (incomingByte == '7') left();
// if it's a capital 8 (ASCII 56), go to void left_upward:
if (incomingByte == '8') left_upward();
}
void scene()
{
neutral();
delay(4000);
lc.clearDisplay(0);
blink_eyes();
delay(4000);
lc.clearDisplay(0);
neutral();
delay(3000);
lc.clearDisplay(0);
blink_eyes();
delay(4000);
lc.clearDisplay(0);
blink_eyes();
delay(4000);
lc.clearDisplay(0);
neutral();
delay(3000);
lc.clearDisplay(0);
blink_eyes();
delay(4000);
lc.clearDisplay(0);
blink_eyes();
delay(4000);
lc.clearDisplay(0);
neutral();
delay(3000);
lc.clearDisplay(0);
blink_eyes();
delay(4000);
lc.clearDisplay(0);
blink_eyes();
delay(4000);
lc.clearDisplay(0);
neutral();
delay(3000);
lc.clearDisplay(0);
blink_eyes();
delay(4000);
lc.clearDisplay(0);
}
void neutral()
{
// Code goes here.
}
void blink_eyes()
{
// Code goes here.
}
void dizzy()
{
// Code goes here.
}
void downward()
{
// Code goes here.
}
void left()
{
// Code goes here.
}
void left_downward()
{
// Code goes here.
}
void left_upward()
{
// Code goes here.
}
void right()
{
// Code goes here.
}
void right_downward()
{
// Code goes here.
}
void right_upward()
{
// Code goes here.
}
void suchen_V()
{
// Code goes here.
}
void upward()
{
// Code goes here.
}
This looks like Arduino code.
void loop() is your main loop, anything called in there executes over and over.
Not sure how you want the code to run exactly but this sounds like what you are looking to do:
void loop() { scene(); intake(); }
This will execute the scene() subroutine, then it will execute the intake() subroutine which if a Serial byte is available would execute one of your other subroutines. Then it will loop.
Sorry if I have misunderstood what you are trying to do.
Actually it is Arduino code but we try not to say that name in here ... LOL. Do you know "that other" LOL, microcontroller coding well? I had problems that just stumped me and finally just got it to do MOST of what I needed.
Still a couple hurdles yet to go. One is using the "delay()" in "void scene" The program will do nothing while in delay and that can not happen the way I need it to work. Once a serial command is sent, (ex: move eyes right) it must do it right away and not wait for the delay to end. I believe that a "millis" command will do the trick, is that right?
The other problem was with all the delays in the program it took f-o-r-e-v-e-r to loop through scene and no serial intake would take effect till it ran through the entire loop. That was taking too much time so adding "void intake" before each delay solved that for me.
I'm hoping the millis or another timing code will get me away from using delays.
Hey, nobody said robot building was easy!
As of Arduino 0018, delayMicroseconds() no longer disables interrupts.
But you will want to use Serial Event to catch incoming Serial Events.
so rather than a loop checking for Data in the Serial buffer.
if (Serial.available() > 0) {
rather than this use
void serialEvent() { while (Serial.available()) {
incomingByte = Serial.read(); // if it's a capital S (ASCII 83), go to void scene: if (incomingByte == 'S'Winky scene(); } // if it's a capital D (ASCII 68), go to void dizzy: if (incomingByte == 'D'Winky dizzy(); // if it's a capital V (ASCII 86), go to void suchen_V: if (incomingByte == 'V'Winky suchen_V();
// if it's a capital 1 (ASCII 49), go to void upward: if (incomingByte == '1'Winky upward(); // if it's a capital 2 (ASCI ... Bla bla bla
}
the void serialEvent() is called anytime data arrives in the serial buffer