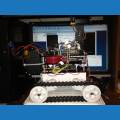
RobertL184
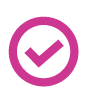
I am trying to send charatcer strings to a serial LCD and have been having difficulties. One of the things I ran into was that space characters were not being sent to it properly. So I started playing around and ran across an interesting bug. I think it is related to some of the problems I have been having. Part of my problem was sending too much data to fast to the display so my first thought was to break a sentence up at the spaces and sleep(50) at every space. This actually worked for me but in making it work I found a interesting bug.
I was looking for Spaces in a string and found that I couldn't find them if I checked with an If statement that read like this if($thischar=" ") but I could find them if I had a variable set to a space character and used an if statement that matched the variable if($thischar=$space).
Below is an example script. The first repeat loop works the second one does not.
$string="My Name is Frizxnizzle!" $how_many=Length($string) $lookfor=" " $found=0 $arrayfound=0 Repeat($strcount,0,$how_many-1,1) $this_char=GetCharAt($string,$strcount) if($this_char=$lookfor) $found=$found+1 endif $this_char=$string[$strcount] if($this_char=$lookfor) $arrayfound=$arrayfound+1 endif EndRepeat Print ("Count = " + $found) Print ("Array Count = " + $arrayfound) $found=0 $arrayfound=0 Repeat($strcount,0,$how_many-1,1) $this_char=GetCharAt($string,$strcount) if($this_char=" ") $found=$found+1 endif $this_char=$string[$strcount] if($this_char=" ") $arrayfound=$arrayfound+1 endif EndRepeat Print ("Count = " + $found) Print ("Array Count = " + $arrayfound)
The Results I get are:
Start Count = 3 Array Count = 3 Count = 0 Array Count = 0 Done
I think the results should be:
Start Count = 3 Array Count = 3 Count = 3 Array Count = 3 Done
I send a lot of data to my LCD without any issues. The voltage is updated every second, the current every 200ms, the time once a second, I've even produced some text based animation on the LCD without issue...
Have you tried using the hex code for a space? i.e. IF($thischar = 0x20)
You would need to use 0x22 if you were looking for double quotes as I found out when playing around with the TellyMate.
However, all of that aside you should be able to send the string "My Name is Frizxnizzle!" to the display without any issue, in fact I am struggling to see a scenario where you would be sending too much too fast to the display - what is it you were trying to send?
Hit another String Parsing problem with sending characters to my LCD Display.
My idea was to read the battery voltage on port ADC1 and have it displayed on the LCD Display.
I finally got it to work with the following code:
$port_value=getadc(ADC1) $voltage=$port_value * $division_factor #Display Battery Volts on On Board Robot Display $roundvolts=Round($voltage,2) $DispVolts="+" + ToString($roundvolts) + " V" SendSerial(D8,9600,$DispVolts)
This code did not:
$port_value=getadc(ADC1) $voltage=$port_value * $division_factor #Display Battery Volts on On Board Robot Display $roundvolts=Round($voltage,2) $DispVolts=ToString($roundvolts) SendSerial(D8,9600,$DispVolts)
I finally figured out that the error message out of the ARC script compiler said it was not seeing $DispVolts as a string in the SendSerial command and instead was seeing it as a number even though the number has a decimal point and is cast to a string just one line ahead of the SendSerial. Putting the "+" sign in front and the "V" at the end finally got the ARC script compiler to see $DispVolts as a text string and not a number.
During Testing with the LCD Display I was also having problems with the SendSerial command parsing the following command:
SendSerial(D8,9600,"0123456")
The ARC compiler did not send the string. Again treating the string of numbers in quotes as a integer number to be sent and not a string.
The following did work though if memory serves me correctly.
SendSerial(D8,9600,"A012345B")
If seems if the first character in a SendSerial string to send is a number the compiler treats it as a number. If it is an alpha character it treats it as a string. I do not recall for sure but it seemed if the first character was a space it would ignore the space and then make its decision on the second character. This may be a legacy feature but it is not exactly following current practices.
@Rich I agree that I should not be having the timing problem with the LCD Display. From what I can tell the processor on the LCD is a little slow for the default 9600 baud rate and when I send it too many characters to fast I overflow the input buffer because the display can't process characters out to the input buffer fast enough. I will try changing the baud rate but for now I have simply fixed the problem by putting a sleep in to any string that is longer than 8 characters. A simple sleep when a space is to be displayed does the trick which is how I got into some of these string parsing oddities.
Guess I'll close this out. now. tired of getting emails.